一、Vue2
1. 父传子
props
<!-- 父组件 -->
<template>
<child-component title="这是标题" content="这是内容,可能有各种类型" />
</template>
// 子组件
export default {
props: {
marjorCollegeName: {
type: String,
default: ''
},
content: {
type: [Array, String], // 把可能的类型都列出来
default: () => []
},
}
}
事件传值
<!-- 父组件 -->
<template>
<child-component ref="childcomponent" />
</template>
<script>
export default {
methods: {
this.$ref.childcomponent.changeData()
}
}
</script>
// 子组件
export default {
methods: {
changeData() {
}
}
}
vuex
vuex
的值是实时同步的,所有组件都可以访问到,由于内容过多,本文不做详细介绍,附另一篇详细介绍 vuex
使用方法的博客链接。
vuex使用方法:https://blog.csdn.net/m0_53808238/article/details/117091249
2. 子传父
事件传值
// 子组件
this.$emit('changeValue', 100)
// 父组件
export default {
methods: {
changeValue(value) {
console.log(value)
}
}
}
vuex
同上,
vuex使用方法:https://blog.csdn.net/m0_53808238/article/details/117091249
3. 兄弟组件互传
路由传参
this.$router.push({
path: "/detail",
query: {
id: 12345
},
})
export default {
computed: {
id() {
return this.$route.query.id;
},
},
}
浏览器缓存
使用 sessionStorage
或者 localStorage
进行存储/读取。
// 存储
sessionStorage.setItem("id", 12345);
localStorage.setItem("id", 12345);
// 读取
sessionStorage.getItem("id");
localStorage.getItem("id");
如果是对象类型,需要使用 JSON
进行转换。
JSON.stringify() 、JSON.parse()
vuex
同上,
vuex使用方法:https://blog.csdn.net/m0_53808238/article/details/117091249
二、Vue3
1. 父传子
props
<!-- 输入 -->
<template>
<child-component id="12345" />
</template>
ts
的写法接收时需要断言类型,如果不确定是否使用需要加一个 ?
// 接收
<script setup lang='ts'>
const props = defineProps<{
id?: string,
}>()
</script>
Pinia
Pinia 使用方法内容有点多,本文限于篇幅,不做介绍,附一篇详细介绍使用方法的博客链接。
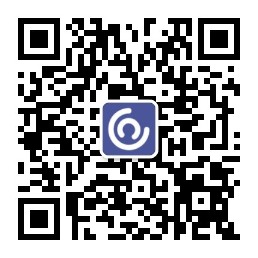
Pinia 使用方法:https://juejin.cn/post/7213994684262891576
依赖注入
依赖注入不仅可以父子传值,也可以传递给更深层次的子组件。如果提供的值是响应式数据,那么注入的值也是响应式的。所谓注入就是读取的意思。
// 父组件-提供
import {
provide } from 'vue'
provide('id', 12345)
// 后代组件-注入
import {
inject } from 'vue'
// 注入时需提供一个默认值
const id = inject<string>('id', '')
事件传值
<!-- 父组件 -->
<script setup lang='ts'>
import {
ref } from 'vue'
const childComponent = ref(null)
childComponent.value.getList()
</script>
<template>
<child-component ref="childComponent"></child-component>
</template>
// 子组件
const getList = () => {
console.log(12345)
}
2. 子传父
事件传值
<!-- 父组件 -->
<child-component @onSearch="getList"></child-component>
// 子组件
const emit = defineEmits(['onSearch'])
const onSearch = () => {
emit('onSearch', 123456)
}
Pinia
同上。
Pinia 使用方法:https://juejin.cn/post/7213994684262891576
3. 兄弟组件互传
路由传参
// 传递
import {
useRouter } from 'vue-router'
const router = useRouter()
router.push({
path: '/detail',
query: {
id: 12345
}
})
// 接收
import {
useRoute, computed } from 'vue-router'
const route = useRoute()
const id = computed(() => {
return route.query.id
})
浏览器缓存
使用 sessionStorage
或者 localStorage
进行存储/读取。
// 存储
sessionStorage.setItem("id", 12345);
localStorage.setItem("id", 12345);
// 读取
sessionStorage.getItem("id");
localStorage.getItem("id");
如果是对象类型,需要使用 JSON
进行转换。
JSON.stringify() 、JSON.parse()
Pinia
同上。
Pinia 使用方法:https://juejin.cn/post/7213994684262891576
END