WPF属性笔记系列
第一章 【WPF属性笔记】【IsHitTestVisible】
第二章 【WPF属性笔记】【DataTrigger】
前言
关于WPF属性DataTrigger的学习笔记。
一、DataTrigger介绍
- WPF中,根据数据的值不同,UI的界面随之改变(显示控件、隐藏控件以及改变控件的其他属性),这时我们可以用DataTrigger触发器
二、DataTrigger的使用
下面的案例实现功能,根据条件(数据的值)不同时,显示不同的按钮。
从案例的运行效果图中可以看到,当Condition的值变化时,将会触发xaml中的事件,从而改变控件原本的显示状态。
xaml:
<Window.Resources>
<local:Core x:Key="core" />
<Style TargetType="{x:Type Button}">
<Setter Property="Margin" Value="5" />
<Setter Property="Padding" Value="0,8.5" />
<Setter Property="FontSize" Value="16" />
</Style>
</Window.Resources>
<Grid>
<ContentControl>
<ContentControl.ContentTemplate>
<DataTemplate>
<StackPanel>
<WrapPanel HorizontalAlignment="Center">
<Button Content="条件1" Click="Button_Click_1"/>
<Button Content="条件2" Click="Button_Click_2"/>
<Button Content="条件3" Click="Button_Click_3"/>
</WrapPanel>
<TextBlock Text="按钮列表" HorizontalAlignment="Center" FontSize="18"/>
<TextBlock HorizontalAlignment="Center" VerticalAlignment="Center" Foreground="Red" FontSize="16" Text="{Binding Source={StaticResource ResourceKey=core},Path=Condition}"/>
<CheckBox x:Name="cb1" IsChecked="True" HorizontalAlignment="Center" Visibility="Collapsed"/>
<TextBox x:Name="tb2" Text="666666" HorizontalAlignment="Center" Visibility="Collapsed"/>
<ComboBox x:Name="cbb3" HorizontalAlignment="Center" Visibility="Collapsed"/>
<Button x:Name="btn1" Content="编辑按钮1" Visibility="Collapsed"/>
<Button x:Name="btn2" Content="编辑按钮2" Visibility="Collapsed"/>
<Button x:Name="btn3" Content="编辑按钮3" Visibility="Collapsed"/>
</StackPanel>
<DataTemplate.Triggers>
<DataTrigger Binding="{Binding Source={StaticResource ResourceKey=core},Path=Condition}" Value="条件1">
<Setter TargetName="cb1" Property="Visibility" Value="Visible" />
<Setter TargetName="btn1" Property="Visibility" Value="Visible"/>
</DataTrigger>
<DataTrigger Binding="{Binding Source={StaticResource ResourceKey=core},Path=Condition}" Value="条件2">
<Setter TargetName="tb2" Property="Visibility" Value="Visible" />
<Setter TargetName="btn1" Property="Visibility" Value="Visible"/>
<Setter TargetName="btn2" Property="Visibility" Value="Visible"/>
</DataTrigger>
<DataTrigger Binding="{Binding Source={StaticResource ResourceKey=core},Path=Condition}" Value="条件3">
<Setter TargetName="cbb3" Property="Visibility" Value="Visible" />
<Setter TargetName="btn1" Property="Visibility" Value="Visible"/>
<Setter TargetName="btn2" Property="Visibility" Value="Visible"/>
<Setter TargetName="btn3" Property="Visibility" Value="Visible"/>
</DataTrigger>
</DataTemplate.Triggers>
</DataTemplate>
</ContentControl.ContentTemplate>
</ContentControl>
</Grid>
通知类
public class ProperyChangeBase : INotifyPropertyChanged, INotifyPropertyChanging
{
public event PropertyChangedEventHandler? PropertyChanged;
public event PropertyChangingEventHandler? PropertyChanging;
public void NotifyPropertyChanged(string propertyName)
{
this.PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
public void NotifyPropertyChanging(string propertyName)
{
this.PropertyChanging?.Invoke(this, new PropertyChangingEventArgs(propertyName));
}
public void OnPropertyChanged(string propertyName)
{
NotifyPropertyChanged(propertyName);
}
public void OnPropertyChanging(string propertyName)
{
NotifyPropertyChanging(propertyName);
}
public void SetProperty([CallerMemberName] string? propertyName = null)
{
if (propertyName != null)
{
NotifyPropertyChanged(propertyName);
}
}
public void SetProperty<T>(ref T field, T newValue, [CallerMemberName] string? propertyName = null)
{
if (field != null && newValue != null)
{
SetProperty_Null(ref field, newValue, propertyName);
}
}
public void SetProperty_Null<T>(ref T field, T newValue, [CallerMemberName] string? propertyName = null)
{
if (!EqualityComparer<T>.Default.Equals(field, newValue))
{
if (string.IsNullOrEmpty(propertyName))
{
field = newValue;
return;
}
NotifyPropertyChanging(propertyName);
field = newValue;
NotifyPropertyChanged(propertyName);
}
}
public void SetProperty<T>(T oldValue, T newValue, Action<T> callback, [CallerMemberName] string? propertyName = null)
{
if (oldValue != null && newValue != null && !EqualityComparer<T>.Default.Equals(oldValue, newValue))
{
if (string.IsNullOrEmpty(propertyName))
{
callback(newValue);
return;
}
NotifyPropertyChanging(propertyName);
callback(newValue);
NotifyPropertyChanged(propertyName);
}
}
}
实体类
public class Core : ProperyChangeBase
{
public string Condition
{
get => _Condition;
set {
SetProperty(ref _Condition, value); }
}
private string _Condition = "条件1";
}
C# 代码:
private Core core1 = new Core();
public MainWindow()
{
InitializeComponent();
if (this.FindResource("core") is Core thecore)
{
core1 = thecore;
}
core1.Condition = "条件3";
}
private void Button_Click_1(object sender, RoutedEventArgs e)
{
core1.Condition = "条件1";
}
private void Button_Click_2(object sender, RoutedEventArgs e)
{
core1.Condition = "条件2";
}
private void Button_Click_3(object sender, RoutedEventArgs e)
{
core1.Condition = "条件3";
}
展现效果:
总结
不积硅步,何以至千里
扫描二维码关注公众号,回复:
16638522 查看本文章
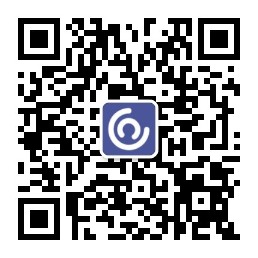