接下来我们来学习怎么编写一个IIC设备驱动。
1、驱动程序分析
我们先在Linux内核代码中打开一个叫做At24.c的文件,只要是属于AT24开头的设备都可以使用这个驱动。我们接下来分析这个驱动。
/*-------------------------------------------------------------------------*/ static struct i2c_driver at24_driver = { .driver = { .name = "at24", .owner = THIS_MODULE, }, .probe = at24_probe, .remove = __devexit_p(at24_remove), .id_table = at24_ids, }; static int __init at24_init(void) { io_limit = rounddown_pow_of_two(io_limit); return i2c_add_driver(&at24_driver); } module_init(at24_init); static void __exit at24_exit(void) { i2c_del_driver(&at24_driver); } module_exit(at24_exit);
初始化函数中主要是注册一个I2C设备驱动。我们分析(&at24_driver);里面的成员,比较重要的成员有2个,一个是probe函数,另一个是at24_ids,这里面存放支持AT24设备的ID列表,比如说AT24C02,AT24C08等等,有兴趣的可以看一下这个表。
我们接下来分析probe函数。这个函数很长,如果要做到读懂每一行代码肯定是非常难的,我们最基本的应该掌握这个函数大概的流程和中心。比如说注册某个东西,创建某个东西,初始化某个东西。
/*-------------------------------------------------------------------------*/ static int at24_probe(struct i2c_client *client, const struct i2c_device_id *id) { struct at24_platform_data chip; bool writable; bool use_smbus = false; struct at24_data *at24; int err; unsigned i, num_addresses; kernel_ulong_t magic; if (client->dev.platform_data) { chip = *(struct at24_platform_data *)client->dev.platform_data; } else { if (!id->driver_data) { err = -ENODEV; goto err_out; } magic = id->driver_data; chip.byte_len = BIT(magic & AT24_BITMASK(AT24_SIZE_BYTELEN)); magic >>= AT24_SIZE_BYTELEN; chip.flags = magic & AT24_BITMASK(AT24_SIZE_FLAGS); /* * This is slow, but we can't know all eeproms, so we better * play safe. Specifying custom eeprom-types via platform_data * is recommended anyhow. */ chip.page_size = 1; chip.setup = NULL; chip.context = NULL; } if (!is_power_of_2(chip.byte_len)) dev_warn(&client->dev, "byte_len looks suspicious (no power of 2)!\n"); if (!is_power_of_2(chip.page_size)) dev_warn(&client->dev, "page_size looks suspicious (no power of 2)!\n"); /* Use I2C operations unless we're stuck with SMBus extensions. */ if (!i2c_check_functionality(client->adapter, I2C_FUNC_I2C)) { if (chip.flags & AT24_FLAG_ADDR16) { err = -EPFNOSUPPORT; goto err_out; } if (!i2c_check_functionality(client->adapter, I2C_FUNC_SMBUS_READ_I2C_BLOCK)) { err = -EPFNOSUPPORT; goto err_out; } use_smbus = true; } if (chip.flags & AT24_FLAG_TAKE8ADDR) num_addresses = 8; else num_addresses = DIV_ROUND_UP(chip.byte_len, (chip.flags & AT24_FLAG_ADDR16) ? 65536 : 256); at24 = kzalloc(sizeof(struct at24_data) + num_addresses * sizeof(struct i2c_client *), GFP_KERNEL); if (!at24) { err = -ENOMEM; goto err_out; } mutex_init(&at24->lock); at24->use_smbus = use_smbus; at24->chip = chip; at24->num_addresses = num_addresses; /* * Export the EEPROM bytes through sysfs, since that's convenient. * By default, only root should see the data (maybe passwords etc) */ at24->bin.attr.name = "eeprom"; at24->bin.attr.mode = chip.flags & AT24_FLAG_IRUGO ? S_IRUGO : S_IRUSR; at24->bin.read = at24_bin_read; at24->bin.size = chip.byte_len; at24->macc.read = at24_macc_read; writable = !