简介:
在之前的项目中,很多是使用导航栏中视图层,通过KVC来获取网络状态,感觉这个方法有些投机取巧,到了iOS11,iPhoneX的导航栏视图层也进行了修改,除了蛋疼的UI,在获取使用以前获取网络状态的方法时候,果然炸了-_-
解决方案:
可以使用Reachability 的方案来解决该问题,GitHub地址,也可以去CSDN资源页下载。
OC代码:
#import <Foundation/Foundation.h>
#import <SystemConfiguration/SystemConfiguration.h>
//! Project version number for MacOSReachability.
FOUNDATION_EXPORT double ReachabilityVersionNumber;
//! Project version string for MacOSReachability.
FOUNDATION_EXPORT const unsigned char ReachabilityVersionString[];
/**
* Create NS_ENUM macro if it does not exist on the targeted version of iOS or OS X.
*
* @see http://nshipster.com/ns_enum-ns_options/
**/
#ifndef NS_ENUM
#define NS_ENUM(_type, _name) enum _name : _type _name; enum _name : _type
#endif
extern NSString *const kReachabilityChangedNotification;
typedef NS_ENUM(NSInteger, NetworkStatus) {
// Apple NetworkStatus Compatible Names.
NotReachable = 0,
ReachableViaWiFi = 2,
ReachableViaWWAN = 1
};
@class Reachability;
typedef void (^NetworkReachable)(Reachability * reachability);
typedef void (^NetworkUnreachable)(Reachability * reachability);
typedef void (^NetworkReachability)(Reachability * reachability, SCNetworkConnectionFlags flags);
@interface Reachability : NSObject
@property (nonatomic, copy) NetworkReachable reachableBlock;
@property (nonatomic, copy) NetworkUnreachable unreachableBlock;
@property (nonatomic, copy) NetworkReachability reachabilityBlock;
@property (nonatomic, assign) BOOL reachableOnWWAN;
+(instancetype)reachabilityWithHostname:(NSString*)hostname;
// This is identical to the function above, but is here to maintain
//compatibility with Apples original code. (see .m)
+(instancetype)reachabilityWithHostName:(NSString*)hostname;
+(instancetype)reachabilityForInternetConnection;
+(instancetype)reachabilityWithAddress:(void *)hostAddress;
+(instancetype)reachabilityForLocalWiFi;
-(instancetype)initWithReachabilityRef:(SCNetworkReachabilityRef)ref;
-(BOOL)startNotifier;
-(void)stopNotifier;
-(BOOL)isReachable;
-(BOOL)isReachableViaWWAN;
-(BOOL)isReachableViaWiFi;
// WWAN may be available, but not active until a connection has been established.
// WiFi may require a connection for VPN on Demand.
-(BOOL)isConnectionRequired; // Identical DDG variant.
-(BOOL)connectionRequired; // Apple's routine.
// Dynamic, on demand connection?
-(BOOL)isConnectionOnDemand;
// Is user intervention required?
-(BOOL)isInterventionRequired;
-(NetworkStatus)currentReachabilityStatus;
-(SCNetworkReachabilityFlags)reachabilityFlags;
-(NSString*)currentReachabilityString;
-(NSString*)currentReachabilityFlags;
@end
代码概要:
1.Reachability中,包含了三个枚举类型,分别代表无网络,WiFi网络,数据网络
NotReachable = 0,
ReachableViaWiFi = 2,
ReachableViaWWAN = 1
2.也可以使用
-(BOOL)isReachable;
-(BOOL)isReachableViaWWAN;
-(BOOL)isReachableViaWiFi;
三个方法来获取当前是否支持某种网络类型
扫描二维码关注公众号,回复:
1882619 查看本文章
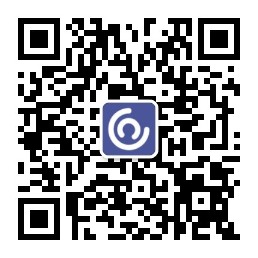
使用方式:
1.将Reachability.h和Reachability.m拷贝到工程中,代码中导入Reachability.h
2.获取网络类型代码:
BOOL isExistenceNetwork = YES;
Reachability *reach = [Reachability reachabilityWithHostName:@"www.apple.com"];
switch ([reach currentReachabilityStatus]) {
case NotReachable:
isExistenceNetwork = NO;
NSLog(@"Current Reachability Status : notReachable");
break;
case ReachableViaWiFi:
isExistenceNetwork = YES;
NSLog(@"Current Reachability Status : WIFI");
break;
case ReachableViaWWAN:
isExistenceNetwork = YES;
NSLog(@"Current Reachability Status : 3/4G");
break;
default:
break;
}
使用网络连接至''www.apple.com'',可以自定义。。此时可以根据当前网络连接状态,获取是使用哪种类型。。