目录标题
Chapter 1: Introduction
Why Choose Rust as a Medium for Learning English
Rust is a modern programming language that focuses on performance, safety, and concurrency (并发性). It has gained significant traction (关注度) in the tech industry due to its robust (健壮的) features. Learning Rust can not only enhance your programming skills but also provide an excellent opportunity to improve your English. The language’s documentation and community support are primarily in English, offering a natural setting to practice the language.
Similarities and Differences Between Rust and C++
If you’re already proficient in C++, transitioning to Rust can be relatively smooth. Both languages share some common paradigms (范式), such as strong typing (强类型) and low-level memory control (底层内存控制). However, there are also key differences that make Rust unique:
Memory Safety (内存安全性)
Rust aims to eliminate common bugs related to memory management, which are often a concern in C++.
Ownership (所有权)
One of the most distinctive features of Rust is its ownership model, which ensures that each value in the program has a single “owner” responsible for its management.
Immutability (不可变性)
By default, variables in Rust are immutable, meaning they cannot be altered once declared. This is in contrast to C++, where mutability (可变性) is the default behavior.
Community and Ecosystem (社区和生态系统)
Rust has a vibrant (充满活力的) community and a growing ecosystem of libraries and tools, many of which are open-source (开源的).
By exploring these aspects, you’ll not only gain a deeper understanding of Rust but also encounter various English terms and phrases that are essential in the tech industry. This dual focus makes learning Rust a rewarding experience on multiple fronts.
In the upcoming chapters, we will delve into (深入探讨) the basic syntax and features of Rust, starting with variable binding and immutability.
Stay tuned!
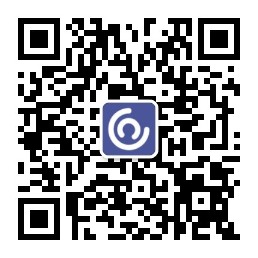
Chapter 2: Variable Binding and Immutability
Variable Declaration and Initialization
In Rust, declaring a variable is straightforward. You use the let
keyword to declare a variable. By default, variables are immutable, meaning they cannot be changed once they are initialized.
let x = 5;
Here, x
is an immutable variable initialized with the value 5
.
Immutable Variables
Immutable variables are variables that cannot be changed once they are declared. This is the default behavior in Rust and is different from languages like C++ where variables are mutable by default.
let y = 6;
// This will throw an error
y = 7;
Attempting to change the value of an immutable variable y
will result in a compilation error.
Mutable Variables
If you need a variable that can change its value, you can declare it as mutable using the mut
keyword.
let mut z = 8;
z = 9; // This is allowed
Here, z
is a mutable variable, and changing its value is permitted.
Variable Scope in Rust (作用域)
Just like in C++, variables in Rust have a scope, which is the range within the program where they are accessible.
{
let a = 10; // 'a' is accessible only within this block
}
// 'a' is not accessible here
In the above example, the variable a
is only accessible within the block where it is declared.
By understanding these fundamental concepts about variable binding and immutability, you’re taking the first steps into the world of Rust programming. These principles are not just syntactic sugar; they are part of Rust’s core philosophy to ensure memory safety and efficient code execution.
Chapter 3: Data Types in Rust
3.1 Scalar Types (标量类型)
Integers (整型)
In Rust, integers can be signed or unsigned, ranging from 8 to 64 bits. The default integer type is i32
, which is generally the fastest, even on 64-bit systems.
let x: i32 = 42;
let y: u64 = 1234567890;
Floating-Point Numbers (浮点型)
Rust provides two primitive types for floating-point numbers: f32
and f64
. The default is f64
because it offers more precision.
let x = 3.14; // f64 by default
let y: f32 = 2.71;
Booleans (布尔型)
The boolean type in Rust has two values, true
and false
. They are often used in conditional statements.
let is_true = true;
let is_false: bool = false;
Characters (字符型)
In Rust, the char
type represents a Unicode scalar value, which is more than just ASCII.
let a = 'a';
let omega = 'Ω';
3.2 Compound Types (复合类型)
Tuples (元组)
A tuple is a collection of values with different types. Tuples are immutable by default.
let tuple: (i32, f64, char) = (42, 6.28, 'J');
Arrays (数组)
An array is a collection of elements with the same type. The size of an array is fixed.
let arr: [i32; 5] = [1, 2, 3, 4, 5];
Structs (结构体)
Structs are custom data types that let you name and package together multiple related values.
struct Point {
x: i32,
y: i32,
}
let origin = Point {
x: 0, y: 0 };
Enums (枚举)
Enums allow you to define a type by enumerating its possible variants.
enum Direction {
North,
South,
East,
West,
}
let dir = Direction::North;
Understanding these data types is crucial for effective programming in Rust. They serve as the building blocks for more complex data structures and algorithms. As you get more comfortable with these types, you’ll also become more fluent in the technical English terms that are commonly used in programming documentation and discussions.
