DOM:Document Object Model 文档对象类型
模态框案例
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>模态框案例</title> <style type="text/css"> *{margin:0;padding: 0;} html,body{height: 100%;} /* 4. 设置所创建的div的样式*/ /*rgba最后一个参数表示透明度,0~1之间取值*/ #box{height: 100%;width: 100%;background: rgba(0,0,0,0.3);} /*8. 设置p标签的样式*/ #content{ position: relative; top: 150px; width: 400px; height: 200px; line-height: 200px; text-align: center; color: black; background-color: red; margin:auto; /*margin:auto 表示上下左右自动居中*/ } /*10. 设置span标签的样式*/ #span1{ position: absolute; top: 0; right: 0; width: 20px; width: 20px; line-height: 20px; color: black; background-color: white; text-align: center; } </style> </head> <body> <!-- id是button的属性;为其添加一个方法(事件)onclick,不要写在行内,写在js标签里 --> <button id="btn" >弹出</button> </body> <script type="text/javascript"> // dom 树状结构;每个标签被视为一个节点,节点称为DOM元素 console.log(document) // 1. getElementById() :通过标签ID获取DOM元素 var btn = document.getElementById("btn") console.log(btn) // 2. 创建一个DOM元素 div var oDiv = document.createElement("div") // 为创建的这个div DOM元素设置ID oDiv.id = "box"; // 5. 创建 p DOM元素 var oP = document.createElement("p") oP.id = "content"; // 6. 为p标签添加内容 oP.innerHTML = "模态框成功弹出" // 7. 把p标签添加到div中 oDiv.appendChild(oP) // 添加子节点 // 9. 创建span DOM 元素, 并p标签中添加 span,并为span标签添加内容 X var oSpan = document.createElement("span") oSpan.id = "span1"; oSpan.innerHTML = "X"; oP.appendChild(oSpan) // onclick对应一种方法 btn.onclick = function(){ // alert(111) // 3. 动态的在 body 中 在 btn 前面添加一个div console.log(this) // btn.parentNode.insertBefore(oDiv,btn) // 所以上面的代码可以用this 代替,如下 this.parentNode.insertBefore(oDiv,btn) } // 11. 点击X 关闭弹出的页面 oSpan.onclick = function(){ // removeChild() : 移除子节点; body这个父节点调用的removeChild()这个方法,所以oDiv.parentNode oDiv.parentNode.removeChild(oDiv) // oDiv.parentNode 不能用 this.parentNode代替,因为 this.parentNode表示oSpan的父节点 oP } </script> </html> <!-- 总结: getElementById() // 获取节点DOM元素 createElement() // 创建节点DOM元素 oDiv.id = "box" // 设置节点的属性 oP.innerHTML = "模态框成功弹出" // 设置节点中的内容(字符串格式) appendChild() // 往父节点中添加子节点 onclick // 点击事件 parentNode // 获取父节点 insertBefore(所插入的新节点,新节点后面的节点) // 插入新节点 removeChild() // 移除子节点 -->
点击有惊喜 案例
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>点击有惊喜</title> <style type="text/css"> *{margin: 0;padding: 0;} .box{ width: 200px; height: 200px; background: red; text-align: center; color: white; line-height: 200px; margin:30px auto; } </style> </head> <body> <div class="box"> 点击有惊喜 ! </div> </body> <script type="text/javascript"> // 通过 类名 获取DOM元素 var oBox = document.getElementsByClassName("box")[0] console.log(oBox) var a = 0; oBox.onclick = function(){ a++; // 通过 a%4 取余的方式实现循环 if (a%4===1){ this.style.background = "green"; // this指的是 oBox // innerText表示只取到标签内的文本;innerHTML是把标签内的所有元素都取到 this.innerText = "继续点"; }else if (a%4===2){ this.style.background = "blue"; this.innerText = "哈哈,骗你的" }else if (a%4===3){ this.style.background = "transparent"; // 表示 透明的 this.innerText = "" }else{ this.style.background = "red"; this.innerText = "点击有惊喜 !" } } </script> </html> <!-- style中有很多属性,background只是其中之一 -->
