Vue3响应式API——ref
ref 是Vue3中最常用一个响应式 API,可以用来定义所有类型的数据
注意:本文写法为TypeScript,如果没有了解过需要先了解一下TypeScript
TypeScript文档阅读
类型声明
在开始使用 API 之前,要先了解一下在 TypeScript 中,ref 需要如何进行类型声明 声明方式:使用 <> 来包裹类型定义,紧跟在 ref API 之后:
//基本数据类型
const name=ref<number>(1)
//引用数据类型
const foods1=ref<Array<string>>(['苹果','香蕉'])
变量定义
/*
*基本类型
*/
字符串
const str = ref<string>('前端');
数值
const nums= ref<number>(1);
布尔值
const isbool= ref<boolean>(true);
......
/*
*引用类型
*/
数组
const ids=ref<Array<number>>([1,2,3])
对象数组
1.声明对象的格式
interface Person{
id: number,
name: string
};
- 定义一个成员组
const Students= ref<Person[]>([
{
id: 1,
name: 'Tom'
},
{
id: 2,
name: 'Jack'
}
]);
变量读取和赋值
被 ref 包裹的变量会全部变成对象,不管你定义的是什么类型的值,都会转化为一个 ref 对象,其中 ref 对象具有指向内部值的单个 property .value。
注意:
- 读取任何 ref 对象的值都必须通过 xxx.value
- 普通变量使用 let 才可以修改值, ref 对象是个引用类型,可以在 const 定义的时候,直接通过 .value 来修改
- 在数据修改时,可以使用 forEach、map、filter 等遍历函数来操作你的 ref 数组,或者直接重置
// 1.读取一个字符串没有使用ref
const msg: string = 'Hello World!';
console.log('msg的值', msg);
// 2.读取一个字符串使用ref
const str = ref<string>('Hello World!');
console.log('str的值', str.value);
// 3.读取一个数组使用ref
const ids = ref<number[]>([ 1, 2, 3 ]);
console.log('第二个id', ids.value[1]);
// 4.修改数据
const data = ref<string[]>([]);
data.value = api.data.map( (item: any) => item.text );
Vue3响应式API——reactive
reactive 是继 ref 之后最常用的一个响应式 API 了,相对于 ref,它的局限性在于只适合对象、数组
类型声明和定义
reactive 对象:
// 声明对象的格式
interface Member {
id: number,
name: string
};
// 定义一个成员对象
const userInfo: Member = reactive({
id: 1,
name: 'Tom'
});
reactive 数组:
// 普通数组
const uids: number[] = [ 1, 2, 3];
// 对象数组
interface Member {
id: number,
name: string
};
// 定义一个成员对象数组
const userList: Member[] = reactive([
{
id: 1,
name: 'Tom'
},
{
id: 2,
name: 'Petter'
},
{
id: 3,
name: 'Andy'
}
]);
变量的读取与赋值
reactive 对象在读取字段的值,或者修改值的时候,与普通对象是一样的
// 声明对象的格式
interface Member {
id: number,
name: string
};
// 定义一个成员对象
const userInfo: Member = reactive({
id: 1,
name: 'Tom'
});
// 读取用户名
console.log(userInfo.name);
// 修改用户名
userInfo.name = 'Petter';
对于 reactive 数组,和普通数组会有一些区别:
- 在 vue3中,如果你使用 reactive 定义数组,必须只使用那些不会改变引用地址的操作
- 不要对通过 reactive 定义的对象进行解构,解构后得到的变量会失去响应性。
失去响应式:
let uids: number[] = reactive([ 1, 2, 3 ]);
// 丢失响应性的步骤
uids = [];
// 异步获取数据后,模板依然是空数组
setTimeout( () => {
uids.push(1);
}, 1000);
正确方式:
let uids: number[] = reactive([ 1, 2, 3 ]);
// 不会破坏响应性
uids.length = 0;
// 异步获取数据后,模板可以正确的展示
setTimeout( () => {
uids.push(1);
}, 1000);
应用场景
ref
- 当数值类型是 JS 原始类型时使用 (如:string、number)
- 当赋值对象,且后续需要被重新赋值时 (例如一个数组)
reactive
扫描二维码关注公众号,回复:
16490018 查看本文章
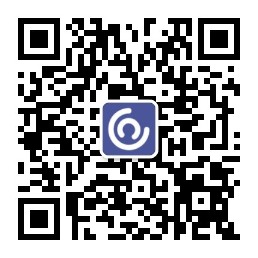
- 当数值类型是对象,且不需要重新赋值时使用,ref() 内部也是调用 reactive()
参考文献:链接地址