1.流的简介与操作流程:
2.文件的读取与输出:
1).文件的读取:
package byteIO; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; /** * * 文件的读取 * 1、建立联系 File对象 * 2、选择流 文件输入流 InputStream FileInputStream * 3、操作 : byte[] buffer =new byte[1024]; +read+读取大小 * 输出 * 4、释放资源 : 关闭 * * @author Wang * */ public class InputStream { public static void main(String[] args) { File file = new File("F:/testIO/Demo03.java");//建立联系 FileInputStream input = null; //选择流 提升作用域 try { input = new FileInputStream(file); byte[] buffer = new byte[1024]; //设置每次读取1024个字节 这里相当于设置一个固定大小的缓存器 每次读取的时候都是通过他读取 int len = 0; //用一个中间变量看看实际每次读取了多少个字节 StringBuilder sb = new StringBuilder();//把读取出来的文件全部转化为String类型存放在这数组里面 while((len = input.read(buffer))!=-1) { //循环读取 read这个方法等读取完毕的时候他会返回一个-1 String str =new String(buffer,0,len); //把Byte类型的转化为 String sb.append(str); //将指定的字符串附加到此字符序列。 把每次读取出来的String放进这个数组里面 } System.out.println(sb); //输出读取的文件 } catch (FileNotFoundException e) { e.printStackTrace(); System.out.println("文件不存在"); } catch (IOException e) { e.printStackTrace(); System.out.println("文件读取失败"); } finally { if(input != null) { //如果input这个引用不是null 就说明这个文件还没被关闭 那么我们就关闭他 try { input.close(); } catch (IOException e) { e.printStackTrace(); System.out.println("文件关闭失败"); } } } } }
2)文件的写入:
package byteIO; import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; /** * * 文件的写入 * 写出文件 * 1、建立联系 File对象 目的地 * 2、选择流 文件输出流 OutputStream FileOutputStream * 3、操作 : write() +flush * 4、释放资源 :关闭 * * @author Wang * */ public class OutputStream { public static void main(String[] args) { File file = new File("F:/testIO/1.txt"); FileOutputStream os = null; try { os = new FileOutputStream(file,true); //后面的这个参数如果为true的话那么就是在文件的后面追加 如果为false那么就是覆盖 String str = "我的老家就住在这个地方好 i like it very munch."; byte[] buffer = str.getBytes(); // 把你需要写入的这个字符串转化为Byte[] 的格式 os.write(buffer,0,buffer.length); //将buffer中的数据从0写buffer.length长度的数据 os.flush(); //刷新缓存区 强制把缓存区的数据写到文件中 } catch (FileNotFoundException e) { e.printStackTrace(); System.out.println("没有找到指定文件"); } catch (IOException e) { e.printStackTrace(); System.out.println("文件写入失败"); } finally { if(os != null) { try { os.close(); } catch (IOException e) { e.printStackTrace(); } } } } }
3.文件的拷贝:(就是读取和写入的综合)
package byteIO; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; /** * * 写一个文件的copy代码 我们在这里copy一个图片文件 * * @author Wang * */ public class CopyFile { public static void main(String[] args) throws IOException { File file1 = new File("F:/testIO/java.png"); File file2 = new File("F:/testIO/javacopy.png"); FileInputStream is = null; is = new FileInputStream(file1); //这里我们把异常抛出去这样的话便于我们阅读 FileOutputStream os = new FileOutputStream(file2); byte[] buffer = new byte[1024]; int length = 0; while((length = is.read(buffer)) != -1) {//抛异常 os.write(buffer,0,length); } os.flush(); //强制刷出 os.close(); is.close(); //先开后关 } }
4.文件夹的拷贝
扫描二维码关注公众号,回复:
1628216 查看本文章
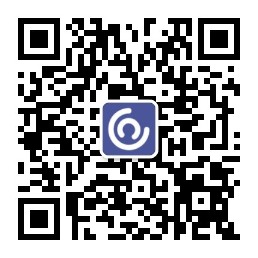
package byteIO; import java.io.File; import java.io.IOException; /** * * 文件夹的拷贝 * 检索子目录的时候我们还是用递归 * 如果是文件夹就创建 如果是文件就Copy * * @author Wang * */ public class copyDir { public static void main(String[] args) { /* File src = new File("F:/testIO/a"); //调用第一个方法 File target = new File("F:/testIO/e"); try { copyDirDetail(src,target); } catch (IOException e) { System.out.println("拷贝文件夹失败"); e.printStackTrace(); }*/ /*try { //调用第二个方法 copyDirDetail("F:/testIO/a","F:/testIO/d"); } catch (IOException e) { e.printStackTrace(); }*/ try { copyUtil.copyDirDetail("F:/testIO/a","F:/testIO/d"); } catch (IOException e) { e.printStackTrace(); } } public static void copyDirDetail(File src,File target) throws IOException { if(src.isFile()) { target = new File(target,src.getName());//这一句很重要 请仔细体会一下 就是将src文件的地址给target 每次递归深一层就要改变一下 copyUtil.copyFile(src,target); }else{ target = new File(target,src.getName());//这一句很重要 请仔细体会一下 就是将src文件的地址给target 每次递归深一层就要改变一下 target.mkdirs(); //按照目标文件夹的地址创建一个文件夹 保证目标文件夹存在 for(File temp : src.listFiles()) { copyDirDetail(temp,target); } } } public static void copyDirDetail(String srcPath,String targetPath) throws IOException { File src = new File(srcPath); File target = new File(targetPath); copyDirDetail(src,target); } }
5.我们把copy方法包装成一个类
