js判断是否为null,undefined,NaN,空串或者空对象
这里写目录标题
特殊值
null
是一个特殊的值,表示一个空或者不存在的对象引用,表示一个变量被人为的设置为空对象,不是原始状态。
undefined
当一个变量被声明,但没有赋值时,它的默认值是 undefined。此外,当访问不存在的对象属性或数组元素时,结果也是 undefined。
NaN
(Not a Number)是一个特殊的值,表示在执行数学运算时,结果不是一个合法的数字。NaN 属于 Number 类型,它与任何值(包括 NaN 本身)都不相等。
出现NaN的情况:
- 数学运算中的无效操作,例如:
0 / 0
或Math.sqrt(-1)
- 将非数字的值强制转换为数字时,例如:
parseInt("abc")
或Number("xyz")
空字符串(“”)
一个空字符串是一个长度为 0 的字符串。它不是 null 或 undefined,而是一个完全有效的字符串,只是它没有内容。
空对象({})
空对象是一个没有任何属性的对象。它不是 null、undefined 或空字符串,而是一个有效的 JavaScript 对象。
typeof函数
基本类型:String、Number、Boolean、Symbol、Undefined、Null
引用类型:Object(object、array、function)
typeof '' // "string"
typeof 'Bill Gates' // "string"
typeof 0 // "number"
typeof 3.14 // "number"
typeof false // "boolean"
typeof true // "boolean"
typeof Symbol() // "symbol"
typeof Symbol('mySymbol'); // "symbol"
typeof undefined // "undefined"
typeof null // "object"
typeof {name:'Bill', age:62} // "object"
typeof [0, 1, 2] // "object"
typeof function(){} // "function"
typeof (()=>{}) // "function"
typeof testnull // "function" testnull是函数名
判断undefined
let a = undefined;
if (typeof (a) === "undefined" || a === undefined) {
console.log("undefined");
}

判断null
需要注意的是 typeof(null) = object
let b = null;
console.log(typeof (b));
if (b === null) {
console.log("null1");
}
// !key && typeof(key)!=undefined 过滤完之后只剩 null 和 0 了,再用一个 key!=0 就可以把 0 过滤掉了
if (!b && typeof (b) != "undefined" && b != 0) {
console.log("null2");
}
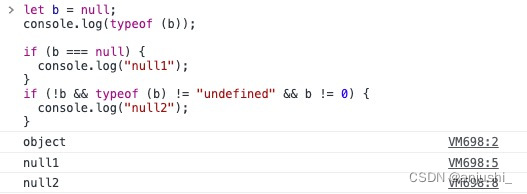
undefined和null
if(a == null) { // 等同于 a === undefined || a === null
console.log("为null");
}
undefined 和 null 用 == 比较是相等的
null 的类型是 object,undefined 的类型是 undefined
== 是先把左右两边转化为相同的类型,再进行区分
=== 和 == 的理解
=== :严格相等,会先比较类型,再比较值
== :宽松相等,是先把左右两边转化为相同的类型,再进行区分,仅要求值相等,而不要求类型
true 转化为整数后是 1,false 转化为整数后是 0
https://cloud.tencent.com/developer/article/1703891
https://blog.csdn.net/qq_42533666/article/details/129190944
判断NaN
NaN具有非自反的特点,所谓的非自反就是说,NaN 与谁都不相等,包括它本身,但在 NaN != NaN 下会返回true,可以根据这个特性判断
let num = NaN;
let num2 = 0/0;
if (typeof (num) === "number" && isNaN(num)) {
console.log("num is NaN1");
}
if (num2 != num2) {
console.log('num is NaN2');
}
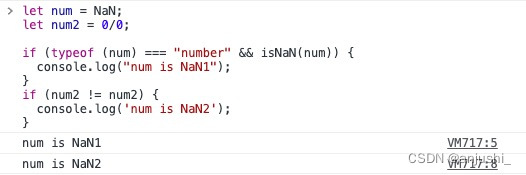
https://blog.csdn.net/Lu5957/article/details/122609879
https://blog.csdn.net/qq_43563538/article/details/103815482
https://www.cnblogs.com/k1023/p/11851943.html
判断空字符串
直接将其与空字符串比较,或者调用 trim() 函数去掉前后的空格,然后判断字符串的长度
- 判断字符串为空(空格算空)
let str = " ";
if (str === '' || str.trim().length === 0){
console.log('empty str 1');
}
if (typeof str == 'string' && str.length > 0) {
console.log('not empty str');
}
- 判断字符串不为空(空格不算空)
if (typeof s == 'string' && s.length > 0) {
console.log('not empty str');
}
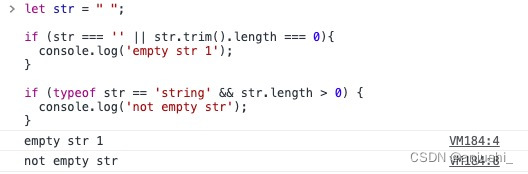
https://blog.csdn.net/K346K346/article/details/113182838
判断对象为空
使用JSON.stringify()
将 JavaScript 值转换为 JSON 字符串,再判断该字符串是否为"{}"
let data = {};
if (JSON.stringify(data) === '{}') {
console.log('empty obj 1');
}
使用es6的方法Object.keys()
if (Object.keys(data).length === 0) {
console.log('empty obj 2');
}
for in 循环判断
通过 for…in 语句遍历变量的属性
if (judge(data)) {
console.log('empty obj 3');
}
function judge(data) {
for (let key in data) {
return false;
}
return true;
}
使用Object对象的getOwnPropertyNames方法
使用Object对象的getOwnPropertyNames方法,获取到对象中的属性名,存到一个数组中,返回数组对象,通过判断数组的length来判断此对象是否为空
if (Object.getOwnPropertyNames(data).length == 0) {
console.log('empty obj 4');
}
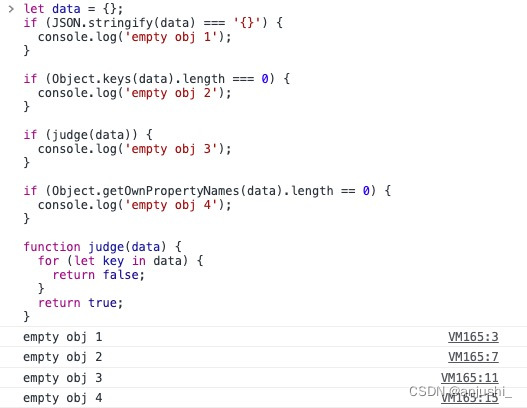
https://www.jb51.net/article/209387.htm
https://www.cnblogs.com/shanhubei/p/16969190.html
https://blog.csdn.net/qq_38709383/article/details/123224833
判断空数组
首先判断变量是否为数组,然后通过 length 属性确定
let arr = [];
if (arr.length == 0) {
console.log('empty arr 1');
}
if (arr instanceof Array && !arr.length) {
console.log('empty arr 2');
}
if (Array.isArray(arr) && !arr.length) {
console.log('empty arr 3');
}
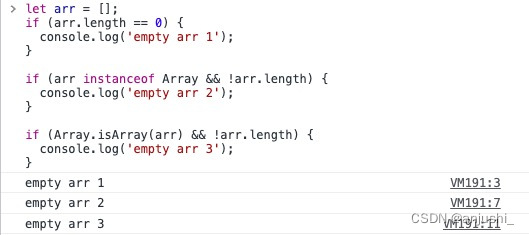