小猪佩奇知识准备:
turtle模块:
时间控制:turtle.speed(0)
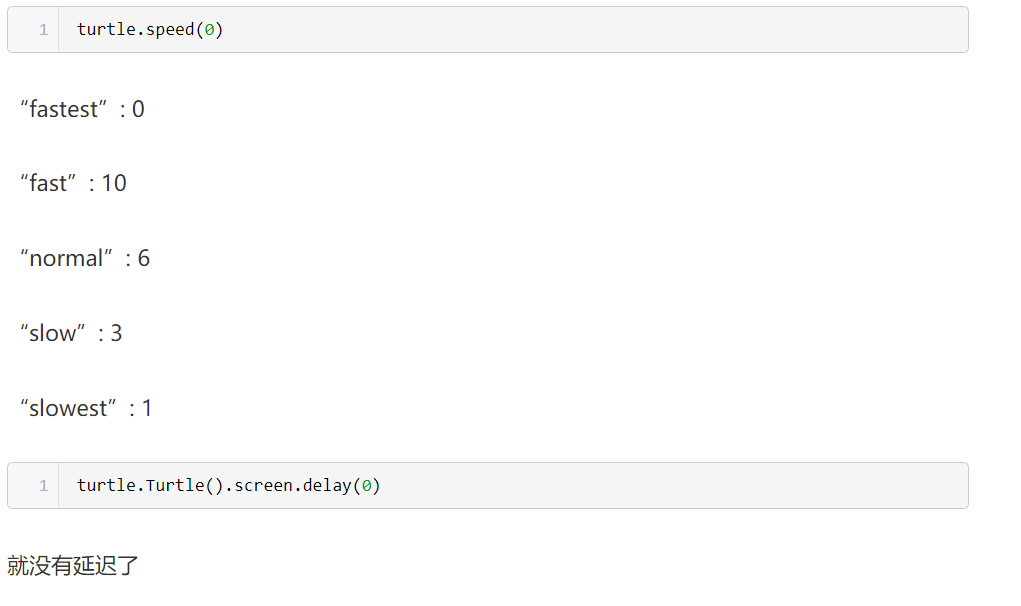

1 import turtle as t 2 3 4 def running(): 5 6 t.pensize(4) 7 t.hideturtle() 8 t.colormode(255) 9 t.color((255, 155, 192), "pink") 10 t.setup(840, 500) 11 t.speed(10) 12 13 # 鼻子 14 t.pu() 15 t.goto(-100, 100) 16 t.pd() 17 t.seth(-30) 18 t.begin_fill() 19 a = 0.4 20 for i in range(120): 21 if 0 <= i < 30 or 60 <= i < 90: 22 a = a + 0.08 23 t.lt(3) # 向左转3度 24 t.fd(a) # 向前走a的步长 25 else: 26 a = a - 0.08 27 t.lt(3) 28 t.fd(a) 29 t.end_fill() 30 31 t.pu() 32 t.seth(90) 33 t.fd(25) 34 t.seth(0) 35 t.fd(10) 36 t.pd() 37 t.pencolor(255, 155, 192) 38 t.seth(10) 39 t.begin_fill() 40 t.circle(5) 41 t.color(160, 82, 45) 42 t.end_fill() 43 44 t.pu() 45 t.seth(0) 46 t.fd(20) 47 t.pd() 48 t.pencolor(255, 155, 192) 49 t.seth(10) 50 t.begin_fill() 51 t.circle(5) 52 t.color(160, 82, 45) 53 t.end_fill() 54 55 # 头 56 t.color((255, 155, 192), "pink") 57 t.pu() 58 t.seth(90) 59 t.fd(41) 60 t.seth(0) 61 t.fd(0) 62 t.pd() 63 t.begin_fill() 64 t.seth(180) 65 t.circle(300, -30) 66 t.circle(100, -60) 67 t.circle(80, -100) 68 t.circle(150, -20) 69 t.circle(60, -95) 70 t.seth(161) 71 t.circle(-300, 15) 72 t.pu() 73 t.goto(-100, 100) 74 t.pd() 75 t.seth(-30) 76 a = 0.4 77 for i in range(60): 78 if 0 <= i < 30 or 60 <= i < 90: 79 a = a + 0.08 80 t.lt(3) # 向左转3度 81 t.fd(a) # 向前走a的步长 82 else: 83 a = a - 0.08 84 t.lt(3) 85 t.fd(a) 86 t.end_fill() 87 88 # 耳朵 89 t.color((255, 155, 192), "pink") 90 t.pu() 91 t.seth(90) 92 t.fd(-7) 93 t.seth(0) 94 t.fd(70) 95 t.pd() 96 t.begin_fill() 97 t.seth(100) 98 t.circle(-50, 50) 99 t.circle(-10, 120) 100 t.circle(-50, 54) 101 t.end_fill() 102 103 t.pu() 104 t.seth(90) 105 t.fd(-12) 106 t.seth(0) 107 t.fd(30) 108 t.pd() 109 t.begin_fill() 110 t.seth(100) 111 t.circle(-50, 50) 112 t.circle(-10, 120) 113 t.circle(-50, 56) 114 t.end_fill() 115 116 # 眼睛 117 t.color((255, 155, 192), "white") 118 t.pu() 119 t.seth(90) 120 t.fd(-20) 121 t.seth(0) 122 t.fd(-95) 123 t.pd() 124 t.begin_fill() 125 t.circle(15) 126 t.end_fill() 127 128 t.color("black") 129 t.pu() 130 t.seth(90) 131 t.fd(12) 132 t.seth(0) 133 t.fd(-3) 134 t.pd() 135 t.begin_fill() 136 t.circle(3) 137 t.end_fill() 138 139 t.color((255, 155, 192), "white") 140 t.pu() 141 