文件相关操作
1.说明
这个是一个文件操作相关的工具类方法分享,主要是有一些小的点:
- 最新文件不扫码
- 写出文件使用固定编码
2.工具详情
方法可能并不通用,特别是最后的根据参数清理文件夹,里边有专门对文件夹路径的判断。
2.1 根据路径获取指定数量的文件列表
此方法会扫码rootPath
下的所有文件,根据fileCount
获取指定数量的文件:
- 可添加对文件的筛选(根据文件名称包含某些字符串)
- 不能用在多线程的场景
private List<File> files = new ArrayList<>();
/**
* 根据路径获取指定数量的文件列表
*
* @param rootPath 目录
* @param fileCount 数量
* @return 文件列表
*/
public List<File> getFiles(String rootPath, int fileCount) {
List<File> fileList = new ArrayList<>();
Path rootDir = Paths.get(rootPath);
try {
DirectoryStream<Path> paths = Files.newDirectoryStream(rootDir);
for (Path path : paths) {
File file = path.toFile();
if (file.isDirectory()) {
fileList.addAll(getFiles(file.getAbsolutePath(), fileCount - fileList.size()));
} else {
if (files.contains(file)) {
continue;
}
if (System.currentTimeMillis() - file.lastModified() < 10000) {
continue;
}
files.add(file);
fileList.add(file);
}
if (fileList.size() >= fileCount) {
break;
}
}
paths.close();
files.clear();
} catch (Exception e) {
e.printStackTrace();
}
return fileList;
}
2.2 复制文件
这个是非常常用的方法。
/**
* 复制文件
*
* @param sourceFile 源文件
* @param targetFile 目标文件
* @return 是否复制成功
*/
public boolean fileCopyToNewFile(File sourceFile, File targetFile) {
boolean success = false;
try {
FileUtils.copyFile(sourceFile, targetFile);
success = true;
} catch (IOException e) {
e.printStackTrace();
log.error("fileCopyToNewFile Failed!");
}
return success;
}
2.3 根据参数创建文件夹
非常常用,不再解释。
/**
* 根据参数创建文件夹
*
* @param dirPath 文件夹路径
* @param describe 文件夹描述
*/
public void creatDirByParam(String dirPath, String describe) {
// 获取文件夹路径
File file = new File(dirPath);
// 判断文件夹是否创建,没有创建则创建新文件夹
if (!file.exists()) {
if (file.mkdirs()) {
log.info(" - - - - - - 创建{} [{}] - - - - - - ", describe, dirPath);
}
}
}
2.4 根据图片路径获取图片
根据图片路径将图片展示在浏览器。
/**
* 根据图片路径获取图片
*
* @param imagePath 图片路径
* @return 图片数据
*/
public ResponseEntity<byte[]> getImageByPath(String imagePath) {
File file = new File(imagePath);
byte[] imageBytes = {
};
try {
imageBytes = Files.readAllBytes(file.toPath());
} catch (IOException e) {
e.printStackTrace();
}
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.IMAGE_JPEG);
return new ResponseEntity<>(imageBytes, headers, HttpStatus.OK);
}
调用方法:
@GetMapping(value = "/getImageByImagePath", produces = "application/json;charset=UTF-8")
public Map<String, Object> getImageByImagePath(String pic) {
return getImageByImagePath(pic);
}
2.5 根据参数输出文件
使用固定的编码格式输出文件。
/**
* 根据参数输出文件
*
* @param filePath 文件路径
* @param data 数据对象
* @param size 写出的条数
*/
public static void writeDataToFile(String filePath, LinkedBlockingQueue<String> data, int size) {
try {
// 创建 BufferedWriter 对象,提高写入性能
BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(filePath), StandardCharsets.UTF_8));
// 写入数据
for (int i = 0; i < size; i++) {
bufferedWriter.write(data.take());
if (i < size - 1) {
// 写入换行符
bufferedWriter.newLine();
}
}
// 关闭资源
bufferedWriter.close();
log.info("------writeDataToFile [{}] 条!------", size);
} catch (IOException | InterruptedException e) {
log.error("------writeDataToFile Failed [{}]------", e.getMessage());
}
}
2.6 根据参数清理文件夹
当前方法有特定的判断,不是通用方法。
扫描二维码关注公众号,回复:
16771906 查看本文章
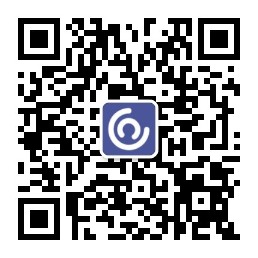
/**
* 根据参数清理文件夹
*
* @param rootPath 根目录
* @param keepDays 保存日期
*/
public void deleteFolderByParam(String rootPath, int keepDays) {
try {
String currentDay = DateUtil.format(DateUtil.offsetDay(new Date(), -keepDays), "yyyyMMdd");
int currentDayInt = Integer.parseInt(currentDay);
File[] devPathArr = new File(rootPath).listFiles();
if (devPathArr != null && devPathArr.length > 0) {
for (File devPath : devPathArr) {
if (devPath.isDirectory()) {
File[] dayPathArr = devPath.listFiles();
if (dayPathArr != null && dayPathArr.length > 0) {
for (File dayPath : dayPathArr) {
if (dayPath.isDirectory()) {
int dirName = Integer.parseInt(dayPath.getName());
if (dirName < currentDayInt) {
File[] files = dayPath.listFiles();
if (files != null && files.length > 0) {
for (File file : files) {
FileUtils.delete(file);
log.info("deleteFolder[{}]file[{}]", dirName, file.getName());
}
}
}
}
}
}
}
}
}
} catch (Exception e) {
log.error("deleteFolderByParam Failed! [{}]", e.getMessage());
}
}
3.总结
文件相关的操作是很常用的,会不断进行补充。