简易留言板:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>简易留言板</title> <style type="text/css"> </style> </head> <body> <h1>简易留言板</h1> <div id="box"></div> <textarea id="msg"></textarea> <input type="button" id="btn" value="留言"> <button onclick="sum()">统计</button> </body> <script type="text/javascript"> // 创建ul标签 var ul = document.createElement("ul"); // 获取box标签 var box = document.getElementById("box"); box.appendChild(ul); var btn = document.getElementById("btn"); // 获取 textarea 中值的方法: var msg = document.getElementById("msg"); // msg.value // 通过 msg.value能获取到msg中的值 // 定义一个全局变量,用于统计计数;button点击一次加1,span标签点击一次减1 var count = 0; // btn有一个onclick事件 btn.onclick = function(){ // btn 的onclick事件是 获取到 textarea中的值并追加到 ul中 console.log(msg.value); // 在点击事件的时候创建 li 标签 var li = document.createElement("li"); // 设置li的内容 li.innerHTML = msg.value + " " + "<span>X</span>"; // 利用 ul.appendChild(li) 多次添加li时,新添加的li会添加到原先的li后面 // 通过 标签名 获取 上面创建的 DOM元素 var lis = document.getElementsByTagName("li"); // 类似数组(HTMLCollection[])的形式 console.log(lis) // 如果lis 中内容为空,则直接在ul中添加li;如果ul中已经有li,则新的li添加到原先的li前面 if (lis.length == 0) { ul.appendChild(li); count++; // button点击则加1 }else{ ul.insertBefore(li,lis[0]); // lis[0] 表示第一个 li标签 count++; // button点击则加1 } msg.value = ""; // 清空 textarea 中的内容 // 遍历 span 标签,为每个 span 标签添加 onclick 事件:点击span标签即移除相应的li span = document.getElementsByTagName("span") for (var i=0; i<span.length;i++){ span[i].onclick = function(){ ul.removeChild(this.parentNode); // this 表示相应的 span 标签;this.parentNode即相应的 li count--; // span点击一次则减1 } } } // 用于统计条数 function sum(){ alert("总共有"+count+"条留言"); } </script> </html>
选项卡
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>选项卡</title> <style type="text/css"> *{padding: 0;margin: 0;} #tab{ width: 480px; margin: 20px auto; border:1px solid red; } ul{list-style: none;} ul li{ float: left; width: 160px; height: 60px; line-height: 60px; text-align: center; background-color: #C6C3C6; } ul li a{ text-decoration: none; color: black; } li.active{ background-color: #FDFDFD; } p{ display: none; height: 200px; line-height: 200px; text-align: center; background-color: #FDFDFD; } p.active{ display: block; } </style> </head> <body> <div id="tab"> <ul> <li class="active"><a href="#">首页</a></li> <li><a href="#">新闻</a></li> <li><a href="#">图片</a></li> </ul> <p class="active">首页内容</p> <p>新闻内容</p> <p>图片内容</p> </div> </body> <script type="text/javascript"> var tabli = document.getElementsByTagName("li"); var tabContent = document.getElementsByTagName("p"); // 遍历 tabli 添加 onclick 点击事件 for (var i=0;i<tabli.length;i++){ // 遍历时的i保存为相应的 index tabli[i].index = i; // 需要注意的地方 // 添加点击事件 tabli[i].onclick = function(){ // 给相应的li添加 class = "active" 之前,先遍历把所有的li的 class 清空、p 的class清空; for (var j=0;j<tabli.length;j++){ tabli[j].className = ""; tabContent[j].className = ""; } // 点击之后让相应li的 class="active" this.className = "active"; // 通过 classname 获取类的值 // 并让相应p的 class="active" console.log(this.index); // this 表示 tabli[i] tabContent[this.index].className = "active"; // 需要注意的地方:此外不能直接写成 tabContent[i].className = "active" 因为遍历完之后i为最后一个数 } } </script> </html>
仿淘宝搜索框(该功能用 palceholder 也能实现)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>仿淘宝搜索框</title> <style type="text/css"> *{margin: 0;padding: 0} #search{position: relative;} input{ outline: none; /* 去除输入框的默认颜色 */ display: block; width: 300px; height: 30px; margin-top: 20px; font-size: 20px; border:2px solid red; border-radius: 10px; } label { position: absolute; top: 6px; left: 10px; font-size: 15px; color: grey; } </style> </head> <body> <div id="search"> <input type="text" id="text1"> <label for="txt" id="msg">路飞学城</label> </div> </body> <script type="text/javascript"> var txt = document.getElementById("text1"); var msg = document.getElementById("msg"); // oninput :检测用户表单输入 txt.oninput = function(){ if (this.value == ""){ // this.value表示输入框中的内容 msg.style.display = "block"; }else{ msg.style.display = "none"; } } </script> </html>
获取当前最新时间
