目录
扫描二维码关注公众号,回复:
15947879 查看本文章
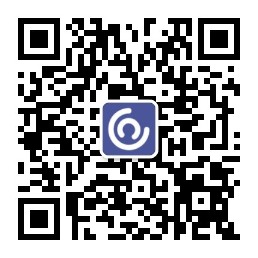
准备工作
本文以实体类为Car对象进行统一分析处理,其对应的数据库中的表如下
java中的Car对象如下:
/**
* @author 风轻云淡
*/
@Data
public class Car {
/**
* 包装类防止null问题
*/
private Long id;
private String carNum;
private String brand;
private Double guidePrice;
private String produceTime;
private String carType;
}
返回对象为pojo实体类(Car)
对应的接口
/**
* 根据id查询
* @param id
* @return Car对象
*/
Car selectById(Long id);
对应的xml文件
<select id="selectById" resultType="pojo.Car">
select
id as id ,
car_num as carNum,
brand as brand,
guide_price as guidePrice,
car_type as carType,
produce_time as produceTime
from t_car where id=#{id}
</select>
resultType我个人的理解就是我们查询sql语句返回的结构集的行的玩意,也就是一个记录
对应的测试代码:
@Test
public void test01(){
SqlSession session = SqlSessionUtil.openSession();
CarMapper mapper = session.getMapper(CarMapper.class);
Car car = mapper.selectById(3L);
System.out.println(car);
}
返回集合对象(List<Car>)
当查询的记录条数是多条的时候,必须使用集合接收。如果使用单个实体类接收会出现异常。如果返回的是一条记录可以用集合接收
对应的接口
/**
* 查询所有car
* @return
*/
List<Car> selectAll();
对应的xml文件
<select id="selectAll" resultType="pojo.Car">
select
id as id ,
car_num as carNum,
brand as brand,
guide_price as guidePrice,
car_type as carType,
produce_time as produceTime
from t_car
</select>
对应的测试代码:
@Test
public void test02(){
SqlSession session = SqlSessionUtil.openSession();
CarMapper mapper = session.getMapper(CarMapper.class);
List<Car> cars = mapper.selectAll();
System.out.println(cars);
}
返回Map
当返回的数据,没有合适的实体类对应的话,可以采用Map集合接收。字段名做key,字段值做value。查询如果可以保证只有一条数据,则返回一个Map集合即可。
对应的接口
/**
* 通过id查询一条记录,返回Map集合
* @param id
* @return
*/
Map<String, Object> selectByIdRetMap(Long id);
对应的xml文件
<select id="selectByIdRetMap" resultType="map">
select id,car_num carNum,brand,guide_price guidePrice,produce_time produceTime,car_type carType from t_car where id = #{id}
</select>
resultMap="map",这是因为mybatis内置了很多别名。【参见mybatis开发手册】
对应的测试代码:
@Test
public void test03(){
SqlSession session = SqlSessionUtil.openSession();
CarMapper mapper = session.getMapper(CarMapper.class);
Map<String, Object> map = mapper.selectByIdRetMap(3L);
System.out.println(map);
}
当然,如果返回一个Map集合,可以将Map集合放到List集合中吗?当然可以,这里就不再测试了。
反过来,如果返回的不是一条记录,是多条记录的话,只采用单个Map集合接收,这样同样会出现之前的异常:TooManyResultsException
返回List<Map>
查询结果条数大于等于1条数据,则可以返回一个存储Map集合的List集合。List<Map>等同于List<Car>
对应的接口
/**
* 查询所有的Car,返回一个List集合。List集合中存储的是Map集合。
* @return
*/
List<Map<String,Object>> selectAllRetListMap();
对应的xml文件
<select id="selectAllRetListMap" resultType="map">
select
id,car_num
carNum,brand,
guide_price
guidePrice,
produce_time
produceTime,car_type carType
from t_car
</select>
对应的测试代码:
@Test
public void test04(){
SqlSession session = SqlSessionUtil.openSession();
CarMapper mapper = session.getMapper(CarMapper.class);
List<Map<String, Object>> list = mapper.selectAllRetListMap();
System.out.println(list);
}
返回Map<String,Map>
拿Car的id做key,以后取出对应的Map集合时更方便。
对应的接口
/**
* 获取所有的Car,返回一个Map集合。
* Map集合的key是Car的id。
* Map集合的value是对应Car。
* @return
*/
@MapKey("id")
Map<Long,Map<String,Object>> selectAllRetMap();
@MapKey("id")指定一个字段作为返回Map中的key,一般使用唯一键来做key
对应的xml文件
<select id="selectAllRetMap" resultType="map">
select
id,
car_num carNum,
brand,
guide_price guidePrice,
produce_time produceTime,
car_type carType
from t_car
</select>
对应的测试代码:
@Test
public void test05(){
SqlSession session = SqlSessionUtil.openSession();
CarMapper mapper = session.getMapper(CarMapper.class);
Map<Long, Map<String, Object>> map = mapper.selectAllRetMap();
System.out.println(map);
}
返回总记录条数
需求:查询总记录条数
对应的接口
/**
* 获取总记录条数
* @return
*/
Long selectTotal();
对应的xml文件
<!--long是别名,可参考mybatis开发手册。-->
<select id="selectTotal" resultType="long">
select count(*) from t_car
</select>
对应的测试代码:
@Test
public void testSelectTotal(){
CarMapper carMapper = SqlSessionUtil.openSession().getMapper(CarMapper.class);
Long total = carMapper.selectTotal();
System.out.println(total);
}
要点
- (1)resultType 属性可以指定结果集的类型,它支持基本类型和实体类类型。我们在前面的 CRUD 案例中已经对此属性进行过应用了。
- (2)需要注意的是,它和 parameterType 一样,如果注册过类型别名的,可以直接使用别名。没有注册过的必须使用全限定类名。例如:我们的实体类此时必须是全限定类名
- (3)同时,当是实体类名称是,还有一个要求,实体类中的属性名称必须和查询语句中的列名保持一致,否则无法实现封装。