本教程中,我们将学习如何在 Spring Boot 中整合使用 Log4j2 日志框架。
Log4j2 介绍
Spring Boot 中默认使用 Logback 作为日志框架,接下来我们将学习如何在 Spring Boot 中集成与配置 Log4j2。在配置之前,我们需要知道的是 Log4j2 是 Log4j 的升级版,它在 Log4j 的基础上做了诸多改进:
- 1.异步日志;
- 2.支持 Java8 lambda 风格的懒加载日志;
- 3.过滤器;
- 4.插件;
- 5.并发性改进;
- 6.支持: SLF4J, Commons Logging, Log4j-1.x 以及 java.util.logging;
- 7.配置热加载;
- 8.自定义日志级别;
看到上面这些新特性,我们肯定特别想在我们的 Spring Boot 应用中使用 Log4j2
添加 Maven 依赖
Spring Boot 默认使用的是 logback, 想要使用 Log4j2, 我们需要首先排除掉默认的日志框架,然后添加 log4j2 依赖,下面是 pom.xml
文件:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-logging</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-log4j2</artifactId>
</dependency>
添加 Gradle 依赖(可选)
如果你是通过 Gradle 来构建项目的,看下面:
dependencies {
compile 'org.springframework.boot:spring-boot-starter-log4j2'
}
configurations {
all {
exclude group: 'org.springframework.boot', module: 'spring-boot-starter-logging'
}
}
添加 Log4j2 配置文件
Spring Boot 支持以下 4 种格式的配置文件:
- 1.xml(默认的)
- 2.json
- 3.yaml
- 4.properties 文件
Spring Boot 如果在 classpath:
目录下找到了 log4j2.xml
或者 log4j2.json
或者 log4j2.properties
或者log4j2.yaml
的其中任意一个配置文件,就会自动加载并使用它。
接下来,我们来看看 log4j2.xml
格式,要如何配置?
在 /src/main/resource
目录下创建 log4j2.xml
配置文件:
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="WARN" monitorInterval="30">
<Properties>
<Property name="PID">????</Property>
<Property name="LOG_PATTERN">%clr{%d{yyyy-MM-dd HH:mm:ss.SSS}}{faint} %clr{%5p} %clr{${sys:PID}}{magenta} %clr{---}{faint} %clr{[%15.15t]}{faint} %clr{%-40.40c{1.}}{cyan} %clr{:}{faint} %m%n%xwEx</Property>
</Properties>
<Appenders>
<Console name="Console" target="SYSTEM_OUT" follow="true">
<PatternLayout pattern="${LOG_PATTERN}"/>
</Console>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
通过 Properties 文件来创建配置文件(可选)
如果你不喜欢通过 xml
的方式,你还可以选择在 /src/main/resources
目录下创建 log4j2.properties
:
扫描二维码关注公众号,回复:
17244934 查看本文章
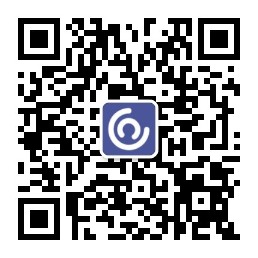
status = error
name = Log4j2Sample
appenders = console
appender.console.type = Console
appender.console.name = STDOUT
appender.console.layout.type = PatternLayout
appender.console.layout.pattern = %d{yyyy-MM-dd HH:mm:ss} - %msg%n
rootLogger.level = warn
rootLogger.appenderRefs = stdout
rootLogger.appenderRef.stdout.ref = STDOUT
Log4j2 实战
一切准备就绪后,我们在 Application 启动类中,创建一个测试的接口,在接口中打印日志:
package site.exception.springbootlog4j2;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class SpringBootLog4j2Application {
public static void main(String[] args) {
SpringApplication.run(SpringBootLog4j2Application.class, args);
}
private static final Logger LOG = LogManager.getLogger(SpringBootLog4j2Application.class);
@GetMapping("/test")
public String test() {
LOG.debug("debug 级别日志 ...");
LOG.info("info 级别日志 ...");
LOG.warn("warn 级别日志 ...");
LOG.error("error 级别日志 ...");
LOG.fatal("fatal 级别日志 ...");
return "Hello Log4j2 !";
}
}
启动项目,看看控制台输出:
2023-10-06 22:15:59.815 INFO 11936 --- [nio-8080-exec-1] s.e.s.SpringBootLog4j2Application : info 级别日志 ...
2023-10-06 22:15:59.815 WARN 11936 --- [nio-8080-exec-1] s.e.s.SpringBootLog4j2Application : warn 级别日志 ...
2023-10-06 22:15:59.815 ERROR 11936 --- [nio-8080-exec-1] s.e.s.SpringBootLog4j2Application : error 级别日志 ...
2023-10-06 22:15:59.815 FATAL 11936 --- [nio-8080-exec-1] s.e.s.SpringBootLog4j2Application : fatal 级别日志 ...
最后
欢迎大家关注我【小白技术圈】,回复 B01或b01,双手奉上Java相关书籍和面试题!