目录
一、什么是less
~~~~~~ Less(Leaner Style Sheets 精简样式表) 是一种动态样式语言,属于 css 预处理器的范畴,它扩展了 CSS 语言,增加了变量、Mixin、函数等特性,使 CSS 更易维护和扩展,Less 既可以在 客户端 上运行 ,也可以借助 Node.js 在服务端运行。
Less 编译工具:
- Koala(opens new window)
- vscode 的 Easy LESS 插件
二、基本使用
2.1 基本语法
- 多行注释保留
- 单行注释不被保留在编译生成的 CSS 中
- @ 声明变量,作为普通属性值使用
/*
多行注释保留
*/
// 单行注释不被保留在编译生成的 CSS 中
@width:100px;
.outer{
width: @width+100px;
height: @width+100px;
border: 1px solid red;
.inner{
margin: 0 auto;
margin-top: 50px;
width: 50px;
height: 50px;
background-color: orange;
}
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" type="text/css" href="../css/01.css"/>
</head>
<body>
<div class="outer">
<div class="inner"></div>
</div>
</body>
</html>
2.2 变量插值
- 变量用于选择器名、属性名、URL、@import语句
/*
变量用于选择器名、属性名、URL、@import语句
*/
@selector:outer;
// 需要添加 {
}
.@{
selector}{
height: 200px;
width: 200px;
background-color: orange;
}
@property: color;
.widget {
@{
property}: #0ee;
background-@{
property}: #999;
}
@images: '../img';
// Usage
body {
color: #444;
background: url('@{images}/white-sand.png');
}
@themes: '../../src/themes';
// Usage
@import '@{
themes}/tidal-wave.less';
2.3 延迟加载
当一个变量被声明多次,会取最后一次的值,并从当前作用域往外寻找变量。
//当一个变量被声明多次,会取最后一次的值,并从当前作用域往外寻找变量。
@var:0;
.outer{
@var:1px;
.inner{
@var:2px;
height: 200px;
width: 200px;
background-color: orange;
border: @var solid black;
@var:3px;
}
width: 300 * @var;
height: 300 * @var;
}
.outer {
width: 300px;
height: 300px;
}
.outer .inner {
height: 200px;
width: 200px;
background-color: orange;
border: 3px solid black;
}
2.4 属性名变量
您可以很容易地将属性看作是使用$prop语法的变量
// 您可以很容易地将属性看作是使用$prop语法的变量
.outer{
width: 200px;
height: @width;
border: 1px solid black;
}
2.5 嵌套
Less 提供了使用嵌套(nesting)代替层叠或与层叠结合使用的能力
//Less 提供了使用嵌套(nesting)代替层叠或与层叠结合使用的能力
.outer{
width: 200px;
height: 200px;
border: 1px solid black;
@width:50px;
@height:50px;
.inner1{
width: @width;
height: @height;
background-color: orange;
}
.inner2{
width: @width;
height: @height;
background-color: red;
}
}
2.6 父选择器
在嵌套规则中, & 表示父选择器,常用于给现有选择器添加伪类。
//在嵌套规则中, &表示父选择器,常用于给现有选择器添加伪类。
// 如果没有 &,会被编译成后代选择器 .inner1 :hover。
.outer{
width: 200px;
height: 200px;
border: 1px solid black;
margin: 0 auto;
position: relative;
.inner1{
width: 100px;
height: 100px;
background-color: orange;
transition: 0.2s linear;
position: absolute;
top: 50px;
left: 50px;
&:hover{
box-shadow: 0 0 20px black;
}
}
}
如果没有 &,会被编译成后代选择器 a :hover。
除此之外,& 还能用于生成重复类名:
//除此之外,& 还能用于生成重复类名:
.box{
&-demo{
width: 200px;
height: 200px;
background-color: red;
}
}
三、混合
3.1 普通混合
混合(Mixin)是一种将一组属性从一个规则集包含(或混入)到另一个规则集的方式,可理解为复制粘贴
//混合(Mixin)是一种将一组属性从一个规则集包含(或混入)到另一个规则集的方式,可理解为复制粘贴。
.test{
width: 100px;
height: 100px;
background-color: orange;
}
.outer{
width: 200px;
height: 200px;
border: 1px solid black;
.inner1{
.test()
}
}
.test {
width: 100px;
height: 100px;
background-color: orange;
}
.outer {
width: 200px;
height: 200px;
border: 1px solid black;
}
.outer .inner1 {
width: 100px;
height: 100px;
background-color: orange;
}
3.2 带参数的混合
- 混合带参数,参数需要按顺序传递
//1.混合带参数,参数需要按顺序传递 .border1(@width,@style,@color){ border: @width @style @color; } .outer{ width: 200px; height: 200px; .border1(1px,solid,black); }
