前言
本篇讲述了顺序表的相关知识,以及动态顺序表的代码实现。
文章目录
1.线性表
顺序表和链表一般情况下都会叫他们线性表。
线性表(linear list)是n个具有相同特性的数据元素的有限序列。线性表是一种在实际中广泛使 用的数据结构,常见的线性表:顺序表、链表、栈、队列、字符串…
线性表在逻辑上是线性结构,也就说是连续的一条直线。但是在物理结构上并不一定是连续的, 线性表在物理上存储时,通常以数组和链式结构的形式存储。
2.顺序表
顺序表简单说就是一个数组。
顺序表是用一段物理地址连续的存储单元依次存储数据元素的线性结构,一般情况下采用数组存
储。在数组上完成数据的增删查改。
顺序表一般可以分为:
- 静态顺序表:使用定长数组存储元素。
- 动态顺序表:使用动态开辟的数组存储。
2.1 静态顺序表
2.2 动态顺序表
扫描二维码关注公众号,回复:
17365329 查看本文章
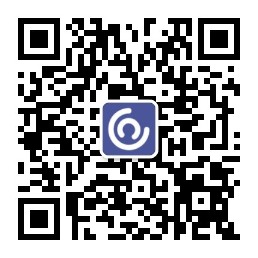
3动态顺序表代码详解
3.1 顺序表功能(头文件)
这里的顺序表和我们之前的通讯录有些相像,我们可以结合之前的通讯录来学习。
链接: 八功能通讯录
顺序表的头文件(功能)如下:seqList.h
#pragma once
#include<stdio.h>
#include<stdlib.h>
#include<assert.h>
typedef int SLDataType;
// sequence list
typedef struct SeqList
{
SLDataType* a;
int size; // 有效数据
int capacity; // 空间容量
}SL;
void SLInit(SL* psl);//初始化顺序表
void SLDestroy(SL* psl);//摧毁顺序表
void SLPrint(SL* psl);//打印顺序表
void SLCheckCapacity(SL* psl);//检测顺序表的容量
// 头尾插入删除
void SLPushBack(SL* psl, SLDataType x);//尾插
void SLPushFront(SL* psl, SLDataType x);//头插
void SLPopBack(SL* psl);//尾删
void SLPopFront(SL* psl);//头删
// 任意下标位置的插入删除
void SLInsert(SL* psl, int pos, SLDataType x);//任意下标插
void SLErase(SL* psl, int pos);//任意下标删
// 找到返回下标
// 没有找到返回-1
int SLFind(SL* psl, SLDataType x);
3.2 各功能函数
3.2.1 初始化顺序表
void SLInit(SL* psl)
{
assert(psl);
psl->a = NULL;
psl->size = 0;
psl->capacity = 0;
}
3.2.2 摧毁顺序表
void SLDestroy(SL* psl)
{
assert(psl);
if (psl->a != NULL)
{
free(psl->a);
psl->a = NULL;
psl->size = 0;
psl->capacity = 0;
}
}
3.2.3 打印顺序表
void SLPrint(SL* psl)
{
assert(psl);
for (int i = 0; i < psl->size; i++)
{
printf("%d ", psl->a[i]);
}
printf("\n");
}
3.2.4 检测顺序表的容量
void SLCheckCapacity(SL* psl)
{
assert(psl);
if (psl->size == psl->capacity)
{
int newCapacity = psl->capacity == 0 ? 4 : psl->capacity * 2;
SLDataType* tmp = (SLDataType*)realloc(psl->a, sizeof(SLDataType) * newCapacity);
if (tmp == NULL)
{
perror("realloc fail");
return;
}
psl->a = tmp;
psl->capacity = newCapacity;
}
}
3.2.5 在顺序表尾部插入元素
void SLPushBack(SL* psl, SLDataType x)
{
assert(psl);
SLCheckCapacity(psl);
psl->a[psl->size] = x;
psl->size++;
}
3.2.6 在顺序表头部插入元素
void SLPushFront(SL* psl, SLDataType x)
{
assert(psl);
SLCheckCapacity(psl);
// 挪动数据
int end = psl->size - 1;
while (end >= 0)
{
psl->a[end + 1] = psl->a[end];
--end;
}
psl->a[0] = x;
psl->size++;
}
3.2.7 在顺序表尾部删除元素
void SLPopBack(SL* psl)
{
assert(psl);
assert(psl->size > 0);
psl->size--;
}
3.2.8 在顺序表头部删除元素
void SLPopFront(SL* psl)
{
assert(psl);
assert(psl->size > 0);
int begin = 1;
while (begin < psl->size)
{
psl->a[begin - 1] = psl->a[begin];
++begin;
}
psl->size--;
}
3.2.9 在顺序表任意处插入元素
void SLInsert(SL* psl, int pos, SLDataType x)
{
assert(psl);
assert(pos >= 0 && pos <= psl->size);
SLCheckCapacity(psl);
// 挪动数据
int end = psl->size - 1;
while (end >= pos)
{
psl->a[end + 1] = psl->a[end];
--end;
}
psl->a[pos] = x;
psl->size++;
}
3.2.10 在顺序表任意处删除元素
void SLErase(SL* psl, int pos)
{
assert(psl);
assert(pos >= 0 && pos < psl->size);
// 挪动覆盖
int begin = pos + 1;
while (begin < psl->size)
{
psl->a[begin - 1] = psl->a[begin];
++begin;
}
psl->size--;
}
3.2.11 查找元素
int SLFind(SL* psl, SLDataType x)
{
assert(psl);
for (int i = 0; i < psl->size; i++)
{
if (psl->a[i] == x)
{
return i;
}
}
return -1;
}
3.2.12 主函数
主函数包括目录及各个功能的测试。完整代码见3.2.13 gitee
3.2.13 完整代码
链接: 动态顺序表完整代码:gitee
4.顺序表的缺陷
1.尾部插入效率尚可,头部或者中间插入删除,需要挪动数据,效率低下。
2.容量满了之后只能扩容。扩容是有一定的消耗,且存在一定的空间浪费。(假设空间为100,扩容到200,但只需要120个数据,会有大量空间浪费)