第四次作业-宝宝相册
题目
用Listview建立宝宝相册,相册内容及图片可自行设定,也可在资料文件中获取。给出模拟器仿真界面及代码截图。 (参考例4-8)
创建工程项目
创建名为baby
的项目工程,最后的工程目录结构如下图所示:
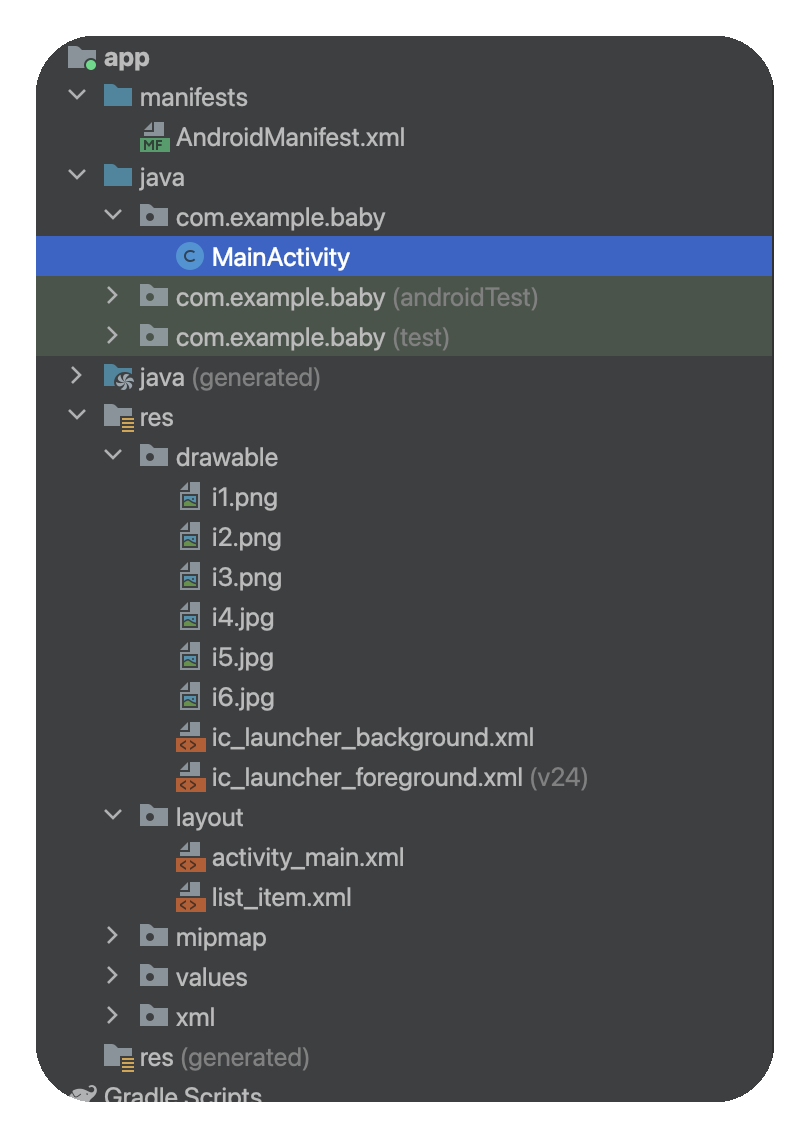
res/drawable文件中的i1、i2、i3、i4、i5、i6均为图片,即宝宝相册图片,网上自行选取照片即可。
res/layout为文件布局文件,activity_main.xml
为自动生成的自定义布局文件,list_item.xml
为自定义布局文件
布局文件
-
创建自定义布局文件
list_item.xml
文件<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="horizontal"> <ImageView android:id="@+id/news_thumb" android:layout_width="100dp" android:layout_height="100dp" android:layout_margin="5dp"/> <LinearLayout android:orientation="vertical" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="5dp"> <TextView android:id="@+id/news_title" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="16sp" /> <TextView android:id="@+id/news_info" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="14sp" android:layout_marginTop="5dp"/> </LinearLayout> </LinearLayout>
-
修改MainActivity.xml布局文件
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="16dp" android:paddingRight="16dp"> <ListView android:id="@+id/list" android:layout_width="match_parent" android:layout_height="match_parent"/> </RelativeLayout>
MainActivity文件
package com.example.baby;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Random;
public class MainActivity extends AppCompatActivity {
private ListView listView;
private SimpleAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 假设有一个包含数据的List
List<Map<String, String>> data = new ArrayList<>();
Map<String, String> item1 = new HashMap<>();
item1.put("news_thumb", String.valueOf(R.drawable.i1)); //R.drawable.i1引用照片资源文件
item1.put("news_title", "毡帽系列");
item1.put("news_info", "此系列服装有点cute,像不像小车夫。");
data.add(item1);
Map<String, String> item2 = new HashMap<>();
item2.put("news_thumb", String.valueOf(R.drawable.i2)); //R.drawable.i2引用照片资源文件
item2.put("news_title", "蜗牛系列");
item2.put("news_info", "宝宝变成了小蜗牛,爬啊爬啊爬啊。");
data.add(item2);
Map<String, String> item3 = new HashMap<>();
item3.put("news_thumb", String.valueOf(R.drawable.i3));
item3.put("news_title", "小蜜蜂系列");
item3.put("news_info", "小蜜蜂,嗡嗡嗡,飞到西,飞到东。");
data.add(item3);
Map<String, String> item4 = new HashMap<>();
item4.put("news_thumb", String.valueOf(R.drawable.i4));
item4.put("news_title", "毡帽系列");
item4.put("news_info", "此系列服装有点cute,像不像小车夫。");
data.add(item4);
Map<String, String> item5 = new HashMap<>();
item5.put("news_thumb", String.valueOf(R.drawable.i5));
item5.put("news_title", "蜗牛系列");
item5.put("news_info", "宝宝变成了小蜗牛,爬啊爬啊爬啊。");
data.add(item5);
Map<String, String> item6 = new HashMap<>();
item6.put("news_thumb", String.valueOf(R.drawable.i6));
item6.put("news_title", "小蜜蜂系列");
item6.put("news_info", "小蜜蜂,嗡嗡嗡,飞到西,飞到东。");
data.add(item6);
// 定义数据的键与布局文件中组件的映射
String[] from = {
"news_thumb", "news_title", "news_info"};
int[] to = {
R.id.news_thumb, R.id.news_title, R.id.news_info};
// 创建SimpleAdapter
adapter = new SimpleAdapter(this, data, R.layout.list_item, from, to);
// 关联SimpleAdapter与ListView
listView = findViewById(R.id.list);
listView.setAdapter(adapter);
// 为ListView添加一个项目点击监听器,当点击项目时显示对话框
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
// 获取点击项目的数据
Map<String, String> itemData = (Map<String, String>) parent.getItemAtPosition(position);
// 从点击项目的数据中提取文本信息以供对话框使用
String title = itemData.get("news_title");
String info = itemData.get("news_info");
// 创建并显示一个自定义对话框
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setTitle(title)
.setMessage(info)
.setPositiveButton("确定", null); // 没有操作的确定按钮
AlertDialog dialog = builder.create();
dialog.show();
}
});
}
}
修改AndroidManifest.xml文件
<activity
android:name=".MainActivity"
android:exported="true"
android:label="SimpleAdapterDemo"> <!--修改导航栏名称-->
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>