原地址:https://blog.csdn.net/tham_/article/details/45370607
结构体变量、结构指针变量、结构数组作为函数的参数应用实例分析
-
struct stud
-
{
-
long int num;
-
float score;
-
};
/*结构体变量作为函数的参数,修改之后的成员值不能返回到主调函数*/
-
void funvr(struct stud t)
-
{
-
t.num= 2000101;
-
t.score= 71.0;
-
}
/*结构体数组作为函数的参数,修改后的元素的成员值能返回到主调函数*/
-
void funar(struct stud t[])
-
//void funar(struct stud &t)
-
{
-
t[ 0].num= 3000101; /*注意结构体数组元素的成员的引用形式*/
-
t[ 0].score= 81.0;
-
t[ 1].num= 3000102;
-
t[ 1].score= 82.0;
-
}
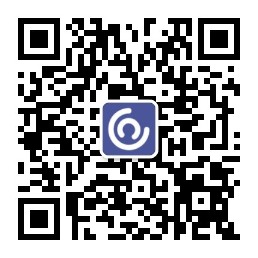
/*结构体指针变量作为函数的参数,修改后的结构体成员的值能返回到主调函数*/
-
void funpr(struct stud *t)
-
{
-
t->num= 4000101; /*注意通过结构体指针变量引用成员的具体形式*/
-
(*t).score= 92.0;
-
}
/*在主函数中分别调用上述函数修改成员值,再验证结果的正确性*/
-
-
-
struct stud
-
{
-
long int num;
-
float score;
-
};
-
-
void funvr(struct stud t)
-
{
-
t.num= 2000101;
-
t.score= 71.0;
-
}
-
-
void funar(struct stud t[])
-
//void funar(struct stud &t)
-
{
-
t[ 0].num= 3000101; /*注意结构体数组元素的成员的引用形式*/
-
t[ 0].score= 81.0;
-
t[ 1].num= 3000102;
-
t[ 1].score= 82.0;
-
}
-
-
void funpr(struct stud *t)
-
{
-
t->num= 4000101; /*注意通过结构体指针变量引用成员的具体形式*/
-
(*t).score= 92.0;
-
}
-
-
void main()
-
{
-
struct stud a[2]={
-
{ 1000101, 61.0}, { 1000102, 62.0}
-
};
-
struct stud b=a[0],*p;
-
-
printf( "old b: b.num:%ld\tb.score:%f\n",b.num,b.score);
-
/*显示结构体变量b的成员的原有值*/
-
funvr(b);
-
/*验证第一种情况,观察并分析结果,看结构体变量作为函数参数时,形参结构体变量成员的值的改变能影响实参结构体变量的成员的值,
-
以下为输出调用函数funvr(b)之后的结果值*/
-
printf( "call funvr() new b: b.num:%ld\tb.score:%f\n ",b.num,b.score);
-
funpr(&b); /*将结构体变量的指针对作为函数的参数*/
-
printf( "call funpr() new b: b.num:%ld\tb.score:%f\n ",b.num,b.score);
-
/*输出结构体数组a元素的原来的成员值*/
-
printf( "old a[0]:a[0].num:%ld\ta[0].score:%f\n ",a[ 0].num,a[ 0].score);
-
printf( "old a[1]:a[1].num:%ld\ta[1].score:%f\n ",a[ 1].num,a[ 1].score);
-
/*将结构体数组a作为函数的参数,然后再输出其元素的成员的值,已经被修改了*/
-
funar(a);
-
printf( " new a[0]:a[0].num:%ld\ta[0].score:%f\n ",a[ 0].num,a[ 0].score);
-
printf( "new a[1]:a[1].num:%ld\ta[1].score:%f\n ",a[ 1].num,a[ 1].score);
-
-
}
【结构体参数调用归纳】
1)结构体变量作为函数参数[实参与形参]时,形参结构体变量成员值的改变不影响对应的实参构体变量成员值的改变。
2)结构体数组或结构体指针变量作为函数参数[实参与形参]时,形参结构体数组元素[或形参结构体指针变量指向的变量]成员值的改变将影响对应的实参构体数组[或实参结构体指针变量指向的变量]成员值的改变。
3)结构体变量可作为函数的参数,函数可返回一结构体类数据
4)p=&b; 使结构体指针变量p指向结构体变量b的空间。
p->num:表示通过指针变量引用结构体变量b的成员num
5)p=a;或p=&a[0];将结构体指针变量指向结构体数组a。则:
①p->num:表示通过指针变量引用结构体数组元素的成员num的值。
②p->num++:表示通过指针变量先引用结构体数组元素的成员num的值,再使该元素的成员num的值加 1,先引用其值然后其加1。
③++p->num:表示使指向的元素的成员num的值加1,再引用其值。
6)p=a;或p=&a[0];表示将结构体指针变量p指向结构体数组a。
①(p++)->num:表示通过指针变量先引用结构体数组元素 的成员num的值,再使指针变量本身加1,指针变量加1表示使指针变量指向结构体数组的下一个元素。
②(++p)->num:先使指针变量本身加1,先使使指针变量指向结构体数组的下一个元素,然后引用指针变量所指向的结构体数组元素的成员num的值。
结构体变量作为函数的形式参数实验总结
-
-
-
-
-
typedef struct str{
-
int len;
-
char s[ 0];
-
-
str( int k)
-
{
-
len=k;
-
}
-
-
}Str;
-
-
void make(Str tmp) // 注意当函数的形参是Str tmp 时,传递的方式是普通的结构体变量传值调用,这里让len的值改变不会影响下面的temp的len
