50. Pow(x, n)
实现 pow(x, n) ,即计算 x 的 n 次幂函数。
示例 1:
输入: 2.00000, 10 输出: 1024.00000
示例 2:
输入: 2.10000, 3 输出: 9.26100
示例 3:
输入: 2.00000, -2 输出: 0.25000 解释: 2-2 = 1/22 = 1/4 = 0.25
说明:
- -100.0 < x < 100.0
- n 是 32 位有符号整数,其数值范围是 [−231, 231 − 1] 。
代码:
class Solution:
def myPow(self, x, n):
"""
:type x: float
:type n: int
:rtype: float
"""
#### the first method , pycharm能够通过,但是在leetcode网页通过不了
# temp = 1
# if n == 0:
# return temp
# elif n < 0:
# temp = 1 / (x **(abs(n))) ### out od range
# else:
# temp = x **n
# return temp
#### the second method
# if n == 0:
# return 1
# if n < 0:
# return 1.0 / self.myPow(x, -n)
# if n % 2 == 1:
# return x * self.myPow(x * x, n //2)
# else:
# return self.myPow(x * x, n // 2)
#### the third method
return x ** n
69. x 的平方根
实现 int sqrt(int x)
函数。
扫描二维码关注公众号,回复:
1826865 查看本文章
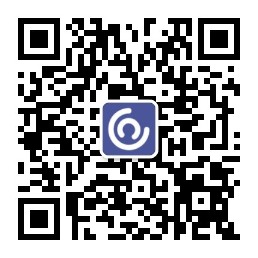
计算并返回 x 的平方根,其中 x 是非负整数。
由于返回类型是整数,结果只保留整数的部分,小数部分将被舍去。
示例 1:
输入: 4 输出: 2
示例 2:
输入: 8 输出: 2 说明: 8 的平方根是 2.82842..., 由于返回类型是整数,小数部分将被舍去。
代码:
class Solution:
def mySqrt(self, x):
"""
:type x: int
:rtype: int
"""
#### the first method
# return int(x ** 0.5)
#### the second method
if x < 0:
raise ValueError("math domain error")
if x <= 1:
return x
left = 0
right = x // 2
while left <= right:
mid = (left + right) >> 1
if mid ** 2 > x:
right = mid - 1
elif mid ** 2 < x:
left = mid + 1
else:
return mid
return left -1