定义排序函数:
方法1:声明外部比较函数
bool Less(const Student& s1, const Student& s2) { return s1.name < s2.name; //从小到大排序 } std::sort(sutVector.begin(), stuVector.end(), Less);
注意:比较函数必须写在类外部(全局区域)或声明为静态函数
当comp作为类的成员函数时,默认拥有一个this指针,这样和sort函数所需要使用的排序函数类型不一样。
否则,会出现错误
方法2:重载类的比较运算符
bool operator<(const Student& s1, const Student& s2) { return s1.name < s2.name; //从小到大排序 } std::sort(sutVector.begin(), stuVector.end());
方法3:声明比较类
struct Less { bool operator()(const Student& s1, const Student& s2) { return s1.name < s2.name; //从小到大排序 } }; std::sort(sutVector.begin(), stuVector.end(), Less());
(一)为什么要用c++标准库里的排序函数Sort()函数是c++一种排序方法之一,学会了这种方法也打消我学习c++以来使用的冒泡排序和选择排序所带来的执行效率不高的问题!因为它使用的排序方法是类似于快排的方法,时间复杂度为n*log2(n),执行效率较高!(二)c++标准库里的排序函数的使用方法I)Sort函数包含在头文件为 #include<algorithm>的c++标准库中,调用标准库里的排序方法可以不必知道其内部是如何实现的,只要出现我们想要的结果即可! II)Sort函数有三个参数:( 1 )第一个是要排序的数组的起始地址。( 2 )第二个是结束的地址(最后一位要排序的地址)( 3 )第三个参数是排序的方法,可以是从大到小也可是从小到大,还可以不写第三个参数,此时默认的排序方法是从小到大排序。Sort函数使用模板:Sort(start,end,,排序方法)下面就具体使用sort()函数结合对数组里的十个数进行排序做一个说明!例一:sort函数没有第三个参数,实现的是从小到大 #include<iostream> #include<algorithm> using namespace std ; int main(){ int a[ 10 ]={ 9 , 6 , 3 , 8 , 5 , 2 , 7 , 4 , 1 , 0 }; for ( int i= 0 ;i< 10 ;i++) cout <<a[i]<<endl;sort(a,a+ 10 ); for ( int i= 0 ;i< 10 ;i++) cout <<a[i]<<endl; return 0 ;}例二通过上面的例子,会产生疑问:要实现从大到小的排序肿么办? 这就如前文所说需要在sort()函数里的第三个参数里做文章了,告诉程序我要从大到小排序!需要加入一个比较函数 complare(),此函数的实现过程是这样的 bool complare( int a, int b){ return a>b;}这就是告诉程序要实现从大到小的排序的方法! #include<iostream> #include<algorithm> using namespace std ; bool complare( int a, int b){ return a>b;} int main(){ int a[ 10 ]={ 9 , 6 , 3 , 8 , 5 , 2 , 7 , 4 , 1 , 0 }; for ( int i= 0 ;i< 10 ;i++) cout <<a[i]<<endl; sort(a,a+ 10 ,complare); //在这里就不需要对complare函数传入参数了,//这是规则 for ( int i= 0 ;i< 10 ;i++) cout <<a[i]<<endl; return 0 ;}例三:通过上面例一、二的方法虽然实现了从大到小和从大到小的排序,这样做还是有点麻烦,因为还需要自己编写告诉程序进行何种排序的函数,c++标准库强大的功能完全可以解决这种麻烦。Sortt函数的第三个参数可以用这样的语句告诉程序你所采用的排序原则less<数据类型>() //从小到大排序 greater<数据类型>() //从大到小排序 结合本例子,这样的就可以完成你想要的任何一种排序原则了 #include<iostream> #include<algorithm> using namespace std ; int main(){ int a[ 10 ]={ 9 , 6 , 3 , 8 , 5 , 2 , 7 , 4 , 1 , 0 }; for ( int i= 0 ;i< 10 ;i++) cout <<a[i]<<endl;sort(a,a+ 10 ,less< int >()); for ( int i= 0 ;i< 10 ;i++) cout <<a[i]<<endl; return 0 ;} #include<iostream> #include<algorithm> using namespace std ; int main(){ int a[ 10 ]={ 9 , 6 , 3 , 8 , 5 , 2 , 7 , 4 , 1 , 0 }; for ( int i= 0 ;i< 10 ;i++) cout <<a[i]<<endl; sort(a,a+ 10 ,greater< int >()); for ( int i= 0 ;i< 10 ;i++) cout <<a[i]<<endl; return 0 ;}例四:利用sort函数还可以实现对字符的排序,排序方法大同小异,下面就把程序范例展示一下 #include<iostream> #include<algorithm> using namespace std ; int main(){ char a[ 11 ]= "asdfghjklk" ; for ( int i= 0 ;i< 10 ;i++) cout <<a[i]<<endl; sort(a,a+ 10 ,greater< char >()); for ( int i= 0 ;i< 10 ;i++) cout <<a[i]<<endl; return 0 ;}
扫描二维码关注公众号,回复:
1592075 查看本文章
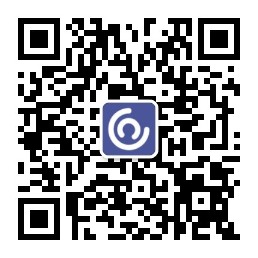