题目
计算数组的小和
java代码
package com.lizhouwei.chapter8;
/**
* @Description: 计算数组的小和
* @Author: lizhouwei
* @CreateDate: 2018/5/8 20:29
* @Modify by:
* @ModifyDate:
*/
public class Chapter8_13 {
public int smallSum(int[] arr) {
int[] res = new int[arr.length];
return mergeSort(arr, 0, arr.length - 1, res);
}
public int mergeSort(int[] arr, int left, int right, int[] res) {
if (left >= right) {
return 0;
}
int mid = (left + right) / 2;
int len1 = mergeSort(arr, left, mid, res);
int len2 = mergeSort(arr, mid + 1, right, res);
int len3 = 0;
int leftIndex = left;
int rightIndex = mid + 1;
int k = left;
while (leftIndex <= mid && rightIndex <= right) {
if (arr[leftIndex] < arr[rightIndex]) {
len3 = len3 + arr[leftIndex] * (right - rightIndex + 1);
res[k++] = arr[leftIndex++];
} else {
res[k++] = arr[rightIndex++];
}
}
while (leftIndex <= mid) {
res[k++] = arr[leftIndex++];
}
while (rightIndex <= right) {
res[k++] = arr[rightIndex++];
}
leftIndex = left;
while (leftIndex < right) {
arr[leftIndex] = res[leftIndex++];
}
return len1 + len2 + len3;
}
//测试
public static void main(String[] args) {
Chapter8_13 chapter = new Chapter8_13();
int[] arr = {1, 3, 5, 2, 4, 6};
System.out.print("数组 arr = {1, 3, 5, 2, 4, 6}中最小和为:");
int maxLen = chapter.smallSum(arr);
System.out.print(maxLen);
}
}
结果
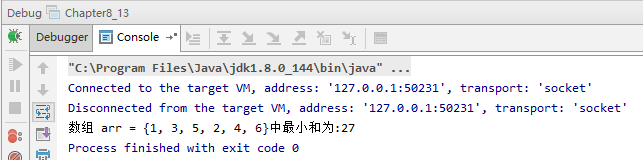