Chapter 4: Function Definition and Invocation
Function Declaration (函数声明)
In Rust, functions are declared using the fn
keyword, followed by the function name, parameters, and return type. The syntax is quite straightforward:
fn function_name(param1: Type1, param2: Type2) -> ReturnType {
// Function body
}
For example, a function to add two integers would look like this:
fn add(a: i32, b: i32) -> i32 {
a + b
}
Parameters and Return Values (参数和返回值)
Rust functions can take zero or more parameters. The type of each parameter must be explicitly stated. The return type is optional; if omitted, the function returns ()
(unit).
// Function with no parameters and no return value
fn display_message() {
println!("Hello, world!");
}
// Function with parameters but no return value
fn greet(name: &str) {
println!("Hello, {}!", name);
}
Function Invocation (函数调用)
Calling a function in Rust is similar to other languages like C++:
let result = add(5, 10);
You can also use named arguments for better readability:
let result = add(a: 5, b: 10);
Higher-Order Functions (高阶函数)
Rust supports higher-order functions, meaning you can pass functions as arguments to other functions or return them.
fn apply<F>(f: F, x: i32) -> i32
where
F: Fn(i32) -> i32,
{
f(x)
}
let square = |x| x * x;
let result = apply(square, 5);
In this chapter, we’ve covered the basics of defining and invoking functions in Rust. Understanding these concepts is crucial for writing efficient and clean code. Stay tuned for the next chapter, where we’ll delve into comments and documentation in Rust.
Chapter 5: Comments and Documentation
Line Comments and Block Comments (行注释和块注释)
In Rust, comments are essential for code readability and maintenance. There are two types of comments:
-
Line Comments: These start with
//
and extend to the end of the line.// This is a line comment.
-
Block Comments: These start with
/*
and end with*/
, spanning multiple lines./* This is a block comment. It spans multiple lines. */
Documentation Comments (文档注释)
Rust also supports documentation comments that are useful for generating documentation automatically. These comments start with ///
for single-line documentation or //!
for module-level documentation.
/// This function adds two numbers.
///
/// # Examples
///
/// ```
/// let sum = add(3, 4);
/// assert_eq!(sum, 7);
/// ```
pub fn add(a: i32, b: i32) -> i32 {
a + b
}
Auto-generating Documentation (自动生成文档)
You can generate HTML documentation for your Rust code using the cargo doc
command. This will produce an HTML file that you can open in a web browser to view the documentation.
cargo doc --open
Best Practices (最佳实践)
- Be Concise (简洁): Keep your comments short and to the point.
- Be Clear (清晰): Use simple language to describe complex logic.
- Avoid Redundancy (避免冗余): Don’t state the obvious; comments should provide additional insights.
By incorporating comments and documentation into your Rust code, you not only make the code more understandable but also create a valuable resource for yourself and others in the future. This practice aligns well with the philosophy of “code as documentation,” making your programming journey more effective and insightful.
结语
在我们的编程学习之旅中,理解是我们迈向更高层次的重要一步。然而,掌握新技能、新理念,始终需要时间和坚持。从心理学的角度看,学习往往伴随着不断的试错和调整,这就像是我们的大脑在逐渐优化其解决问题的“算法”。
这就是为什么当我们遇到错误,我们应该将其视为学习和进步的机会,而不仅仅是困扰。通过理解和解决这些问题,我们不仅可以修复当前的代码,更可以提升我们的编程能力,防止在未来的项目中犯相同的错误。
我鼓励大家积极参与进来,不断提升自己的编程技术。无论你是初学者还是有经验的开发者,我希望我的博客能对你的学习之路有所帮助。如果你觉得这篇文章有用,不妨点击收藏,或者留下你的评论分享你的见解和经验,也欢迎你对我博客的内容提出建议和问题。每一次的点赞、评论、分享和关注都是对我的最大支持,也是对我持续分享和创作的动力。
阅读我的CSDN主页,解锁更多精彩内容:泡沫的CSDN主页