在Android应用中,你可以通过服务(Service)来实现蓝牙数据读取。以下是一个简单的示例,演示如何创建一个Android服务以连接到蓝牙设备并读取数据。在实际应用中,你需要确保你的应用具备蓝牙权限,并使用合适的蓝牙库进行连接和数据读取。
目录
1.创建一个新的Android服务
首先,你需要创建一个继承自Service
的类,这个服务将用于处理蓝牙连接和数据读取。你可以创建一个名为BluetoothService
的类:
import android.app.Service;
import android.content.Intent;
import android.os.Binder;
import android.os.IBinder;
public class BluetoothService extends Service {
// 在这里编写服务的具体逻辑
}
2.实现蓝牙连接和数据读取逻辑
在BluetoothService
类中,你可以实现蓝牙连接和数据读取的逻辑。这通常需要使用Android的蓝牙API或第三方蓝牙库(如Android Bluetooth SDK或其他开源库)。
下面是一个简化的示例,假设你使用Android的蓝牙API(需要处理权限、配对等细节):
import android.app.Service;
import android.content.Intent;
import android.os.Binder;
import android.os.IBinder;
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.bluetooth.BluetoothSocket;
import java.io.IOException;
import java.io.InputStream;
import java.util.UUID;
public class BluetoothService extends Service {
private final IBinder mBinder = new LocalBinder();
private BluetoothAdapter mBluetoothAdapter;
private BluetoothSocket mBluetoothSocket;
private InputStream mInputStream;
private boolean mReadingData = false;
// 定义一个UUID,用于标识蓝牙服务
private static final UUID MY_UUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
public class LocalBinder extends Binder {
BluetoothService getService() {
return BluetoothService.this;
}
}
@Override
public IBinder onBind(Intent intent) {
return mBinder;
}
// 初始化蓝牙连接
public boolean initializeBluetooth() {
mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (mBluetoothAdapter == null) {
return false; // 设备不支持蓝牙
}
BluetoothDevice device = mBluetoothAdapter.getRemoteDevice(蓝牙设备的地址);
try {
mBluetoothSocket = device.createRfcommSocketToServiceRecord(MY_UUID);
mBluetoothSocket.connect();
mInputStream = mBluetoothSocket.getInputStream();
return true;
} catch (IOException e) {
return false; // 连接失败
}
}
// 开始读取数据
public void startReadingData() {
mReadingData = true;
new Thread(new Runnable() {
@Override
public void run() {
byte[] buffer = new byte[1024];
int bytes;
while (mReadingData) {
try {
bytes = mInputStream.read(buffer);
// 处理从蓝牙设备读取的数据
// 发送数据给Activity或者做其他处理
} catch (IOException e) {
break;
}
}
}
}).start();
}
// 停止读取数据
public void stopReadingData() {
mReadingData = false;
}
// 断开蓝牙连接
public void disconnectBluetooth() {
if (mBluetoothSocket != null) {
try {
mReadingData = false;
mInputStream.close();
mBluetoothSocket.close();
} catch (IOException e) {
// 处理异常
}
}
}
}
3.在你的Activity中使用服务
在你的应用的活动(Activity)中,你可以绑定到这个服务,并调用服务的方法来初始化蓝牙连接、启动/停止数据读取和断开蓝牙连接。
以下是一个活动的示例,展示如何使用服务:
扫描二维码关注公众号,回复:
17255279 查看本文章
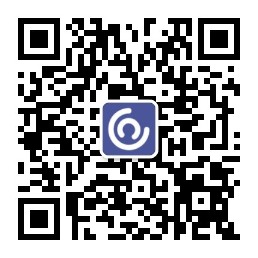
import android.app.Activity;
import android.content.ComponentName;
import android.content.ServiceConnection;
import android.os.Bundle;
import android.os.IBinder;
import android.content.Intent;
public class BluetoothActivity extends Activity {
private BluetoothService mBluetoothService;
private boolean mServiceBound = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_bluetooth);
// 启动服务
Intent intent = new Intent(this, BluetoothService.class);
startService(intent);
// 绑定到服务
bindService(intent, mServiceConnection, BIND_AUTO_CREATE);
}
// 创建服务连接对象
private ServiceConnection mServiceConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
BluetoothService.LocalBinder binder = (BluetoothService.LocalBinder) service;
mBluetoothService = binder.getService();
mServiceBound = true;
// 初始化蓝牙连接
boolean success = mBluetoothService.initializeBluetooth();
if (success) {
// 启动数据读取
mBluetoothService.startReadingData();
}
}
@Override
public void onServiceDisconnected(ComponentName name) {
mServiceBound = false;
}
}
@Override
protected void onDestroy() {
super.onDestroy();
// 停止数据读取和断开蓝牙连接
if (mServiceBound) {
mBluetoothService.stopReadingData();
mBluetoothService.disconnectBluetooth();
}
// 解绑服务
if (mServiceConnection != null) {
unbindService(mServiceConnection);
}
}
}
上述内容提供了一个基本框架,以在Android中创建一个服务来连接蓝牙设备并读取数据。请注意,蓝牙通信通常涉及更多的细节,例如蓝牙配对、错误处理、数据解析等。你需要根据实际需求进行更多的自定义和异常处理。另外,你还需要在AndroidManifest.xml文件中添加相关的权限和服务声明。