int文件对于大多数人来说应该不陌生,一般大型的软件中有些配置文件使用的是ini格式文件。Qt也提供了ini文件的创建,元素的增加和删除获取等功能。
ini中的数据一般包含组,组中包含的key和value值。
在Qt中使用的是类QSettings,这个类就实现了ini格式文件操作的相关函数。
在qt的官方文档中有说明QSettings类有两种使用方式,一中是将其属性通过QSettings配置到系统的注册表中,另一种就是保存为本地的ini文件。
使用QSettings类将配置保存到注册表中
使用步骤如下:
1、使用QCoreApplication::setOrganizationName(“公司名称”);和QCoreApplication::setApplicationName(“应用名称”);
2、直接使用QSettings无参构造对象。
3、使用QSettings的setValue()函数设置属性值。
4、使用QSettings的value获取属性值。
例如,如果你的产品名为Star Runner,而你的公司名为MySoft。(这是qt中的例子)
QCoreApplication::setOrganizationName("MySoft");
QCoreApplication::setApplicationName("Star Runner");
...
QSettings settings;
以上是第一步,这一步执行之后小编估计就会在注册表中创建出对应的注册表结构 MySoft–>Star Runner。
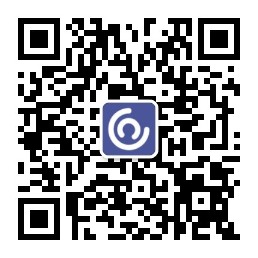
之后在项目的任何位置就可以使用QSettings对象创建和获取软件的属性值了。
settings.setValue("editor/wrapMargin", 68);//设置属性值
int margin = settings.value("editor/wrapMargin").toInt();//获取属性值
如果QSettings中有分组的话可以使用一下方式。例如有一个sensor的组,sensorType为属性,camera为属性值。
设置属性值为:
//m_settings是QSettings的对象
m_settings->beginGroup("sensor"); //打开组,如果没有就创建一个
m_settings->setValue("sensorType","camera");//设置属性和值
m_settings->endGroup();//结束值设置
获取属性值:
m_settings->beginGroup("sensor");//打开组
value = m_settings->value("sensorType").toString();//获取属性值
m_settings->endGroup();
至此,注册表的属性设置就完成了,这里需要注意的就是各个系统中注册表的区别。
还有就是通过以上方式设置的属性值夜可以直接通过注册表进行访问,以上方法主要适用于设置全局通用的属性,使用以上方法相对方便。
直接访问ini文件
直接使用ini本地文件和写到注册标准操作流程差不多相似,只是在创建QSettings对象的时候不一样。
1、使用QSettings类创建对象,在创建对象的过程中需要引入两个参数(文件存储路径和文件格式)。
2、使用QSettings的setValue()函数设置属性值。
3、使用QSettings的value获取属性值。
这种使用方式可以方便配置文件的修改,但是在软件使用过程中无法做到全局通用,不过可以使用C++的单例模式实现全局唯一的使用方式。
例如:创建一个配置文件为myapp.ini的文件,路径为/home/petra/misc/myapp.ini的配置文件。
QSettings settings("/Users/petra/misc/myapp.plist",
QSettings::NativeFormat);//创建QSettings对象
之后在项目的任何位置就可以使用QSettings对象创建和获取软件的属性值了。
settings.setValue("editor/wrapMargin", 68);//设置属性值
int margin = settings.value("editor/wrapMargin").toInt();//获取属性值
如果QSettings中有分组的话可以使用一下方式。例如有一个sensor的组,sensorType为属性,camera为属性值。
设置属性值为:
//m_settings是QSettings的对象
m_settings->beginGroup("sensor"); //打开组,如果没有就创建一个
m_settings->setValue("sensorType","camera");//设置属性和值
m_settings->endGroup();//结束值设置
获取属性值:
m_settings->beginGroup("sensor");//打开组
value = m_settings->value("sensorType").toString();//获取属性值
m_settings->endGroup();
至此就配置文件就设置完成了。
以下是小编封装的一个单例模式的类:
#ifndef CONFIGUREFILE_H
#define CONFIGUREFILE_H
#include <QString>
#include <QSettings>
class configureFile
{
public:
/**
* @brief writeConfig 向配置文件中添加数据
* @param group 所要添加的分类
* @param key 所要添加的键
* @param value 所要添加的值
*/
void writeConfig(QString group,QString key,QString value);
/**
* @brief readConfig 获取配置文件中对应键值的数据
* @param group 分类
* @param key 键
* @return 值
*/
QString readConfig(QString group,QString key);
/**
* @brief getInterface 获取配置文件对象
* @return 返回配置文件对象
*/
static configureFile* getInterface();
private:
configureFile();
~configureFile();
QSettings * m_settings = nullptr;
static configureFile * m_configFile;
};
#endif // CONFIGUREFILE_H
#include "configurefile.h"
configureFile* configureFile::m_configFile = nullptr;
configureFile::configureFile()
{
if(m_settings == nullptr){
m_settings = new QSettings("./hwots.ini",QSettings::IniFormat);
}
}
configureFile::~configureFile()
{
if(m_settings != nullptr){
delete m_settings;
}
}
void configureFile::writeConfig(QString group, QString key, QString value)
{
m_settings->beginGroup(group);
m_settings->setValue(key,value);
m_settings->endGroup();
}
QString configureFile::readConfig(QString group, QString key)
{
QString value;
m_settings->beginGroup(group);
value = m_settings->value(key).toString();
m_settings->endGroup();
return value;
}
configureFile* configureFile::getInterface()
{
if(m_configFile == nullptr){
m_configFile = new configureFile();
}
return m_configFile;
}