目录
介绍
Rust的时间操作主要用到chrono库,接下来我将简单选一些常用的操作进行介绍,如果想了解更多细节,请查看官方文档。
官方文档:chrono - Rust
Cargo.toml引用:chrono = { version = "0.4", features = ["serde"] }
扫描二维码关注公众号,回复:
16854823 查看本文章
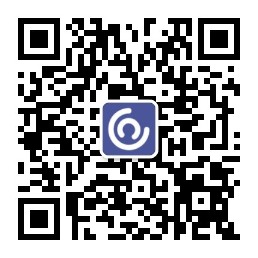
一、计算耗时
Rust标准库,一般用于计算变量start和duration之间的程序运行时间,代码如下:
use std::time::{Duration, Instant};
use std::thread;
fn expensive_function(seconds:u64) {
thread::sleep(Duration::from_secs(seconds));
}
fn main() {
cost();
}
fn cost(){
let start = Instant::now();
expensive_function(2);
let duration = start.elapsed();
println!("耗时: {:?}", duration);
}
二、时间加减法
使用到chrono库的checked_add_signed方法,如果无法计算出日期和时间,方法将返回 None。比如当前时间加一天、加两周、加3小时再减4秒,代码如下:
use chrono::{Duration, Local};
fn main() {
// 获取当前时间
let now = Local::now();
println!("{}", now);
let almost_three_weeks_from_now = now.checked_add_signed(Duration::days(1))
.and_then(|in_2weeks| in_2weeks.checked_add_signed(Duration::weeks(2)))
.and_then(|in_2weeks| in_2weeks.checked_add_signed(Duration::hours(3)))
.and_then(|in_2weeks| in_2weeks.checked_add_signed(Duration::seconds(-4)))
;
match almost_three_weeks_from_now {
Some(x) => println!("{}", x),
None => eprintln!("时间超出范围"),
}
match now.checked_add_signed(Duration::max_value()) {
Some(x) => println!("{}", x),
None => eprintln!("时间超出范围,不能计算出太阳系绕银河系中心一周以上的时间."),
}
}
三、时区转换
使用 chrono库的DateTime::from_naive_utc_and_offset 方法将本地时间转换为 UTC 标准格式。然后使用 offset::FixedOffset 结构体,将 UTC 时间转换为 UTC+8 和 UTC-2。
use chrono::{DateTime, FixedOffset, Local, Utc};
fn main() {
let local_time = Local::now();
let utc_time = DateTime::<Utc>::from_naive_utc_and_offset(local_time.naive_utc(), Utc);
let china_timezone = FixedOffset::east_opt(8 * 3600);
let rio_timezone = FixedOffset::west_opt(2 * 3600);
println!("本地时间: {}", local_time);
println!("UTC时间: {}", utc_time);
println!(
"北京时间: {}",
utc_time.with_timezone(&china_timezone.unwrap())
);
println!("里约热内卢时间: {}", utc_time.with_timezone(&rio_timezone.unwrap()));
}
四、年月日时分秒
获取当前时间年月日、星期、时分秒,使用chrono库:
use chrono::{Datelike, Timelike, Local};
fn main() {
let now = Local::now();
let (is_common_era, year) = now.year_ce();
println!(
"当前年月日: {}-{:02}-{:02} {:?} ({})",
year,
now.month(),
now.day(),
now.weekday(),
if is_common_era { "CE" } else { "BCE" }
);
let (is_pm, hour) = now.hour12();
println!(
"当前时分秒: {:02}:{:02}:{:02} {}",
hour,
now.minute(),
now.second(),
if is_pm { "PM" } else { "AM" }
);
}
五、时间格式化
时间格式化会用到chrono库,用format方法进行时间格式化;NaiveDateTime::parse_from_str方法进行字符串转DateTime,代码如下:
use chrono::{DateTime, Local, ParseError, NaiveDateTime};
fn main() -> Result<(), ParseError>{
let now: DateTime<Local> = Local::now();
// 时间格式化
let ymdhms = now.format("%Y-%m-%d %H:%M:%S%.3f");
// 字符串转时间
let no_timezone = NaiveDateTime::parse_from_str("2015-09-05 23:56:04.800", "%Y-%m-%d %H:%M:%S%.3f")?;
println!("当前时间: {}", now);
println!("时间格式化: {}", ymdhms);
println!("字符串转时间: {}", no_timezone);
Ok(())
}
Rust的时间与日期操作就简单介绍到这里,关注不迷路(*^▽^*)