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>获取当前最新时间</title> <style type="text/css"> </style> </head> <body> </body> <script type="text/javascript"> // 定时器 setInterval(function(){ var date = new Date(); var y = date.getFullYear(); var m = date.getMonth() + 1; var d = date.getDate(); // getDate()得到的结果是: 0~11 var h = date.getHours(); var min = date.getMinutes(); var s = date.getSeconds(); document.body.innerHTML = "今天是" + y + "年" + m + "月" + d + "日" + " " + sum(h) + ":" + sum(min) + ":" + sum(s); // 让时分秒调用sum函数 function sum(x){ // 加0函数:如果时分秒小于10,则在其前面加0 if(x<10){ return "0" + x; } return x; } },1000) </script> </html>
匀速运动案例
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>匀速运动案例</title> <style type="text/css"> *{margin: 0;padding: 0;} .box{ width: 100px; height: 100px; background: pink; position: relative; top: 150px; left: 0; } </style> </head> <body> <div class="wrap"> <button id="btn">运动</button> <div class="box" id="box1"></div> </div> </body> <script type="text/javascript"> var btn = document.getElementById("btn"); var box = document.getElementById("box1") var sum = 0; var timer = null; // 用于储存定时器返回的 id btn.onclick = function(){ timer = setInterval(function(){ sum += 2; if (sum >= 1226){ clearInterval(timer); box.style.display = "none"; // sum = 0; // 如果 sum >= 1226,则返回左侧重新进行 }else{ box.style.left = sum + "px"; // 要记得加单位 px } },10); } </script> </html>
5秒之后关闭广告
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>5秒之后关闭广告</title> <style type="text/css"> *{margin: 0;padding: 0;} img{position: fixed;} #left{left: 0;} #right{right: 0;} ul{list-style: none;} </style> </head> <body> <img src="./1.jpg" id="left"> <img src="./2.jpg" id="right"> <ul> <li>屠龙宝刀,点击就送</li> <li>倚天长剑,点击就送</li> <li>屠龙宝刀,点击就送</li> <li>倚天长剑,点击就送</li> <li>屠龙宝刀,点击就送</li> <li>倚天长剑,点击就送</li> <li>屠龙宝刀,点击就送</li> <li>倚天长剑,点击就送</li> <li>屠龙宝刀,点击就送</li> <li>倚天长剑,点击就送</li> <li>屠龙宝刀,点击就送</li> </ul> </body> <script type="text/javascript"> window.onload = function(){ // window.onload 表示窗口加载的时候 执行后面的函数 var pic1 = document.getElementById("left"); var pic2 = document.getElementById("right"); // 设置定时器 setTimeout(function(){ // 执行一次,所以用 setTimeout pic1.style.display = "none"; pic2.style.display = "none"; },5000) } </script> </html>
小米滚动
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>小米滚动</title> <style type="text/css"> *{margin: 0;padding: 0;} .wrap{ width: 468px; height: 400px; border:3px solid #14191D; position: relative; overflow: hidden; margin: 100px auto; } #xiaomi{ position: absolute; top: 0; /* 通过top值的变化 来实现图片的上下移动 */ left: 0; } span{ display: block; width: 100%; height: 200px; position: absolute; } #picUp{top: 0;} #picDown{top: 200px;} </style> </head> <body> <div id="box" class="wrap"> <img src="./小米6X.jpg" id="xiaomi"> <span class="up" id="picUp"></span> <span class="down" id="picDown"></span> </div> </body> <script type="text/javascript"> var upSpan = document.getElementById("picUp"); var downSpan = document.getElementById("picDown"); var img = document.getElementById("xiaomi"); var count = 0; var timer = null; upSpan.onmouseover = function(){ // 表示鼠标移到 xxx 上面的时候 // 先清定时器 clearInterval(timer) // 移到上面的时候把下面的定时器清掉 timer = setInterval(function(){ count -= 1; count >= -828 ? img.style.top = count + "px" : clearInterval(timer); // condition ? result1 : result2; 即相当于 if.. else.. 语句; 828 = 1228(图片高度)- 400(盒子高度) },10) }; downSpan.onmouseover = function(){ clearInterval(timer) // 移到下面的时候把上面的定时器清掉 timer = setInterval(function(){ count += 1; count <= 0 ? img.style.top = count + "px" : clearInterval(timer); },10) }; </script> </html>