(chip.flags & AT24_FLAG_READONLY); if (writable) { if (!use_smbus || i2c_check_functionality(client->adapter, I2C_FUNC_SMBUS_WRITE_I2C_BLOCK)) { unsigned write_max = chip.page_size; at24->macc.write = at24_macc_write; at24->bin.write = at24_bin_write; at24->bin.attr.mode |= S_IWUSR; if (write_max > io_limit) write_max = io_limit; if (use_smbus && write_max > I2C_SMBUS_BLOCK_MAX) write_max = I2C_SMBUS_BLOCK_MAX; at24->write_max = write_max; /* buffer (data + address at the beginning) */ at24->writebuf = kmalloc(write_max + 2, GFP_KERNEL); if (!at24->writebuf) { err = -ENOMEM; goto err_struct; } } else { dev_warn(&client->dev, "cannot write due to controller restrictions."); } } at24->client[0] = client; /* use dummy devices for multiple-address chips */ for (i = 1; i < num_addresses; i++) { at24->client[i] = i2c_new_dummy(client->adapter, client->addr + i); if (!at24->client[i]) { dev_err(&client->dev, "address 0x%02x unavailable\n", client->addr + i); err = -EADDRINUSE; goto err_clients; } } err = sysfs_create_bin_file(&client->dev.kobj, &at24->bin); if (err) goto err_clients; i2c_set_clientdata(client, at24); dev_info(&client->dev, "%zu byte %s EEPROM %s\n", at24->bin.size, client->name, writable ? "(writable)" : "(read-only)"); dev_dbg(&client->dev, "page_size %d, num_addresses %d, write_max %d%s\n", chip.page_size, num_addresses, at24->write_max, use_smbus ? ", use_smbus" : ""); /* export data to kernel code */ if (chip.setup) chip.setup(&at24->macc, chip.context); return 0; err_clients: for (i = 1; i < num_addresses; i++) if (at24->client[i]) i2c_unregister_device(at24->client[i]); kfree(at24->writebuf); err_struct: kfree(at24); err_out: dev_dbg(&client->dev, "probe error %d\n", err); return err; }
这里好像没有注册什么东西,那么有没有创建某个东西呢?可以看到他在sys下面创建了一个文件err = sysfs_create_bin_file(&client->dev.kobj, &at24->bin);这个文件包含什么东西呢?查看之前对于at24->bin的初始化,可以知道,这个文件叫做eeprom,同时可以猜测,当我们使用这个设备文件来读写eeprom时需要使用到文件里面实现读写操作的函数,
查看代码我们也可以知道这2个函数分别是at24_bin_read和at24_bin_write。我们接下来就分析这2个函数。
先来分析一下写函数at24_bin_write,这个函数调用at24_write,然后再调用at24_eeprom_write函数,实现对eeprom的写操作,我们现在分析这个函数:
/* * Note that if the hardware write-protect pin is pulled high, the whole * chip is normally write protected. But there are plenty of product * variants here, including OTP fuses and partial chip protect. * * We only use page mode writes; the alternative is sloooow. This routine * writes at most one page. */ static ssize_t at24_eeprom_write(struct at24_data *at24, const char *buf, unsigned offset, size_t count) { struct i2c_client *client; struct i2c_msg msg; ssize_t status; unsigned long timeout, write_time; unsigned next_page; /* Get corresponding I2C address and adjust offset */ client = at24_translate_offset(at24, &offset); /* write_max is at most a page */ if (count > at24->write_max) count = at24->write_max; /* Never roll over backwards, to the start of this page */ next_page = roundup(offset + 1, at24->chip.page_size); if (offset + count > next_page) count = next_page - offset; /* If we'll use I2C calls for I/O, set up the message */ if (!at24->use_smbus) { int i = 0; msg.addr = client->addr; msg.flags = 0; /* msg.buf is u8 and casts will mask the values */ msg.buf = at24->writebuf; if (at24->chip.flags & AT24_FLAG_ADDR16) msg.buf[i++] = offset >> 8; msg.buf[i++] = offset; memcpy(&msg.buf[i], buf, count); msg.len = i + count; } /* * Writes fail if the previous one didn't complete yet. We may * loop a few times until this one succeeds, waiting at least * long enough for one entire page write to work. */ timeout = jiffies + msecs_to_jiffies(write_timeout); do { write_time = jiffies; if (at24->use_smbus) { status = i2c_smbus_write_i2c_block_data(client, offset, count, buf); if (status == 0) status = count; } else { status = i2c_transfer(client->adapter, &msg, 1); if (status == 1) status = count; } dev_dbg(&client->dev, "write %zu@%d --> %zd (%ld)\n", count, offset, status, jiffies); if (status == count) return count; /* REVISIT: at HZ=100, this is sloooow */ msleep(1); } while (time_before(write_time, timeout)); return -ETIMEDOUT; }
简要分析一下可以知道,它主要做了2件事,一个是构造msg,另一件事是使用i2c_transfer函数来传输数据,上一节的分析可以知道,i2c设备驱动如果要读写数据,都是通过i2c_transfer把它交给i2c总线驱动或者说是i2c控制器驱动。对应msg的构造我们在上一节课已经讲的非常清楚了,对于i2c_transfer函数,它是属于i2c-core里面的函数,查看他的代码可以知道,他并没有做什么实质性的操作,而是调用i2c适配器里面的读写方法来实现数据的传输 ret = adap->algo->master_xfer(adap, msgs, num);
接下来分析读函数,读函数和写函数的调用过程也类似,依次调用at24_bin_read->at24_read->at24_eeprom_read来实现eeprom的读数据,at24_eeprom_read的实现过程也主要分为msg的构造和数据的传输,msg的构造和上一节课讲的消息构造差不多,也是需要分为2个消息,一个是写消息,然后是读消息的构造。
if (at24->chip.flags & AT24_FLAG_ADDR16) msgbuf[i++] = offset >> 8; msgbuf[i++] = offset; msg[0].addr = client->addr; msg[0].buf = msgbuf; msg[0].len = i; msg[1].addr = client->addr; msg[1].flags = I2C_M_RD; msg[1].buf = buf; msg[1].len = count;
2、驱动程序移植
我们现在来对Linux的i2c驱动代码进行修改和移植。首先是注册i2c设备,为什么要注册i2c设备呢?我们知道当Linux系统检测到符合id_table的设备时将会调用probe函数,因此,我们不仅需要注册i2c驱动,还需要注册i2c设备。怎么样才能注册一个i2c设备呢?
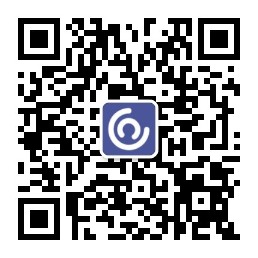
对于每一个IIC设备都会有一个结构来描述,比如说AT24C08:
static struct at24_platform_data at24 = { .byte_len = 8*1024/8, .page_size = 16, .flags = 0, }; static struct i2c_board_info __initdata mini2440_i2c_devices[]= { { I2C_BOARD_INFO("24c08", 0x50), .platform_data = &at24c08, } };由于可能有多个i2c设备,所以把它们保存在一个数组里面。
然后使用i2c_register_board_info函数把我们定义的i2c设备数组注册到Linux内核中:
i2c_register_board_info(0, mini2440_i2c_devices, ARRAY_SIZE(mini2440_i2_devices));
那么在哪里注册这个i2c设备呢?一般是在开发板的系统初始化里面,对应mini2440是在/arch/arm/mach-s3c2440/Mach-mini2440.c文件中的mini2440_machine_init函数中注册。加上下面这2个头文件。
#include<linux/i2c.h>
#include<linux/i2c/at24.h>
这样就算是移植好了i2c的驱动。
接下来还需要再内核中启动对eeprom的支持:
#make menuconfig
选择device driver,选中并进入Misc devices,然后进入 EEPROM Support,选择里面全部的配置,这样就把eeprom的驱动配置好了,然后当注册i2c设备的时候,就会调用probe函数,从而生成/sys/bus/i2c/devices/0-0050/eeprom文件
编译内核:
#make uImage ARCH=arm CROSS_COMPILE=arm-linux-
3、驱动程序测试
测试程序我们分为这几步:
1、打开文件
2、写入数据s
3、读出数据
4、打印
#include <stdio.h> #include <unistd.h> #include <fcntl.h> int main() { int fd; int i; char write_data[256]; char read_data[256]={0}; //打开文件 fd = open("/sys/bus/i2c/devices/0-0050/eeprom", O_RDWR); //写入数据 for (i=0; i<256; i++) write_data[i] = i; lseek(fd, 0, SEEK_SET); write(fd, write_data, 256); //读出数据 lseek(fd, 0, SEEK_SET); read(fd, read_data, 256); //打印数据 for (i=0; i<256; i++) printf("%3d\n", read_data[i]); //关闭文件 close(fd); return 0; }
编译这个程序:
#arm-linux-gcc -stati app.c -o app
然后把它拷贝到开发板上运行
打印出的信息和写入的应该是一样的。