一、基础组件
import 'package:flutter/material.dart';
//容器闪烁
class ContainerFlash extends StatefulWidget {
final Widget child;
final bool play; //是否播放
final int duration; //动画时长(毫秒)
final double speed; //动画速度
final Color initColor; //初始颜色
final Color flashColor; //闪烁颜色
final bool loop; //是否循环播放
ContainerFlash(
{Key key,
this.child,
this.play = false,
this.duration = 2000,
this.speed = 1.0,
this.initColor,
this.flashColor,
this.loop = false})
: super(key: key);
@override
_ContainerFlashState createState() => _ContainerFlashState();
}
class _ContainerFlashState extends State<ContainerFlash>
with TickerProviderStateMixin {
AnimationController _animationController;
Animation<double> _animation;
int _singleAniPeriod; //单次动画时长
@override
void initState() {
super.initState();
//控制单次动画时长实现动画速度变化
_singleAniPeriod = (1000 / widget.speed).truncate();
_animationController = AnimationController(
vsync: this,
duration: Duration(milliseconds: _singleAniPeriod),
);
Animation curveAnimation =
new CurvedAnimation(parent: _animationController, curve: Curves.linear);
_animation = Tween<double>(begin: 0.0, end: 1.0).animate(curveAnimation);
}
@override
void dispose() {
_animationController.dispose();
_animationController = null;
super.dispose();
}
@override
void didUpdateWidget(ContainerFlash oldWidget) {
// TODO: implement didUpdateWidget
super.didUpdateWidget(oldWidget);
if (_animationController != null) {
if (widget.play) {
this._playAnimation();
} else {
_animationController.stop();
_animationController.reset();
}
}
}
@override
Widget build(BuildContext context) {
return Container(
child: ContainerFlashAnimation(
_animation, widget.child, widget.initColor, widget.flashColor),
);
}
//播放动画
_playAnimation() {
_animationController.reset();
_animationController.repeat();
if (widget.loop) return;
Future.delayed(Duration(milliseconds: widget.duration), () {
if (_animationController != null) {
_animationController.stop();
_animationController.reset();
}
});
}
}
class ContainerFlashAnimation extends AnimatedWidget {
final Widget child;
final Color initColor;
final Color flashColor;
ContainerFlashAnimation(
Animation animation, this.child, this.initColor, this.flashColor)
: super(listenable: animation);
@override
Widget build(BuildContext context) {
Animation animation = listenable;
int numVal = (animation.value * 10).truncate();
bool active = numVal % 2 == 1;
Color curColor = active
? (flashColor != null ? flashColor : Colors.white.withOpacity(0.2))
: (initColor != null ? initColor : Colors.transparent);
return Container(
padding: EdgeInsets.all(4),
decoration: BoxDecoration(
color: curColor, borderRadius: BorderRadius.all(Radius.circular(4))),
child: child != null ? child : Container(),
);
}
}
二、组件使用
import 'package:flutter/material.dart';
import './container_flash.dart';// 引入组件
class Example extends StatefulWidget {
const Example({Key key});
@override
State<Example> createState() => _ExampleState();
}
class _ExampleState extends State<Example> {
bool _flash = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('示例'),
centerTitle: true,
),
body: Container(
padding: EdgeInsets.all(20),
color: Colors.blue[900],
width: double.infinity,
child: Column(
children: <Widget>[
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
ContainerFlash(
play: _flash,
child: Text(
'请选择',
style: TextStyle(fontSize: 20),
),
),
ContainerFlash(
play: _flash,
duration: 5000, //持续时长
flashColor: Colors.orange.withOpacity(0.4), //闪烁颜色
child: Text(
'注意事项',
style: TextStyle(fontSize: 20),
),
),
ContainerFlash(
play: _flash,
speed: 2.0, //动画速度
child: FlatButton(
onPressed: () {},
color: Colors.orange,
child: Text('按钮'),
),
),
ContainerFlash(
play: _flash,
loop: true, //是否循环播放
child: Text(
'循环闪烁',
style: TextStyle(fontSize: 20),
),
),
],
),
SizedBox(height: 20),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
FlatButton(
onPressed: () {
_flash = true;
if (mounted) {
setState(() {});
}
},
color: Colors.green,
child: Text('闪烁'),
),
SizedBox(width: 20),
FlatButton(
onPressed: () {
_flash = false;
if (mounted) {
setState(() {});
}
},
color: Colors.red,
child: Text('停止闪烁'),
),
],
)
],
),
),
);
}
}
三、效果演示
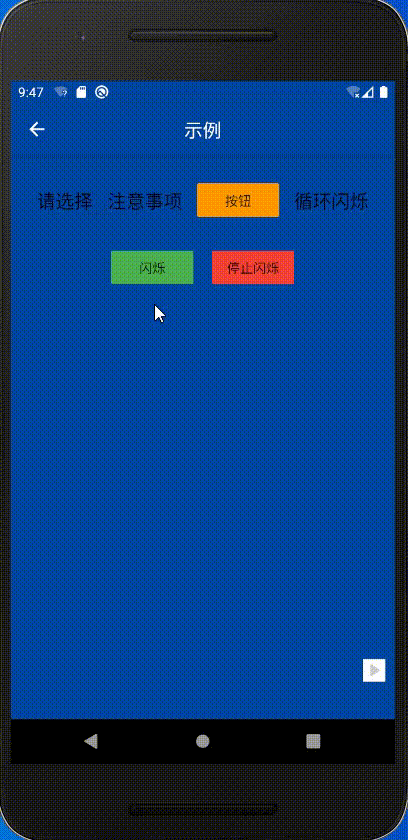