无缝轮播图
扫描二维码关注公众号,回复:
179306 查看本文章
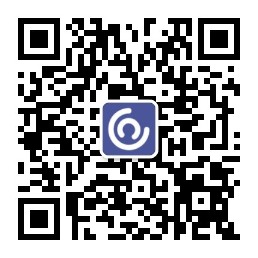
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>无缝轮播图</title> <style type="text/css"> *{margin: 0;padding: 0;} ul{list-style: none;} .box{ width: 600px; height: 200px; margin: 50px auto; overflow: hidden; position: relative; } ul li{float: left;} .box ul{ width: 500%; /* 设置宽度,一张图片是100% */ position: absolute; top: 0; right: 0; /* 通过right值的变化 来移动图片 */ } img{ width: 300px; height: 200px; } </style> </head> <body> <div class="box"> <ul> <li><a href="#"><img src="./1.jpg"></a></li> <li><a href="#"><img src="./2.jpg"></a></li> <li><a href="#"><img src="./5X.jpg"></a></li> <li><a href="#"><img src="./go.jpg"></a></li> <li><a href="#"><img src="./python2.png"></a></li> </ul> </div> </body> <script type="text/javascript"> var box = document.getElementsByClassName("box")[0]; var ul = box.children[0]; // 通过childrec能够获取子节点 var timer = null; var count = 0; timer = setInterval(autoPlay,10) // autoPlay后面不要加 () // 函数的声明 function autoPlay(){ count--; count <= -900 ? count=0 : count; ul.style.left = count + "px"; } // 鼠标移上去时,清除定时器 box.onmouseover = function(){ clearInterval(timer) } // 鼠标离开时,开启定时器 box.onmouseout = function(){ timer = setInterval(autoPlay,10); // 此处也需要返回timer } </script> </html>
BOM
BOM输出:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>BOM为了输出</title> </head> <body> <button onclick="printLuffy()">打印</button> <button onclick="findLuffy()">查找</button> </body> <script type="text/javascript"> // 1. BOM: Browser Object Model 浏览器对象模型 // 核心:浏览器 // 输出、屏幕的宽高、滚动的宽高、 setInterval setTimeout window.open() close() location等等 // 输出;常用输出有以下4个(这些都是window的功能,window可省略不写) // 1.弹出框:alert(1) // 2. console // 主要用于浏览器的调试 // 3. prompt(message,defaultValue) var pro = prompt("路飞学城") console.log(pro) // 4. confirm(): 相较于alert(),confirm()多了“取消”功能;如果点出“确定”,返回true,如果点出“取消”,返回false var m = confirm("学习BOM"); console.log(m) // 输出扩展: // 5. print() : 调用打印机 function printLuffy(){ print(); // 打印功能; } // 6. find() :查找 function findLuffy(){ var m2 = confirm("学习BOM"); find(m2); } </script> </html>
open/close方法
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>open和close方法</title> </head> <body> <!-- 行内JS中的open()方法,window不能省略 --> <button onclick="window.open('https://www.luffycity.com/')">路飞学城</button> <button>打开百度</button> <button onclick="window.close()">关闭</button> <button>关闭</button> </body> <script type="text/javascript"> var oBtn = document.getElementsByTagName("button")[1]; oBtn.onclick = function(){ // open("https://www.baidu.com/") open("about:blank","_self") // 在当前页面(_self)打开一个空白页面(about:blank) } var closeBtn = document.getElementsByTagName("button")[3]; closeBtn.onclick = function(){ if (confirm("是否关闭?")){ close(); } } </script> </html>
BOM其它的对象和方法
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>其它的BOM对象</title> </head> <body> </body> <script type="text/javascript"> // 返回浏览器的用户设备信息 console.log(window.navigator.userAgent) // PC端还是移动端 console.log(window.location) // 取到浏览器本地的信息 // 经常使用的方法 window.location.href = "https://www.baidu.com"; // 跳转 // 全局刷新 (尽量少用) window.location.reload(); // 这个方法会重新把所有的代码走一遍 </script> </html>
client系列