t.seth(90) 142 t.fd(-25) 143 t.seth(0) 144 t.fd(40) 145 t.pd() 146 t.begin_fill() 147 t.circle(15) 148 t.end_fill() 149 150 t.color("black") 151 t.pu() 152 t.seth(90) 153 t.fd(12) 154 t.seth(0) 155 t.fd(-3) 156 t.pd() 157 t.begin_fill() 158 t.circle(3) 159 t.end_fill() 160 161 # 腮 162 t.color((255, 155, 192)) 163 t.pu() 164 t.seth(90) 165 t.fd(-95) 166 t.seth(0) 167 t.fd(65) 168 t.pd() 169 t.begin_fill() 170 t.circle(30) 171 t.end_fill() 172 173 # 嘴 174 t.color(239, 69, 19) 175 t.pu() 176 t.seth(90) 177 t.fd(15) 178 t.seth(0) 179 t.fd(-100) 180 t.pd() 181 t.seth(-80) 182 t.circle(30, 40) 183 t.circle(40, 80) 184 185 # 身体 186 t.color("red", (255, 99, 71)) 187 t.pu() 188 t.seth(90) 189 t.fd(-20) 190 t.seth(0) 191 t.fd(-78) 192 t.pd() 193 t.begin_fill() 194 t.seth(-130) 195 t.circle(100, 10) 196 t.circle(300, 30) 197 t.seth(0) 198 t.fd(230) 199 t.seth(90) 200 t.circle(300, 30) 201 t.circle(100, 3) 202 t.color((255, 155, 192), (255, 100, 100)) 203 t.seth(-135) 204 t.circle(-80, 63) 205 t.circle(-150, 24) 206 t.end_fill() 207 208 # 手 209 t.color((255, 125, 192)) 210 t.pu() 211 t.seth(90) 212 t.fd(-40) 213 t.seth(0) 214 t.fd(-27) 215 t.pd() 216 t.seth(-160) 217 t.circle(300, 15) 218 t.pu() 219 t.seth(90) 220 t.fd(15) 221 t.seth(0) 222 t.fd(0) 223 t.pd() 224 t.seth(-10) 225 t.circle(-20, 90) 226 227 t.pu() 228 t.seth(90) 229 t.fd(30) 230 t.seth(0) 231 t.fd(237) 232 t.pd() 233 t.seth(-20) 234 t.circle(-300, 15) 235 t.pu() 236 t.seth(90) 237 t.fd(20) 238 t.seth(0) 239 t.fd(0) 240 t.pd() 241 t.seth(-170) 242 t.circle(20, 90) 243 244 # 脚 245 t.pensize(10) 246 t.color((112, 128, 128)) 247 t.pu() 248 t.seth(90) 249 t.fd(-75) 250 t.seth(0) 251 t.fd(-180) 252 t.pd() 253 t.seth(-90) 254 t.fd(40) 255 t.seth(-180) 256 t.color("black") 257 t.pensize(15) 258 t.fd(20) 259 260 t.pensize(10) 261 t.color((240, 128, 128)) 262 t.pu() 263 t.seth(90) 264 t.fd(40) 265 t.seth(0) 266 t.fd(90) 267 t.pd() 268 t.seth(-90) 269 t.fd(40) 270 t.seth(-180) 271 t.color("black") 272 t.pensize(15) 273 t.fd(20) 274 275 # 尾巴 276 t.pensize(4) 277 t.color((255, 155, 192)) 278 t.pu() 279 t.seth(90) 280 t.fd(70) 281 t.seth(0) 282 t.fd(95) 283 t.pd() 284 t.seth(0) 285 t.circle(70, 20) 286 t.circle(10, 330) 287 t.circle(70, 30) 288 t.done() 289 290 running()