1.Linux时间戳
#include <time.h> #include <sys/time.h> std::string get_time() { time_t tt = time(NULL);//这句返回的只是一个时间cuo tm* t= localtime(&tt); char ti[128]; sprintf(ti,"%d-%02d-%02d_%02d-%02d-%02d",t->tm_year + 1900, t->tm_mon + 1, t->tm_mday, t->tm_hour, t->tm_min, t->tm_sec); std::string ttt = ti; printf("time: %s\n",ti); return ttt; }
2.字符串分割(非常实用)头文件不列,win,linux均测试
void SplitString(const string& s, vector<string>& v, const string& c) { string::size_type pos1, pos2; pos2 = s.find(c); pos1 = 0; while (string::npos != pos2) { v.push_back(s.substr(pos1, pos2 - pos1)); pos1 = pos2 + c.size(); pos2 = s.find(c, pos1); } if (pos1 != s.length()) v.push_back(s.substr(pos1)); }
3.string2*,win linux均测试
int str2int(const string str) { int temp; stringstream stream(str); stream>>temp; return temp; }
如果要2其他(float double等),直接将int换成其他
4.opencv Rect按中心点外扩Size,头文件不贴
Rect rectCenterScale(Rect rect, Size size) { rect = rect + size; Point pt; pt.x = cvRound(size.width/2.0); pt.y = cvRound(size.height/2.0); return (rect-pt); }
5.创建文件夹
#include <stdio.h> #include <mutex> #include <arpa/inet.h> #include <string.h> #include <unistd.h> #include <arpa/inet.h> #include <sys/stat.h> int creat_dir(std::string dir) { if (access(dir.c_str(), 0) == -1) { std::cout << dir << " is not existing" << std::endl; cout << "now make it" << endl; #ifdef WIN32 int flag=mkdir(dir.c_str()); #endif #ifdef LINUX int flag=mkdir(dir.c_str(), 0777); #endif if (flag == 0) { std::cout << "make successfully" << std::endl; } else { std::cout << "make errorly" << std::endl; } } return 0; }
这个头文件还真忘了,应该就是其中几个(Linux)。win加预处理器定义或者修改mkdir为_mkdir,头文件为#include <direct.h>。
6.base642Mat(base64码转opencv Mat)
static const std::string base64_chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" "abcdefghijklmnopqrstuvwxyz" "0123456789+/"; std::string base64_decode(std::string const &encoded_string) { int in_len = encoded_string.size(); int i = 0; int j = 0; int in_ = 0; unsigned char char_array_4[4], char_array_3[3]; std::string ret; while (in_len-- && (encoded_string[in_] != '=')) { char_array_4[i++] = encoded_string[in_]; in_++; if (i == 4) { for (i = 0; i < 4; i++) char_array_4[i] = base64_chars.find(char_array_4[i]); char_array_3[0] = (char_array_4[0] << 2) + ((char_array_4[1] & 0x30) >> 4); char_array_3[1] = ((char_array_4[1] & 0xf) << 4) + ((char_array_4[2] & 0x3c) >> 2); char_array_3[2] = ((char_array_4[2] & 0x3) << 6) + char_array_4[3]; for (i = 0; (i < 3); i++) ret += char_array_3[i]; i = 0; } } if (i) { for (j = i; j < 4; j++) char_array_4[j] = 0; for (j = 0; j < 4; j++) char_array_4[j] = base64_chars.find(char_array_4[j]); char_array_3[0] = (char_array_4[0] << 2) + ((char_array_4[1] & 0x30) >> 4); char_array_3[1] = ((char_array_4[1] & 0xf) << 4) + ((char_array_4[2] & 0x3c) >> 2); char_array_3[2] = ((char_array_4[2] & 0x3) << 6) + char_array_4[3]; for (j = 0; (j < i - 1); j++) ret += char_array_3[j]; } return ret; } cv::Mat base64ToMat(string &base64) { string decoded_string = base64_decode(base64); std::vector<uchar> data(decoded_string.begin(), decoded_string.end()); cv::Mat image = cv::imdecode(data, CV_LOAD_IMAGE_UNCHANGED); return image; }
头文件就不贴了。win linux亲测
7.Mat2Base64(opencv Mat 转base64码)
static const std::string base64_chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" "abcdefghijklmnopqrstuvwxyz" "0123456789+/"; std::string base64_encode(const char* bytes_to_encode, int in_len) { std::string ret; int i = 0; int j = 0; unsigned char char_array_3[3]; unsigned char char_array_4[4]; while (in_len--) { char_array_3[i++] = *(bytes_to_encode++); if (i == 3) { char_array_4[0] = (char_array_3[0] & 0xfc) >> 2; char_array_4[1] = ((char_array_3[0] & 0x03) << 4) + ((char_array_3[1] & 0xf0) >> 4); char_array_4[2] = ((char_array_3[1] & 0x0f) << 2) + ((char_array_3[2] & 0xc0) >> 6); char_array_4[3] = char_array_3[2] & 0x3f; for (i = 0; (i <4); i++) ret += base64_chars[char_array_4[i]]; i = 0; } } if (i) { for (j = i; j < 3; j++) char_array_3[j] = '\0'; char_array_4[0] = (char_array_3[0] & 0xfc) >> 2; char_array_4[1] = ((char_array_3[0] & 0x03) << 4) + ((char_array_3[1] & 0xf0) >> 4); char_array_4[2] = ((char_array_3[1] & 0x0f) << 2) + ((char_array_3[2] & 0xc0) >> 6); char_array_4[3] = char_array_3[2] & 0x3f; for (j = 0; (j < i + 1); j++) ret += base64_chars[char_array_4[j]]; while ((i++ < 3)) ret += '='; } return ret; } std::string matToBase64(cv::Mat img) { std::vector<uchar> data_encode; cv::imencode(".jpg", img, data_encode); std::string str_encode(data_encode.begin(), data_encode.end()); string base64String = base64_encode(str_encode.c_str(), str_encode.length()); return base64String; }
8.json读和取,需要jsoncpp.cpp和json.h。网上一搜一大把
扫描二维码关注公众号,回复:
1732591 查看本文章
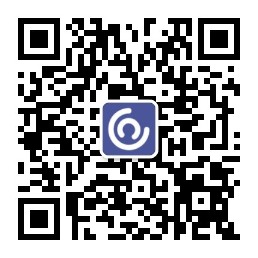
写
std::string getJsonString(parameter *P) { Json::FastWriter writer; Json::Value root; root["flage"] = P->flage; //root["ID_base64"] = P->ID_base64; //root["img_base64"] = P->img_base64; root["up_img_base64"] = P->up_img; root["db"] = P->db; //root["Compare_image"] = P->Compare_image; //root["Feature"] = P->Feature; //root["Score"] = P->Score; std::string jsonString = writer.write(root); return jsonString; }读
Json::Reader reader; Json::Value root; string ID_base64; string base_image_base64; if (reader.parse(postResponseStr, root)) { ID_base64 = root["ID_Base64"].asString(); base_image_base64 = root["Compare_image"].asString(); }----------------------------------
未完待续
----------------------------------