package byteIO; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; /** * * 我们来把文件和文件夹的拷贝封装一下 * * @author Wang * */ public class copyUtil { public static void main(String[] args) { /*File file1 = new File("F:/testIO/Demo03.java"); File file2 = new File("F:/testIO/Demo03Copy.java"); try { copyFile(file1,file2); } catch (IOException e) { System.out.println("需要复制的文件不存在或者是文件打开失败"); e.printStackTrace(); }*/ try { copyFile("F:/testIO/1.txt","F:/testIO/3.txt"); } catch (IOException e) { e.printStackTrace(); System.out.println("需要复制的文件不存在或者是文件打开失败"); } } public static void copyFile(String str1,String str2) throws IOException { copyFile(new File(str1),new File(str2)); } public static void copyFile(File file1,File file2) throws IOException { if(! file1.isFile()){ //不是文件或者为null System.out.println("只能拷贝文件"); throw new IOException("只能拷贝文件"); } if(file2.isDirectory()){ System.out.println(file2.getAbsolutePath()+"不能建立于文件夹同名的文件"); throw new IOException(file2.getAbsolutePath()+"不能建立于文件夹同名的文件"); } FileInputStream is = new FileInputStream(file1); //这里我们把异常抛出去这样的话便于我们阅读 FileOutputStream os = new FileOutputStream(file2); byte[] buffer = new byte[1024]; int length = 0; while((length = is.read(buffer)) != -1) {//抛异常 os.write(buffer,0,length); } os.flush(); //强制刷出 os.close(); is.close(); //先开后关 } public static void copyDirDetail(File src,File target) throws IOException { if(src.isFile()) { target = new File(target,src.getName());//这一句很重要 请仔细体会一下 就是将src文件的地址给target 每次递归深一层就要改变一下 copyUtil.copyFile(src,target); }else{ target = new File(target,src.getName());//这一句很重要 请仔细体会一下 就是将src文件的地址给target 每次递归深一层就要改变一下 target.mkdirs(); //按照目标文件夹的地址创建一个文件夹 保证目标文件夹存在 for(File temp : src.listFiles()) { copyDirDetail(temp,target); } } } public static void copyDirDetail(String srcPath,String targetPath) throws IOException { File src = new File(srcPath); File target = new File(targetPath); copyDirDetail(src,target); } }
6.字符流的读取
package cahrIO; import java.io.File; import java.io.FileReader; import java.io.IOException; import java.io.Reader; /** * * 字符流的读取 * * @author Wang * */ public class reader { public static void main(String[] args) { File src = new File("F:/testIO/Demo03.java"); Reader reader = null; try { reader = new FileReader(src); char[] buffer = new char[1000]; //这次是每次读取1000个字符了 int length = 0; StringBuilder sb = new StringBuilder(); while((length = reader.read(buffer)) != -1) { String str = new String(buffer,0,length); sb.append(str); } System.out.println(sb); } catch (IOException e) { e.printStackTrace(); System.out.println("文件读取失败"); }finally { if(reader != null) { try { reader.close(); } catch (IOException e) { e.printStackTrace(); System.out.println("文件关闭失败"); } } } } }
7.字符流的写入;
package cahrIO; import java.io.File; import java.io.FileWriter; import java.io.IOException; import java.io.Writer; /** * * 字符流的输出 * * @author Wang * */ public class writer { public static void main(String[] args) { File target = new File("F:/testIO/3.txt"); Writer writer = null; try { writer = new FileWriter(target,true); //后面加一个true 实在文件后面追加 String str = new String("锄禾日当午,码农真辛苦\r\n码农真辛苦"); writer.write(str);//这个不用缓冲数组进行转换 //writer.append("倒萨发了看电视剧 "); //这个也是在文件后面追加 writer.flush(); //强制刷出 } catch (IOException e) { e.printStackTrace(); System.out.println("文件打开失败"); } finally { if(writer != null) { try { writer.close(); } catch (IOException e) { e.printStackTrace(); System.out.println("文件关闭失败"); } } } } }
8.字符流的copy
package cahrIO; import java.io.File; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import java.io.Reader; import java.io.Writer; /** * * 字符流的copy * * @author Wang * */ public class copy { public static void main(String[] args) { File src = new File("F:/testIO/Demo03.java"); File target = new File("F:/testIO/Demo04.java"); Reader reader = null; Writer writer = null; try { reader = new FileReader(src); writer = new FileWriter(target); char[] buffer = new char[1000]; //这次是每次读取1000个字符了 int length = 0; while((length = reader.read(buffer)) != -1) { writer.write(buffer); } } catch (IOException e) { e.printStackTrace(); System.out.println("文件读取失败"); }finally { if(reader != null) { try { reader.close(); //先开相关 writer.close(); } catch (IOException e) { e.printStackTrace(); System.out.println("文件关闭失败"); } } } } }
注意:
文件的写入当文件不存在的时候,他会自己建立这个文件;
字节流什么文件都可以处理;
字符流只能处理纯文本;
每次读取的时候会把数据线都到buffer[] 这个数组里面,相当于缓冲了;
字节流与字符流的读取和写入这些操作基本一致就是用的方法不同而已;
/r/n 前者代表将光标放到行首,\n就是换行;
在写入的时候需要强制刷出 别忘强制刷出 fluse();