1、简述
在现实世界中可能会出现服务器被虚假请求轰炸的情况,因此您可能希望控制这种虚假的请求。
一些实际使用情形可能如下所示:
-
API配额管理-作为提供者,您可能希望根据用户的付款情况限制向服务器发出API请求的速率。这可以在客户端或服务端实现。
-
安全性-防止DDOS攻击。
-
成本控制–这对服务方甚至客户方来说都不是必需的。如果某个组件以非常高的速率发出一个事件,它可能有助于控制它,它可能有助于控制从客户端发送的请求。
常见的限流算法:令牌桶算法, 漏桶算法。比较成熟的有分布式hystrix, sentinel,还有guava高并发限流ratelimiter。本文主要是介绍Redis如何对指定的key进行计数限流的。
2、引用和配置
引用redis的maven包,包括客户端连接插件jedis。
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
</dependency>
在application.yml配置中添加Redis服务端配置:
spring:
#redis配置#
redis:
database: 0
host: 192.168.254.128
port: 6379
password: 123456
timeout: 5000
jedis:
pool:
max-active: 8
max-wait: 5000
max-idle: 8
min-idle: 1
3、Config配置
添加redis configuration配置bean和redis存储json转换。
@Configuration
public class MyRedisConfig {
@Value("${spring.redis.host}")
private String host;
@Value("${spring.redis.password}")
private String password;
@Value("${spring.redis.port}")
private int port;
@Value("${spring.redis.database}")
private int database;
//@SuppressWarnings("all")
@Bean
public StringRedisTemplate redisTemplate(RedisConnectionFactory factory){
StringRedisTemplate template = new StringRedisTemplate(factory);
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jackson2JsonRedisSerializer.setObjectMapper(om);
RedisSerializer stringSerializer = new StringRedisSerializer();
template.setKeySerializer(stringSerializer);
template.setValueSerializer(jackson2JsonRedisSerializer);
template.setHashKeySerializer(stringSerializer);
template.setHashValueSerializer(jackson2JsonRedisSerializer);
template.afterPropertiesSet();
return template;
}
@Bean
public JedisConnectionFactory jedisConnectionFactory() {
RedisStandaloneConfiguration configuration = new RedisStandaloneConfiguration();
configuration.setHostName(host);
configuration.setPassword(RedisPassword.of(password));
configuration.setPort(port);
configuration.setDatabase(database);
JedisConnectionFactory jedisConnectionFactory = new JedisConnectionFactory(configuration);
jedisConnectionFactory.getPoolConfig().setMaxIdle(30);
jedisConnectionFactory.getPoolConfig().setMinIdle(10);
return jedisConnectionFactory;
}
}
4、添加组件
添加自定义的限速拦截器组件,到时候拦截器会根据该组件注释来拦截对应的接口请求,实现跟业务解耦。
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface RequestLimit {
/**
* 调用方唯一key的名字
*
* @return
*/
String name();
/**
* 限制访问次数
* @return
*/
int limitTimes();
/**
* 限制时长,也就是计数器的过期时间
*
* @return
*/
long timeout();
/**
* 限制时长单位
*
* @return
*/
TimeUnit timeUnit();
}
- name :表示请求方唯一的身份参数,如userId,token等。
- limitTimes :表示限制访问次数,也就是他在指定时间内可以访问多少次。
- timeout:表示限制访问次数的有效期,一分钟还是一个小时。
- timeUnit:表示限速实际的单位,秒、分钟、小时等
5、拦截器
添加拦截器实现HandlerInterceptor 来实现对添加@RequestLimit 进行拦截处理当前请求在规定的时间是否超出。当前采用的是redis的BoundValueOperations 来计算请求次数在规定的时间内是否异常。
/**
* 请求限流拦截器
*/
@Slf4j
@Component
public class RequestLimitInterceptor implements HandlerInterceptor {
@Autowired
private RedisTemplate redisTemplate;
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
if(handler instanceof HandlerMethod){
HandlerMethod handlerMethod = (HandlerMethod) handler;
//判断接口是否添加requestLimit
if(handlerMethod.hasMethodAnnotation(RequestLimit.class)){
RequestLimit requestLimit = handlerMethod.getMethod().getAnnotation(RequestLimit.class);
