目录
一、什么是thymeleaf?
thymeleaf是一个基于Java的模板引擎,它可以用来处理XML/XHTML/HTML5等格式的文件,将数据和文本渲染到模板中,生成动态的页面。它与JSP,Velocity,FreeMarker等模板引擎类似,也可以轻易地与Spring Boot等Web框架集成。SpringBoot官方并不推荐使用JSP,而是使用thymeleaf。
thymeleaf的主要目标是提供一种优雅和自然的模板语法,让模板文件既可以在浏览器中正确显示,也可以作为静态原型,方便开发团队之间的协作1。它还支持多种模式,如XML,XHTML,HTML5,Legacy HTML5等,可以处理任何XML文件或非XML文件。
thymeleaf的基本语法如下:
- 使用
th:
前缀来标识thymeleaf的属性或标签,如<div th:text="${name}"></div>
表示将name变量的值渲染到div标签中。 - 使用
${}
来表示变量表达式,如${name}
表示name变量的值。 - 使用
#
来表示工具对象表达式,如${#numbers.formatDecimal(price, 1, 2)}
表示使用numbers工具对象来格式化price变量的值为两位小数。 - 使用
@
来表示链接表达式,如<a th:href="@{/product/{id}(id=${prod.id})}">View</a>
表示使用prod.id变量的值来构造一个相对于当前上下文路径的链接。 - 使用
*
来表示选择表达式,如<input type="text" th:field="*{name}"/>
表示将name属性绑定到input标签中。 - 使用
_
来表示文字替换表达式,如<p th:text="_(${session.user.name} + ' is ' + ${session.user.age} + ' years old')"></p>
表示将括号内的字符串替换为变量值后渲染到p标签中。
例如:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Thymeleaf Demo</title>
</head>
<body>
<h1 th:text="${title}">Thymeleaf Demo</h1>
<p th:if="${message}" th:text="${message}">Welcome!</p>
<ul>
<li th:each="item : ${items}" th:text="${item.name}">Item</li>
</ul>
<form th:action="@{/submit}" th:object="${form}" method="post">
<input type="text" th:field="*{name}" placeholder="Name"/>
<input type="email" th:field="*{email}" placeholder="Email"/>
<button type="submit">Submit</button>
</form>
</body>
</html>
二、如何在Spring Boot中使用thymeleaf?
在Spring Boot中使用thymeleaf非常简单,只需要以下几个步骤:
1、在Maven或Gradle项目中添加thymeleaf相关的依赖包。
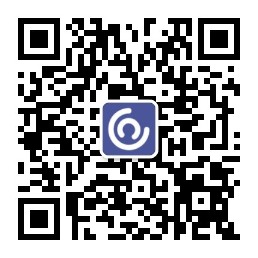
<!-- Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- Gradle -->
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
2、在resources目录下创建一个名为templates的子目录,并在其中放置thymeleaf模板文件。
<!-- templates/index.html -->
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Thymeleaf Demo</title>
</head>
<body>
<h1 th:text="${title}">Thymeleaf Demo</h1>
<p th:if="${message}" th:text="${message}">Welcome!</p>
<ul>
<li th:each="item : ${items}" th:text="${item.name}">Item</li>
</ul>
<form th:action="@{/submit}" th:object="${form}" method="post">
<input type="text" th:field="*{name}" placeholder="Name"/>
<input type="email" th:field="*{email}" placeholder="Email"/>
<button type="submit">Submit</button>
</form>
</body>
</html>
3、在Java代码中创建一个控制器类,并使用@Controller注解来标识,并使用@RequestMapping或@GetMapping等注解来映射请求路径和方法,并使用Model或ModelAndView等对象来传递数据到模板中。
// DemoController.java 控制器类
@Controller
public class DemoController {
// 处理根路径的GET请求,返回index模板
@GetMapping("/")
public String index(Model model) {
// 设置模型属性
model.addAttribute("title", "Thymeleaf Demo");
model.addAttribute("message", "Welcome!");
model.addAttribute("items", Arrays.asList(
new Item("Apple", 1.99),
new Item("Banana", 0.99),
new Item("Orange", 1.49)
));
model.addAttribute("form", new Form());
// 返回模板名称,不需要后缀
return "index";
}
// 处理/submit路径的POST请求,接收表单数据并重定向到/result模板
@PostMapping("/submit")
public ModelAndView submit(@ModelAttribute Form form) {
// 创建ModelAndView对象,设置视图名称和模型属性
ModelAndView mav = new ModelAndView();
mav.setViewName("result");
mav.addObject("title", "Thymeleaf Demo");
mav.addObject("message", "You have submitted the form successfully!");
mav.addObject("form", form);
// 返回ModelAndView对象
return mav;
}
}
4、启动Spring Boot应用程序,并在浏览器中访问http://localhost:8080/,查看渲染后的页面。
三、thymeleaf的优势和局限性
thymeleaf是一种优秀的模板引擎,它有以下优点:
- 它是基于标准的,可以让你创建符合XML和Web标准的模板,如果你需要的话。
- 它是自然的,可以让你的模板文件在浏览器中直接显示,也可以作为静态原型,方便设计和开发。
- 它是灵活的,支持多种模式,多种数据类型,多种表达式,也支持自定义方言和处理器,可以扩展和增强框架的功能。
- 它是集成的,可以与Spring Boot等Web框架无缝集成,也可以与Eclipse,IntelliJ IDEA等开发工具配合使用。
当然,thymeleaf也有一些局限性,如:
- 它是基于XML的,可能会让一些习惯了HTML的开发者感到不太舒服。
- 它是基于服务器端的,可能会增加服务器的负担和响应时间。
- 它是基于字符串的,可能会导致一些转义或编码的问题。
四、总结
本文介绍了thymeleaf模板引擎的概念、特点和使用方法,并分析了它的优势和局限性。我们可以看出,thymeleaf是一种适合于现代HTML5 JVM Web开发的模板引擎,它可以让我们更容易地创建优雅和自然的模板。当然,在使用thymeleaf时,我们也需要注意一些语法规则和性能问题。