655. Add Strings
以字符串的形式给出两个非负整数 num1 和 num2,返回 num1 和 num2 的和。
样例
给定 num1 = “123”,num2 = “45”
返回 “168”
public class Solution {
/**
* @param num1: a non-negative integers
* @param num2: a non-negative integers
* @return: return sum of num1 and num2
*/
public String addStrings(String num1, String num2) {
// write your code here
int num,carry=0;
int len1=num1.length()-1;
int len2=num2.length()-1;
String result="";
while (len1>=0&&len2>=0){
num=(carry+num1.charAt(len1)-'0'+num2.charAt(len2)-'0')%10;
carry=(carry+num1.charAt(len1--)-'0'+num2.charAt(len2--)-'0')/10;
result= num+result;
}
if(len1>len2){
while (len1>=0){
num=(carry+num1.charAt(len1)-'0')%10;
result=num+result;
carry=(carry+num1.charAt(len1--)-'0')/10;
}
}else while (len2>=0){
num=(carry+num2.charAt(len2)-'0')%10;
result=num+result;
carry=(carry+num2.charAt(len2--)-'0')/10;
}
if(carry==1)
return carry+result;
else return result;
}
}
671. 循环单词
The words are same rotate words if rotate the word to the right by
loop, and get another. Count how many different rotate word sets in
dictionary.E.g. picture and turepic are same rotate words.
样例
Given dict = [“picture”, “turepic”, “icturep”, “word”, “ordw”, “lint”]
return 3.
public class Solution {
/*
* @param words: A list of words
* @return: Return how many different rotate words
*/
public int countRotateWords(List<String> words) {
// Write your code here
Set<String> dict=new HashSet<>();
for(String w:words){
String s=w+w;
for(int i=0;i<w.length();i++){
dict.remove(s.substring(i,i+w.length()));
}
dict.add(w);
}
return dict.size();
}
}
684. 缺少的字符串
给出两个字符串,你需要找到缺少的字符串
样例
给一个字符串 str1 = This is an example, 给出另一个字符串 str2 = is example
返回 [“This”, “an”]
public class Solution {
/**
* @param str1: a given string
* @param str2: another given string
* @return: An array of missing string
*/
public List<String> missingString(String str1, String str2) {
// Write your code here
if(str1.length()<str2.length()){
String temp=str1;
str1=str2;
str2=temp;
}
String STR1[]=str1.split(" ");
String STR2[]=str2.split(" ");
List<String>s2=new ArrayList<>();
for(String s:STR2)
s2.add(s);
List<String>result=new ArrayList<>();
for(String s:STR1)
if(!s2.contains(s))
result.add(s);
return result;
}
}
697. Sum of Square Numbers
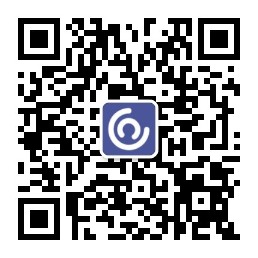
给一个整数 c, 你需要判断是否存在两个整数 a 和 b 使得 a^2 + b^2 = c.
样例
给出 n = 5
返回 true // 1 * 1 + 2 * 2 = 5
给出 n = -5
返回 false
public class Solution {
/**
* @param num: the given number
* @return: whether whether there're two integers
*/
public boolean checkSumOfSquareNumbers(int num) {
// write your code here
for(int i=(int)Math.sqrt(num);i>=0;i--){
if(i*i==num) return true;
int j=num-i*i; int k=(int)Math.sqrt(j);
if(k*k==j) return true;
}
return false;
}
}
702. 连接两个字符串中的不同字符
给出两个字符串, 你需要修改第一个字符串,将所有与第二个字符串中相同的字符删除, 并且第二个字符串中不同的字符与第一个字符串的不同字符连接
样例
给出 s1 = aacdb, s2 = gafd
返回 cbgf
给出 s1 = abcs, s2 = cxzca;
返回 bsxz
public class Solution {
/**
* @param s1: the 1st string
* @param s2: the 2nd string
* @return: uncommon characters of given strings
*/
public String concatenetedString(String s1, String s2) {
// write your code here
String result="";
for(char s:s1.toCharArray()){
if(s2.indexOf(s+"")==-1)
result+=s;
}
for(char s:s2.toCharArray())
if(s1.indexOf(s+"")==-1)
result+=s;
return result;
}
}
小结
天色已晚,改日再刷