一、语法介绍
1、枚举类型
2、结构体
3、数组
4、数组的遍历 for while foreach
扫描二维码关注公众号,回复:
1601950 查看本文章
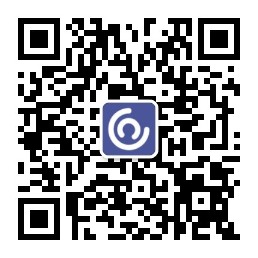
5、数组排序:快速排序和冒泡排序(见下方实例)
二、语法实践
using System; namespace 数据类型操作 { /// <summary> /// 结构体声明 /// </summary> struct EnimyPosition { public float pos1; public float pos2; public float pos3; } /// <summary> /// 枚举类型定义 /// </summary> enum GameState//默认为int类型,也可以使用冒号来继承其他整形,索引从0开始,如果认为赋值后,索引值为所赋的值,索引从所赋的值开始 { Start, Pause, Success, Failed } /// <summary> /// 方向 /// </summary> enum Direction { East,//东 West,//西 South,//南 North//北 } /// <summary> /// 路径 /// </summary> struct Way { public float distance; public Direction direction; } class Program { static void Main(string[] args) { //类型转换 byte mByte = 25; int mInt = mByte;//隐式转换 小转大 mByte = (byte)mInt;//显示转换 大转小 括号内为需要转换到的类型 string str = "123"; int num = Convert.ToInt32(str);//使用Convert进行类型转换 //枚举类型 GameState mState = GameState.Start; if (mState == GameState.Start) { Console.WriteLine("当前处于游戏开始状态"); } //结构体使用 EnimyPosition enimyPosition; enimyPosition.pos1 = 1; enimyPosition.pos2 = 2; enimyPosition.pos3 = 3; //结构体练习 //定义一个表示路径的结构,路径有一个方向和一个距离组成,方向有东西南北 //思路:方向可定义为枚举类型,距离可以定义在结构体中 Way bjTogz; bjTogz.direction = Direction.North; bjTogz.distance = 2137.57f; //数组声明方式 int[] scores1 = { 6, 14, 7, 8 }; int[] scores2 = new int[10]; int[] scores3 = new int[5] { 81, 3235, 46754, 754 ,34634}; //数组的遍历 int[] scores4 = new int[8] { 81, 3235, 46754, 754, 34634 , 252, 346, 54674}; //for循环遍历 for (int i = 0; i < scores4.Length; i++) { Console.WriteLine("使用for循环遍历的结果是:{0}" + scores4[i]); } //while循环遍历 int index = 0; while (index < scores4.Length) { Console.WriteLine("使用while循环遍历的结果是:{0}" + scores4[index]); index++; } //foreach循环遍历 foreach (int item in scores4) { Console.WriteLine("使用foreach循环遍历的结果是:{0}" + item); } //字符串特殊处理 string mUrl = " www.Wang DouDou.com "; for (int i = 0; i < mUrl.Length; i++) { Console.WriteLine("网址的每一个字符的输出内容是:{0}", mUrl[i]); } string str1 = mUrl.ToLower(); Console.WriteLine("全部转换为小写的输出内容是:{0}", str1); string str2 = mUrl.ToUpper(); Console.WriteLine("全部转换为大写的输出内容是:{0}", str2); string str3 = mUrl.Trim(); Console.WriteLine("去掉字符串所有空格的输出内容是:{0}", str3); string str4 = mUrl.TrimStart(); Console.WriteLine("去除字符串起始位置空格后的输出内容是:{0}", str4); string str5 = mUrl.TrimEnd(); Console.WriteLine("去除字符串末尾位置空格后的输出内容是:{0}", str5); string[] str6 = mUrl.Split('.'); foreach (string item in str6) { Console.WriteLine("通过点分割后的字符串的输出内容是:{0}", item); } //猜数字游戏 //生成一个0到50的随机数,让用户来猜,如果用户猜大或猜小了,提示用户猜大或猜小了,并提示用户继续输入,如果正确,则恭喜用户,并退出 Random random = new Random(); int number = random.Next(0, 51); Console.WriteLine("我们来玩个游戏吧,请猜猜我现在想的一个0-50之间的数是多少,输入你的答案吧!"); while (true) { int input = Convert.ToInt32(Console.ReadLine()); if (input > number) { Console.WriteLine("您猜大了,请继续"); } else if (input < number) { Console.WriteLine("您猜小了,请继续"); } else if(input == number) { Console.WriteLine("恭喜您猜对了"); break; } } //练习:接收用户输入的一串数字,使用空格符分割,并将用户输入的数字数组进行排序 string inputStr = Console.ReadLine(); string[] tempStrArr = inputStr.Split(' '); int[] tempIntArr = new int[tempStrArr.Length]; for (int i = 0; i < tempStrArr.Length; i++) { int temp = Convert.ToInt32(tempStrArr[i]); tempIntArr[i] = temp; } Array.Sort(tempIntArr);//使用内置的自带数组排序(快速排序) //冒泡排序 for (int i = 0; i < tempIntArr.Length - 1; i++) { for (int j = 0; j < tempIntArr.Length - i; j++) { if (tempIntArr[i + 1] < tempIntArr[i]) { int temp = tempIntArr[i]; tempIntArr[i] = tempIntArr[i + 1]; tempIntArr[i + 1] = temp; } } } for (int i = 0; i < tempIntArr.Length; i++) { Console.WriteLine("排序后的数组内容是:" + tempIntArr[i]); } Console.ReadKey(); } } }