.outer { width: 200px; height: 200px; border: 1px solid black; }
- 混合带参数并有默认值
需注意的是,就算有默认值,也要按顺序传递
//2.混合带参数并有默认值
.border2(@width:2px,@style,@color:red){
border: @width @style @color;
}
.outer{
.inner1{
width: 100px;
height: 100px;
.border2(1px,solid);
}
}
3.3 命名参数
可以在向混合传参是指定参数名称,从而不需要按顺序传入
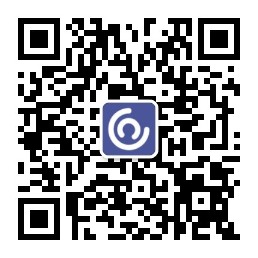
//可以在向混合传参时指定参数名称,从而不需要按顺序传入
.border1(@width:2px,@style,@color){
border: @width @style @color;
}
.outer{
width: 200px;
height: 200px;
.border1(@style:solid,@color:black);
}
3.4 @arguments 变量
@arguments
变量包含了传入的所有参数
//@arguments 变量包含了传入的所有参数
.box-shadow(@x: 0, @y: 0, @blur:20px, @color: #000){
box-shadow: @arguments;
}
.outer{
height: 200px;
width: 200px;
border: 1px solid black;
.box-shadow(0,0,@color:red);
}
3.5 匹配模式
在多个相同的混合中(参数个数必须相同),匹配特定的混合。
.mixin(dark, @color) {
color: darken(@color, 10%);
}
.mixin(light, @color) {
color: lighten(@color, 10%);
}
// @_ 表示匹配所有
.mixin(@_, @color) {
display: block;
}
@switch: light;
.class {
.mixin(@switch, #888);
}
.class {
color: #a2a2a2;
display: block;
}
四、运算
计算结果以操作数最左侧的单位类型为准
//计算结果以操作数最左侧的单位类型为准
@conversion-1: 5cm + 10mm; // 6cm
@conversion-2: 2 - 3cm - 5mm; // -1.5cm
@conversion-3: 3.1 * 2cm; // 6.2cm
@conversion-4: 4px / 2; // 4px / 2
// conversion is impossible
@incompatible-units: 1cm - 1px; // 0.97354167cm
// example with variables
@base: 5%;
@filler: @base * 2; // 10%
@other: @base + @filler; // 15%
@color: #224488 / 2; // #112244
body{
background-color: #112244 + #111; // #223355
}
五、继承
5.1 继承格式
继承可让多个选择器应用同一组属性,最终编译为并集选择器
其性能比混合高,但灵活性比混合低
/*
继承可让多个选择器应用同一组属性,最终编译为并集选择器
其性能比混合高,但灵活性比混合低
*/
.inner1{
&:extend(.inner);
background-color: orange;
}
.inner{
width: 50px;
height: 50px;
}
.inner1 {
background-color: orange;
}
.inner,
.inner1 {
width: 50px;
height: 50px;
}
5.2 继承all
可理解为把 all 前的选择器出现的地方全都替换为 extend 前的选择器
即把 .test 替换为 .replacement 生成一个新的选择器应用样式,且不破坏原有的选择器
/*
可理解为把 all 前的选择器出现的地方全都替换为 extend 前的选择器
即把 .test 替换为 .replacement 生成一个新的选择器应用样式,且不破坏原有的选择器
*/
.box.inner1{
width: 200px;
height: 200px;
background-color: orange;
}
.inner2:extend(.inner1 all){
}
.box.inner1,
.box.inner2 {
width: 200px;
height: 200px;
background-color: orange;
}
六、避免编译
通过加引号可以避免 Less 编译,直接把内容输出到 CSS 中
//通过加引号可以避免 Less 编译,直接把内容输出到 CSS 中
.outer{
width: '200px + 100px';
height: 200px;
background-color: orange;
}
.outer {
width: '200px + 100px';
height: 200px;
background-color: orange;
}
七、命名空间和访问符
有时,出于组织结构或仅仅是为了提供一些封装的目的,你希望对混合(mixins)进行分组。你可以用 Less 更直观地实现这一需求。假设你希望将一些混合(mixins)和变量置于 #bundle 之下,为了以后方便重用或分发:
#bundle() {
.button {
display: block;
border: 1px solid black;
background-color: grey;
&:hover {
background-color: white;
}
}
.tab {
... }
.citation {
... }
}
现在,如果我们希望把 .button 类混合到 #header a 中,我们可以这样做:
#header a {
color: orange;
#bundle.button(); // 还可以书写为 #bundle > .button 形式
}
八、映射
从 Less 3.5 版本开始,你还可以将混合(mixins)和规则集(rulesets)作为一组值的映射(map)使用。
#colors() {
primary: blue;
secondary: green;
}
.button {
color: #colors[primary];
border: 1px solid #colors[secondary];
}
.button {
color: blue;
border: 1px solid green;
}