The mafia is finally resting after a year of hard work and not so much money. Mafianca, the little mascot of the gang wanted to go to the beach.
Little did they know that the rival gang had prepared a trap to the little girl and her rubber duck. They know that Mafianca, despite loving the beach, hates water. So they set up some water sprayers to ruin her day. The beach can be seen as a plane. The sprayers are located at different (x, y) points along the shore. Each sprayer is so strong that it creates an infinite line of water, reaching every point with x-coordinate equal to the sprayer.
Mafianca's bodyguards figured this plan out. They didn't have the time to destroy the sprayers, so they decided to do something else. Lying around on the beach was a piece of modern art: a bunch of walls, represented by horizontal line segments, painted by some futuristic anonymous artist. Those walls can be moved, but they can only be moved together and horizontally.
The bodyguards decided that they would try to move this piece of art in order to block the most sprayers they can. A sprayer is blocked if there's at least one wall in both of its sides.
How many sprayers can they block?
Input begins with the number of sprayers, n (1 ≤ n ≤ 1000) and the number of walls in the modern art, m (1 ≤ m ≤ 1000).
Follows n lines, each with two intergers, y and x (0 ≤ y, x ≤ 106), indicating that there's a sprayer at position x, y.
Then follows m lines, each with three integers: y, xbegin and xend (0 ≤ y ≤ 106) and (0 ≤ xbegin < xend ≤ 106) denoting a wall in the modern art has y-coordinate y, begins at xbegin and ends at xend. No wall will have a y-coordinate shared with a sprayer and the walls with same ywill not intersect.
Print the number of sprayers that can be blocked.
1 1
2 2
3 1 3
0
2 5
2 3
2 6
1 0 2
4 0 4
0 2 3
1 4 5
3 5 7
2
4 5
2 3
2 8
2 20
5 1
1 0 2
4 0 4
0 2 3
1 4 5
3 5 7
2
3 4
2 0
2 4
4 7
1 0 2
3 1 3
5 0 2
5 3 4
2
In the test case #4, the beach looks like this:
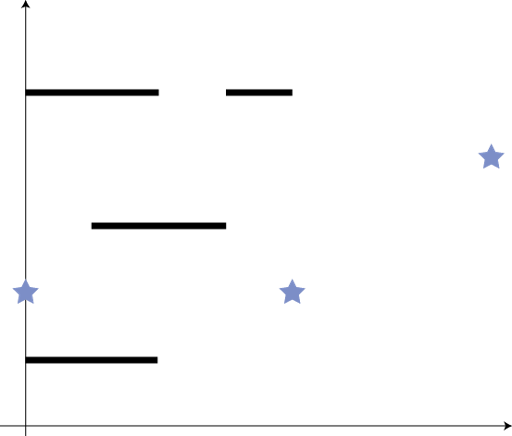
A solution would be
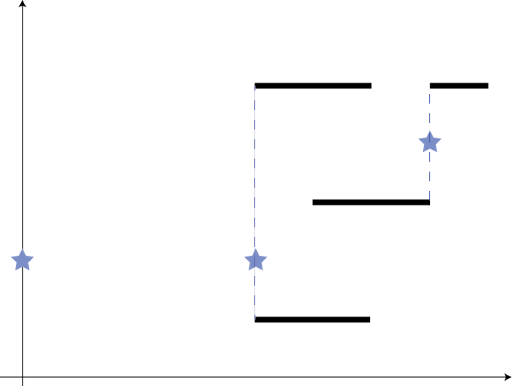

1 #include <iostream> 2 #include <fstream> 3 #include <sstream> 4 #include <cstdlib> 5 #include <cstdio> 6 #include <cmath> 7 #include <string> 8 #include <cstring> 9 #include <algorithm> 10 #include <queue> 11 #include <stack> 12 #include <vector> 13 #include <set> 14 #include <map> 15 #include <list> 16 #include <iomanip> 17 #include <cctype> 18 #include <cassert> 19 #include <bitset> 20 #include <ctime> 21 22 using namespace std; 23 24 #define pau system("pause") 25 #define ll long long 26 #define pii pair<int, int> 27 #define pb push_back 28 #define mp make_pair 29 #define clr(a, x) memset(a, x, sizeof(a)) 30 31 const double pi = acos(-1.0); 32 const int INF = 0x3f3f3f3f; 33 const int MOD = 1e9 + 7; 34 const double EPS = 1e-9; 35 const int D = 1e6; 36 37 /* 38 #include <ext/pb_ds/assoc_container.hpp> 39 #include <ext/pb_ds/tree_policy.hpp> 40 41 using namespace __gnu_pbds; 42 tree<pli, null_type, greater<pli>, rb_tree_tag, tree_order_statistics_node_update> T; 43 */ 44 45 int n, m; 46 struct point { 47 int x, y; 48 point () {} 49 point (int x, int y) : x(x), y(y) {} 50 bool operator < (const point &p) const { 51 return x < p.x; 52 } 53 } P[1015]; 54 int cnt[2000015], con[2015], tcnt[2015]; 55 map<int, int> mmp; 56 set<int> ss; 57 pii p[2015]; 58 int main() { 59 scanf("%d%d", &n, &m); 60 for (int i = 1; i <= n; ++i) { 61 scanf("%d%d", &P[i].y, &P[i].x); 62 } 63 for (int i = 1; i <= m; ++i) { 64 int y, xs, xe; 65 scanf("%d%d%d", &y, &xs, &xe); 66 p[i * 2 - 1] = pii(xs, y); 67 p[i * 2] = pii(xe + 1, y - 2 * D); 68 mmp[xs]; mmp[xe + 1]; 69 } 70 int cnt_index = 0; 71 for (map<int, int>::iterator it = mmp.begin(); it != mmp.end(); ++it) { 72 it -> second = ++cnt_index; 73 con[cnt_index] = it -> first; 74 } 75 sort(p + 1, p + 2 * m + 1); 76 for (int i = 1; i <= n; ++i) { 77 ss.clear(); 78 clr(tcnt, 0); 79 for (int j = 1; j <= 2 * m; ) { 80 int k = j; 81 while (k <= 2 * m && p[k].first == p[j].first) { 82 if (p[k].second < 0) ss.erase(p[k].second + 2 * D); 83 else ss.insert(p[k].second); 84 ++k; 85 } 86 if (ss.lower_bound(P[i].y) != ss.begin() && ss.lower_bound(P[i].y) != ss.end()) { 87 tcnt[mmp[p[j].first]] = 1; 88 } else { 89 tcnt[mmp[p[j].first]] = 0; 90 } 91 j = k; 92 } 93 for (int j = 1; j < cnt_index; ++j) { 94 if (tcnt[j]) { 95 ++cnt[D + con[j] - P[i].x]; 96 --cnt[D + con[j + 1] - P[i].x]; 97 } 98 } 99 } 100 int ans = cnt[0]; 101 for (int i = 1; i <= 2 * D; ++i) { 102 cnt[i] += cnt[i - 1]; 103 ans = max(ans, cnt[i]); 104 } 105 printf("%d\n", ans); 106 return 0; 107 }