1.功能介绍
检查用户是否登录,根据是否登录,决定显示的内容。
如果用户未登录,显示“注册 | 登录”;如果用户已登录:显示“Welcome xxx | 注销”。
2.实现步骤及代码
2.1 创建一个网络请求的服务
http.service.ts
//a-service-http快捷键
import { Injectable } from '@angular/core';
import { Http,Response } from '@angular/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/operator/map';
import 'rxjs/add/operator/catch';
@Injectable()
export class HttpService {
//实例化Http类
constructor(private http:Http) { }
//封装方法,参数为字符串类型的请求数据地址
sendRequest(myUrl:string){
//a-http-get快捷键
return this.http.get(myUrl,{withCredentials:true})
.map((response:Response)=>response.json());
//{withCredentials:true}指定证书,允许获取跨域中的session数据,客户端设置true
}
}
2.2 在根模块中引入服务
app.module.ts
import {HttpService} from './utility/service/http.service';
@NgModule({
providers:[
HttpService
]
})
2.3 在组件中使用服务
header.component.ts
import { Component, OnInit } from '@angular/core';
//将服务注入到组件中
import { HttpService } from '../service/http.service';
@Component({
selector: 'storeHeader',
templateUrl: './header.component.html',
styleUrls:['./header.css']
})
export class HeaderComponent implements OnInit {
//保存登录状态的数据,false表示未登录,true表示已经登录
isLogin:boolean=true;
//保存用户名的数据
uname:string='';
//实例化Http类
constructor(private myHS:HttpService) { }
ngOnInit() {
//发送请求,使用http服务
this.myHS.sendRequest('http://127.0.0.1/xxx-project/data/users/session_data.php').subscribe((result:any)=>{
//如果result.uname中有数据,说明已登录,把用户名读取到头部,如果没有说明未登录
if(result.uname){
this.isLogin=true;
this.uname=result.uname;
}
})
}
}
2.4 将数据显示到模板中
<li *ngIf="!isLogin">
<a href="register.html">注册</a>
<a>|</a>
</li>
<li *ngIf="!isLogin">
<a href="login.html">登录</a>
</li>
<li *ngIf="isLogin">
<a href="javascript:;">
Welcome {{uname}}
</a>
<a>|</a>
</li>
<li *ngIf="isLogin">
<a id="logout" href="javascript:;">注销</a>
</li>
2.5 在服务器端解决跨域问题
init.php
<?php
//解决跨域
header('Access-Control-Allow-Origin:http://localhost:3000');
//在服务器端颁发证书,设置true,可以获取session数据
header('Access-Control-Allow-Credentials:true');
2.6 在服务器端存储用户登录数据
session_data.php
<?php
header('Content-Type: application/json;charset=UTF-8');
//引用公共文件init.php
require('../init.php');
session_start();
//从session中获取uid和uname
@$output['uid'] = $_SESSION['uid'];
@$output['uname'] = $_SESSION['uname'];
//返回当前登录用户的信息
echo json_encode($output);
3.相关知识点
Angular的工作方式
- 用 Angular 扩展语法编写 HTML 模板
- 用组件类管理这些模板
- 用服务添加应用逻辑
- 用模块打包发布组件与服务
数据绑定
将数据绑定到视图上面,如果数据发生了修改,视图就会自动的更新。
数据绑定的实现方式:所有的指令和双花括号都有数据绑定的功能。
数据绑定的原理:object.defineProperty
Angular选择指令
语法:
<any *ngIf="expression"></any>
功能:根据表达式执行的结果的真假来决定 是否要将该标签添加到DOM。
多重分支判断:*ngSwitchCase *ngSwitchDefault
服务 service
服务类角色:在angular中把组件中应用逻辑封装在不同的服务类中.
(组件封装的目的是为了复用视图,而服务封装的目的是为了复用数据和方法。)
服务类最大的消费者是组件。
扫描二维码关注公众号,回复:
1679620 查看本文章
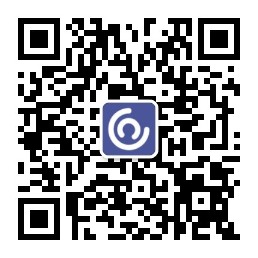
相关内容:确认登录及注销功能的代码(前端+后端)
上文:购物车页面的功能(php+Ajax+jQuery)
更多内容,欢迎关注微信公众号“让知识成为资产”。