c语言 成绩管理系统
#include<stdio.h>
#include<malloc.h>
#include<windows.h>
#define LEN sizeof(struct Student)
struct Student{
long num;
char name[20];
char sex[20];
float chinese;
float math;
float english;
struct Student *next;
}student={
1001,"Wang","男",100,100,100,NULL};
void printLine(int n,char a){
for(int i=0;i<n;i++){
printf("%c",a);
}
printf("\n");
}
int isExist(struct Student *head,int num){
struct Student *p=head;
while(p!=NULL){
if(p->num==num){
return 1;
}
p=p->next;
}
return 0;
}
struct Student *addInfo(struct Student *head){
struct Student *p=head;
if(p!=NULL){
for(;p->next!=NULL;p=p->next);
p->next=(struct Student*)malloc(LEN);
p=p->next;
printLine(50,'-');
printf("请依次输入学号,姓名,性别,语文,数学,英语(空格分开):\n");
scanf("%d %s %s %f %f %f",&p->num,&p->name,&p->sex,&p->chinese,&p->math,&p->english);
p->next=NULL;
return head;
}else{
p=(struct Student*)malloc(LEN);
printf("请依次输入学号,姓名,性别,语文,数学,英语(空格分开):\n");
scanf("%d %s %s %f %f %f",&p->num,&p->name,&p->sex,&p->chinese,&p->math,&p->english);
p->next=NULL;
return p;
}
}
struct Student *deleteInfo(struct Student *head){
struct Student *p=head,*next;
long num;
printLine(50,'-');
printf("请输入需要删除学生的学号(0取消删除):");
scanf("%ld",&num);
if(num==0){
return head;
}
if(p->num==num){
head=p->next;
return head;
}
while(p!=NULL){
next=p->next;
if(next->num==num){
p->next=next->next;
return head;
}
p=next;
}
}
struct Student *searchInfo(struct Student *head){
struct Student *p=head;
long num;
printLine(50,'-');
printf("请输入需要查询学生的学号(0取消删除):");
scanf("%ld",&num);
if(num==0){
return head;
}
for(;p!=NULL;p=p->next){
if(p->num==num){
printLine(50,'-');
printf("%-8ld%-10s%-9s%-9.1f%-9.1f%-8.1f\n",p->num,p->name,p->sex,p->chinese,p->math,p->english);
return head;
}
}
};
struct Student *updateInfo(struct Student *head){
struct Student *p=head,*up,*next;
long num;
printLine(50,'-');
printf("请输入需要修改学生的学号(0取消修改):");
scanf("%ld",&num);
if(num==0){
return head;
}
printLine(50,'-');
if(p->num==num){
up=(struct Student*)malloc(LEN);
printf("请依次输入学号,姓名,性别,语文,数学,英语(空格分开):\n");
scanf("%d %s %s %f %f %f",&up->num,&up->name,&up->sex,&up->chinese,&up->math,&up->english);
up->next=head->next;
return up;
}
while(p!=NULL){
next=p->next;
if(next!=NULL&&next->num==num){
up=(struct Student*)malloc(LEN);
printf("请依次输入学号,姓名,性别,语文,数学,英语(空格分开):\n");
scanf("%d %s %s %f %f %f",&up->num,&up->name,&up->sex,&up->chinese,&up->math,&up->english);
p->next=up;
up->next=next->next;
return head;
}
p=next;
}
return head;
}
void printAllInfo(struct Student *head){
struct Student *p=head;
printLine(50,'-');
printf("学号 姓名 性别 语文 数学 英语\n");
while(p!=NULL){
printf("%-8ld%-10s%-9s%-9.1f%-9.1f%-8.1f\n",p->num,p->name,p->sex,p->chinese,p->math,p->english);
p=p->next;
}
}
void controlTab(struct Student *head,int clear,int echo){
struct Student *p=head;
while(1){
if(clear){
system("cls");
printf("\n*******************成绩管理系统*******************\n");
printLine(50,'-');
printf(" *1 刷系数据\n");
printf(" *2 查询指定数据\n");
printf(" *3 添加新数据\n");
printf(" *4 更新指定数据\n");
printf(" *5 删除指定数据\n");
clear=0;
}
if(echo){
printLine(50,'-');
printAllInfo(p);
echo=0;
}
int operation;
printLine(50,'-');
printf("请输入你所需要进行的操作:");
scanf("%d",&operation);
switch(operation){
case 1:{
clear=1;
echo=1;
break;
}
case 2:{
searchInfo(p);
clear=0;
echo=0;
break;
}
case 3:{
p=addInfo(p);
clear=1;
echo=1;
break;
}
case 4:{
p=updateInfo(p);
clear=1;
echo=1;
break;
}
case 5:{
p=deleteInfo(p);
clear=1;
echo=1;
break;
}
}
}
}
int main(){
controlTab(&student,1,1);
return 0;
}
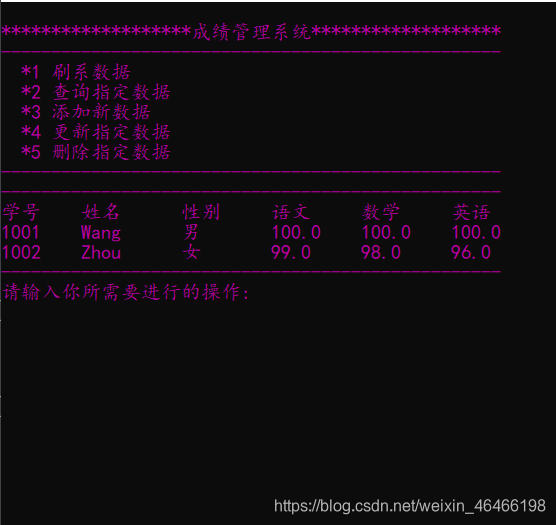