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>client系列</title> <style type="text/css"> .box{ width: 200px; height: 200px; position: relative; border:1px solid red; margin: 10px 0px 0px 0px; padding: 10px; } </style> </head> <body> <div class="box"> </div> </body> <script type="text/javascript"> // client系列 // clientTop :内容区域到边框顶部的距离 // clientLeft : 内容区域到边框左部的距离 // clientWidth : 内容区域的 + 左右padding值,即可视宽度 // clientHeight : 内容区域的 + 上下padding值,即可视高度 var oBox = document.getElementsByClassName("box")[0]; console.log(oBox.clientTop) console.log(oBox.clientLeft) console.log(oBox.clientWidth) console.log(oBox.clientHeight) </script> </html>
屏幕的可视区域
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>屏幕的可视区域</title> </head> <body> </body> <script type="text/javascript"> // 通常需要写 window.onload = ... window.onload = function(){ window.onresize = function(){ // window.onresize能够监听浏览器可视区域的大小;当浏览器大小发生变化时,自动触发该方法 console.log(document.documentElement.clientWidth); // 浏览器屏幕的可视宽度 console.log(document.documentElement.clientHeight); // 浏览器屏幕的可视高度 } } </script> </html>
注:单个区域用client,整个屏幕用 document.documentElement
offset系列
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>offset系列</title> </head> <body> <div style="position: relative;"> <div id="box" style="width: 200px;height: 200px;border:1px solid red;padding: 10px;margin: 10px;position: absolute;top:20px;left: 30px;"> </div> </div> </body> <script type="text/javascript"> window.onload = function(){ var box = document.getElementById("box"); // 占位宽 高 Top Left console.log(box.offsetTop); console.log(box.offsetLeft); console.log(box.offsetWidth); console.log(box.offsetHeight); // offsetTop :如果盒子没有设置定位:到浏览器顶部的距离;如果盒子设置定位:以盒子为基准的top值 // offsetLeft :如果盒子没有设置定位:到浏览器左部的距离;如果盒子设置定位:以盒子为基准的left值 // offsetWidth :内容+左右padding + 左右border // offsetHeight : 内容+上下padding + 上下border } </script> </html>
scroll系列
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>scroll系列</title> <style type="text/css"> *{margin: 0;padding: 0;} </style> </head> <body style="width: 2000px;height: 2000px;"> <div style="height: 200px;background-color: red;"></div> <div style="height: 200px;background-color: green;"></div> <div style="height: 200px;background-color: blue;"></div> <div style="height: 200px;background-color: black;"></div> <div style="height: 200px;background-color: yellow;"></div> <div id="box" style="width: 200px;height: 200px;border:1px solid red;overflow: auto;"> <p> 哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈 哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈 哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈 哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈 哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈 哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈 哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈 哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈 哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈哈 </p> </div> </body> <script type="text/javascript"> window.onload = function(){ // 实时监听滚动事件 // window.onscroll 是获取整个屏幕 // window.onscroll = function(){ // onscrll:监听滚动事件 // console.log(111) // console.log("上"+document.documentElement.scrollTop); // 距离浏览器顶部的距离 // console.log("左"+document.documentElement.scrollLeft); // 距离浏览器左侧的距离 // console.log("宽"+document.documentElement.scrollWidth); // 屏幕的宽度 // console.log("高"+document.documentElement.scrollHeight); // 屏幕的高度 // } var box = document.getElementById("box"); box.onscroll = function(){ // id为box的区域调用 onscroll() console.log("上"+box.scrollTop); // 距离盒子顶部的距离 console.log("左"+box.scrollLeft); // 距离盒子左侧的距离 console.log("宽"+box.scrollWidth); // 内容的宽度(包含padding,但不包含 边框和margin),即 内容的宽度+padding console.log("高"+box.scrollHeight); // 内容的高度(包含padding,但不包含 边框和margin),即 内容的高度+padding } } </script> </html>