JSONObject object = new JSONObject();
String token = request.getParameter(requestLimit.name());
response.setContentType("text/json;charset=utf-8");
object.put("timestamp", System.currentTimeMillis());
BoundValueOperations<String, Integer> boundValueOperations = redisTemplate.boundValueOps(token);
if(StringUtils.isEmpty(token)){
object.put("result", "token is invalid");
response.getWriter().print(JSON.toJSONString(object));
} else if(checkLimit(token,requestLimit)){
object.put("result","token is success,请求成功");
long expire = boundValueOperations.getExpire();
return true;
}else {
object.put("result", "达到访问次数上限,禁止访问!");
response.getWriter().print(JSON.toJSONString(object));
}
return false;
}
}
return true;
}
/**
* 限速校验
* @param token
* @param limit
* @return
*/
private Boolean checkLimit(String token, RequestLimit limit){
BoundValueOperations<String , Integer> boundValueOperations = redisTemplate.boundValueOps(token);
Integer count = boundValueOperations.get();
if(Objects.isNull(count)){
redisTemplate.boundValueOps(token).set(1,limit.timeout(), limit.timeUnit());
}else if(count > limit.limitTimes()){
return Boolean.FALSE;
}else {
redisTemplate.boundValueOps(token).set(++count, boundValueOperations.getExpire(),limit.timeUnit());
}
return Boolean.TRUE;
}
}
实现拦截器后我们需要将当前拦截器添加到WebMvcConfigurer拦截器中:
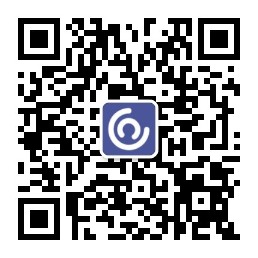
@Configuration
public class MyWebConfig implements WebMvcConfigurer {
@Autowired
private RequestLimitInterceptor requestLimitInterceptor;
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(requestLimitInterceptor).addPathPatterns("/**");
WebMvcConfigurer.super.addInterceptors(registry);
}
}
这样我们就完成了简易的拦截限速器,我们在请求的接口前添加@RequestLimit就可以限速了:
/**
* 分页查询
* @param param
* @return
*/
@RequestLimit(name = "token", limitTimes = 20, timeout = 60, timeUnit = TimeUnit.SECONDS)
@GetMapping("/page")
public R page(TagParam param) {
Query query = param.toQuery();
PageInfo<Tag> pageInfo = tagService.page(query);
return R.ok().put("pageInfo", pageInfo);
}
6、BoundValueOperations 使用
6.1 BoundValueOperations
就是一个绑定key的对象,我们可以通过这个对象来进行与key相关的操作
BoundValueOperations boundValueOps = redisTemplate.boundValueOps("token");
6.2 set(V value)
给绑定键重新设置值(如果没有值,则会添加这个值)
boundValueOps.set("token");
6.3 get()
获取绑定键的值。
String str = (String) boundValueOps.get();
System.out.println(str);
6.4 set(V value, long timeout, TimeUnit unit)
给绑定键设置新值并设置过期时间
boundValueOps.set("token",30, TimeUnit.SECONDS);
6.5 getAndSet(V value)
如果有这个值则获取没有则设置
String oldValue = (String) boundValueOps.getAndSet("token");
String newValue = (String) boundValueOps.get();
6.6 increment(double delta)和increment(long delta)
它是Redis的自增长键,前提是绑定值的类型是double或long类型。increment是单线程的,所以它是安全的。
BoundValueOperations boundValueOps = redisTemplate.boundValueOps("token");
boundValueOps.set(1);
System.out.println(boundValueOps.get());
boundValueOps.increment(1);
System.out.println(boundValueOps.get());
注意!使用该方法,需要使用StringRedisSerializer序列化器才能使用increment方法,否则会报错
7、代码地址
不管代码实现方式如何,还是要自己动手来实现才能体验设计的思想,让自己成长的更快,理解的更透彻。
代码地址:https://gitee.com/lhdxhl/redis.git
参考文章:https://zhuanlan.zhihu.com/p/427906048