@RequestBody
主要用来接收前端传递给后端的json字符串中的数据的(请求体中的数据的);GET方式无请求体,所以使用@RequestBody接收数据时,前端不能使用GET方式提交数据,而是用POST方式进行提交。
示例:
@RestController
public class TestController{
@RequestMapping("\myqxin")
@ResponseBody
public RetureResult test(@RequestBody User user){
return new ReturnResult();
}
}
这样的方式只能接收一个json对象,有时我们需要接收多个json对象。
示例:
@RestController
public class TestController{
@RequestMapping("\myqxin")
@ResponseBody
public RetureResult test(@RequestBody List<User> user){
return new ReturnResult();
}
}
这样能接收多个同类型的json对象,但是有时我们需要接收的对象是不同类型的。需要接收多个不同的json对象
示例:
@RestController
public class TestController{
@RequestMapping("\myqxin")
@ResponseBody
public RetureResult test(@RequestBody User user,@RequestBody Address address){
return new ReturnResult();
}
}
上面这种方式就是错误的,原因是request的content-body是以流的形式进行读取的,读取完一次后,便无法再次读取了。
解决方式一
增加一个包装类,讲所需要的类写入,提供get/set
示例:
@RestController
public class TestController{
@RequestMapping("\myqxin")
@ResponseBody
public RetureResult test(@RequestBody Param param){
User user=param.getUser();
Address address=param.getAddress();
return new ReturnResult();
}
}
class Param{
private User user;
private Address address;
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
解决方式二
前端请求格式如下:
{
"productFormInfos":[
{
"attactMon": "3",
"claimP": "0.7",
"insuredAmt": "100000",
"insuredP": "0.2",
"notPayValue": "20000",
"type": "健康体",
"usedDrug": "",
"waitMon1": "1",
"waitMon2": "5",
"waitMon3": "5"
},
{
"attactMon": "",
"claimP": "0.3",
"insuredAmt": "100000",
"insuredP": "1",
"notPayValue": "20000",
"type": "带病体",
"usedDrug": "0.2",
"waitMon1": "",
"waitMon2": "",
"waitMon3": ""
}],
"cancerAttackInfos":[
{
"cancerType": "全癌种",
"value": 1
},
{
"cancerType": "多发性骨髓瘤",
"value": -0.7
}],
"znjsRegionInfos":[
{
"age1": "0.041985",
"age2": "0.0444276510925037",
"age3": "0.0382427339114538",
"age4": "0.0261105159086444",
"age5": "0.0449479905248563",
"age6": "0.0772911243564838",
"age7": "0.0810856929668603",
"age8": "0.0765560498761178",
"age9": "0.0749054098877601",
"age10": "0.0871027056596078",
"age11": "0.0865625242702434",
"age12": "0.0688175656296236",
"age13": "0.0715534521423089",
"age14": "0.0687895196577188",
"age15": "0.0452628418871741",
"age16": "0.0279018803309113",
"age17": "0.0219935342690072",
"age18": "0.0117872756063724",
"age19": "0.00467652625930834",
"age20": "0",
"age21": "0",
"livePopulation": "1001",
"mediInsuredPlt": "1549.7678",
"type": "城镇职工",
"updateTime": "2021-04-21T03:01:04.108Z",
"domicilePopulation": "1002",
"subUseP": "1",
"treatP": "1",
"treatPD": "1",
"treatPlanP": "1",
"treatPlanPD": "1",
"byStageP": "1",
"byStagePD": "1",
"diagnosisP": "1",
"diagnosisPD": "1"
},{
"age1": "0.041985",
"age2": "0.0444276510925037",
"age3": "0.0382427339114538",
"age4": "0.0261105159086444",
"age5": "0.0449479905248563",
"age6": "0.0772911243564838",
"age7": "0.0810856929668603",
"age8": "0.0765560498761178",
"age9": "0.0749054098877601",
"age10": "0.0871027056596078",
"age11": "0.0865625242702434",
"age12": "0.0688175656296236",
"age13": "0.0715534521423089",
"age14": "0.0687895196577188",
"age15": "0.0452628418871741",
"age16": "0.0279018803309113",
"age17": "0.0219935342690072",
"age18": "0.0117872756063724",
"age19": "0.00467652625930834",
"age20": "0",
"age21": "0",
"livePopulation": "1001",
"mediInsuredPlt": "349.83",
"type": "城乡居民",
"domicilePopulation": "1002",
"subUseP": "1",
"treatP": "1",
"treatPD": "1",
"treatPlanP": "1",
"treatPlanPD": "1",
"byStageP": "1",
"byStagePD": "1",
"diagnosisP": "1",
"diagnosisPD": "1"
}]
}
将接收参数定义为Map<String, Object>,然后使用map转object工具,转换成需要的对象。
扫描二维码关注公众号,回复:
16930105 查看本文章
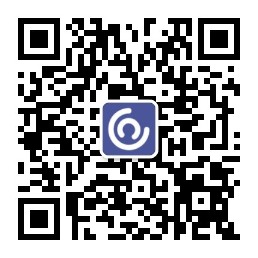
此时,即使自定义的Param类中的属性即使比json数据中的属性少了,也没关系。
示例:
@RestController
public class TestController{
@RequestMapping("\myqxin")
@ResponseBody
public Object test(@RequestBody Map<String, Object> models){
String json1 = JSON.toJSONString(models.get("znjsRegionInfos"));
String json2 = JSON.toJSONString(models.get("productFormInfos"));
String json3 = JSON.toJSONString(models.get("cancerAttackInfos"));
// 转json,这里接收的每个同类型对象都有多个,所以是个集合,需要转json
List<ZnjsRegionInfo> znjsRegionInfos = JSON.parseArray(json1, ZnjsRegionInfo.class);
List<ProductFormInfo> productFormInfos = JSON.parseArray(json2, ProductFormInfo.class);
List<CancerAttackInfo> cancerAttackInfos = JSON.parseArray(json3, CancerAttackInfo.class);
return models;
}
}