-
{
-
tmp.len= 2;
-
}
-
-
void make_ptr(Str &tmp) // 当函数的形参是Str &tmp,时传递的是结构体的地址,当这里使得tmp.len值改变时,实参temp.len值也会改变
-
{
-
tmp.len= 3;
-
}
-
-
void make_ptr2(Str *tmp) // 函数参数是一个Str类型的指针,传地址调用就不用说了,(上面的引用方式是C++的特性之一,C语言不能那样使用)
-
{
-
tmp->len= 4;
-
}
-
-
-
int main(int argc, char** argv) {
-
-
struct str temp(1);
-
printf( "temp=%d\n",temp);
-
-
make(temp);
-
printf( "temp=%d\n",temp);
-
-
make_ptr(temp);
-
printf( "temp=%d\n",temp);
-
-
return 0;
-
}
结构体变量作为函数参数传递的3种方法
将一个结构体变量中的数据传递给另一个函数,有下列3种方法:
用结构体变量名作参数。一般较少用这种方法。
用指向结构体变量的指针作实参,将结构体变量的地址传给形参。
用结构体变量的引用变量作函数参数。
下面通过一个简单的例子来说明,并对它们进行比较。
【例7.5】有一个结构体变量stu,内含学生学号、姓名和3门课的成绩。要求在main函数中为各成员赋值,在另一函数print中将它们的值输出。
-
1) 用结构体变量作函数参数。
-
-
-
-
using namespace std;
-
struct Student//声明结构体类型Student
-
{
-
int num;
-
char name[ 20];
-
float score[ 3];
-
};
-
int main( )
-
{
-
void print(Student); //函数声明,形参类型为结构体Student
-
Student stu; //定义结构体变量
-
stu.num= 12345; //以下5行对结构体变量各成员赋值
-
stu.name= "Li Fung";
-
stu.score[ 0]= 67.5;
-
stu.score[ 1]= 89;
-
stu.score[ 2]= 78.5;
-
print(stu); //调用print函数,输出stu各成员的值
-
return 0;
-
}
-
void print(Student st)
-
{
-
cout<<st.num<< " "<<st.name<< " "<<st.score[ 0]
-
<< " " <<st.score[ 1]<< " "<<st.score[ 2]<< endl;
-
}
-
运行结果为:
-
12345 Li Fung 67.5 89 78.5 ( 2)
-
2)用指向结构体变量的指针作实参在上面程序的基础上稍作修改即可。
-
复制纯文本新窗口
-
-
-
-
using namespace std;
-
struct Student
-
{
-
int num; string name; //用string类型定义字符串变量
-
float score[ 3];
-
}stu={ 12345, "Li Fung", 67.5, 89, 78.5}; //定义结构体student变量stu并赋初值
-
int main( )
-
{
-
void print(Student *); //函数声明,形参为指向Student类型数据的指针变量
-
Student *pt=&stu; //定义基类型为Student的指针变量pt,并指向stu
-
print(pt); //实参为指向Student类数据的指针变量
-
return 0;
-
}
-
//定义函数,形参p是基类型为Student的指针变量
-
void print(Student *p)
-
{
-
cout<<p->num<< " "<<p->name<< " "<<p->score[ 0]<< " " <<
-
p->score[ 1]<< " "<<p->score[ 2]<< endl;
-
}
在print函数中输出p所指向的结构体变量的各个成员值,它们也就是stu的成员值。在main函数中也可以不定义指针变量pt,而在调用print函数时以&stu作为实参,把stu的起始地址传给实参p。
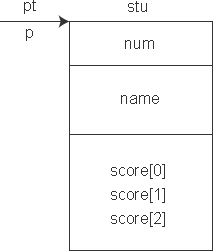
图7.10
-
3) 用结构体变量的引用作函数参数
-
-
-
-
using namespace std;
-
struct Student
-
{
-
int num;
-
string name;
-
float score[ 3];
-
}stu={ 12345, "Li Li", 67.5, 89, 78.5};
-
int main( )
-
{
-
void print(Student &);
-
//函数声明,形参为Student类型变量的引用
-
print(stu);
-
//实参为结构体Student变量
-
return 0;
-
}
-
//函数定义,形参为结构体Student变量的引用
-
void print(Student &stud)
-
{
-
cout<<stud.num<< " "<<stud.name<< " "<<stud.score[ 0]
-
<< " " <<stud.score[ 1]<< " "<<stud.score[ 2]<< endl;
-
}
程序(1)用结构体变量作实参和形参,程序直观易懂,效率是不高的。
程序(2)采用指针变量作为实参和形参,空间和时间的开销都很小,效率较高。但程序(2)不如程序(1)那样直接。
程序(3)的实参是结构体Student类型变量,而形参用Student类型的引用,虚实结合时传递的是stu的地址,因而效率较高。它兼有(1)和(2)的优点。
引用变量主要用作函数参数,它可以提高效率,而且保持程序良好的可读性(引用'&'是C++的新特性)。在本例中用了string方法定义字符串变量,在某些C++系统中目前不能运行这些程序,读者可以修改程序,使之能在自己所用的系统中运行。