写了好一阵子终于把微信小程序的登陆功能实现了,之前就因为返回openid耽误了好一段时间,所以今天就跟大家分享一下java后台的返回openid及解密用户信息。
微信小程序前端:
js页面:
wx.login({
success:
function (res) {
var code = res.code;
//登录凭证
if (code) {
//2、调用获取用户信息接口
wx.getUserInfo({
success:
function (res) {
console.log({ encryptedData: res.encryptedData, iv: res.iv, code: code })
//3.请求自己的服务器,解密用户信息 获取unionId等加密信息
wx.request({
url:
'http://域名/**.action',
//自己的服务接口地址
method:
'get',
header: {
"Content-Type":
"applciation/json"
},
data: { encryptedData: res.encryptedData, iv: res.iv, code: code },
success:
function (data) {
//4.解密成功后 获取自己服务器返回的结果
if (data.data.status ==
1) {
var userInfo_ = data.data.userInfo;
console.log(userInfo_)
}
else {
console.log(
'解密失败')
}
},
fail:
function () {
console.log(
'系统错误')
}
})
},
fail:
function () {
console.log(
'获取用户信息失败')
}
})
}
else {
console.log(
'获取用户登录态失败!' + r.errMsg)
}
},
fail:
function () {
console.log(
'登陆失败')
}
})
java后台:我是用ssm搭的框架
spring+springMVC+mybatis+sqlserver
先上controller的代码:
@ResponseBody @RequestMapping(value = "/decodeUserInfo", method = RequestMethod.GET) public Map decodeUserInfo(String encryptedData, String iv, String code) { Map map = new HashMap(); //登录凭证不能为空 if (code == null || code.length() == 0) { map.put("status", 0); map.put("msg", "code 不能为空"); return map; } //小程序唯一标识 (在微信小程序管理后台获取) String wxspAppid = "***********************; //小程序的 app secret (在微信小程序管理后台获取) String wxspSecret = "************************"; //授权(必填) String grant_type = "***************************"; //////////////// 1、向微信服务器 使用登录凭证 code 获取 session_key 和 openid //////////////// //请求参数 String params = "appid=" + wxspAppid + "&secret=" + wxspSecret + "&js_code=" + code + "&grant_type=" + grant_type; //发送请求 String sr = HttpRequest.sendGet("https://api.weixin.qq.com/sns/jscode2session", params); //解析相应内容(转换成json对象) JSONObject json = JSONObject.fromObject(sr); //获取会话密钥(session_key) String session_key = json.get("session_key").toString(); //用户的唯一标识(openid) String openid = (String) json.get("openid"); //////////////// 2、对encryptedData加密数据进行AES解密 //////////////// try { String result = AesCbcUtil.decrypt(encryptedData, session_key, iv, "UTF-8"); if (null != result && result.length() > 0) { map.put("status", 1); map.put("msg", "解密成功"); JSONObject userInfoJSON = JSONObject.fromObject(result); Map userInfo = new HashMap(); userInfo.put("openId", userInfoJSON.get("openId")); userInfo.put("nickName", userInfoJSON.get("nickName")); userInfo.put("gender", userInfoJSON.get("gender")); userInfo.put("city", userInfoJSON.get("city")); userInfo.put("province", userInfoJSON.get("province")); userInfo.put("country", userInfoJSON.get("country")); userInfo.put("avatarUrl", userInfoJSON.get("avatarUrl")); userInfo.put("unionId", userInfoJSON.get("unionId")); map.put("userInfo", userInfo); return map; } } catch (Exception e) { e.printStackTrace(); } map.put("status", 0); map.put("msg", "解密失败"); return map; }
解密工具包AesCbcUtil.class
扫描二维码关注公众号,回复:
1582912 查看本文章
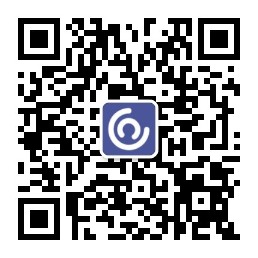
package com.zking.util; import org.apache.commons.codec.binary.Base64; import org.bouncycastle.jce.provider.BouncyCastleProvider; import javax.crypto.BadPaddingException; import javax.crypto.Cipher; import javax.crypto.IllegalBlockSizeException; import javax.crypto.NoSuchPaddingException; import javax.crypto.spec.IvParameterSpec; import javax.crypto.spec.SecretKeySpec; import java.io.UnsupportedEncodingException; import java.security.*; import java.security.spec.InvalidParameterSpecException; public class AesCbcUtil { static { //BouncyCastle是一个开源的加解密解决方案,主页在http://www.bouncycastle.org/ Security.addProvider(new BouncyCastleProvider()); } /** * AES解密 * * @param data //密文,被加密的数据 * @param key //秘钥 * @param iv //偏移量 * @param encodingFormat //解密后的结果需要进行的编码 * @return * @throws Exception */ public static String decrypt(String data, String key, String iv, String encodingFormat) throws Exception { // initialize(); //被加密的数据 byte[] dataByte = Base64.decodeBase64(data.getBytes()); //加密秘钥 byte[] keyByte = Base64.decodeBase64(key.getBytes()); //偏移量 byte[] ivByte = Base64.decodeBase64(iv.getBytes()); try { Cipher cipher = Cipher.getInstance("AES/CBC/PKCS7Padding"); SecretKeySpec spec = new SecretKeySpec(keyByte, "AES"); AlgorithmParameters parameters = AlgorithmParameters.getInstance("AES"); parameters.init(new IvParameterSpec(ivByte)); cipher.init(Cipher.DECRYPT_MODE, spec, parameters);// 初始化 byte[] resultByte = cipher.doFinal(dataByte); if (null != resultByte && resultByte.length > 0) { String result = new String(resultByte, encodingFormat); return result; } return null; } catch (NoSuchAlgorithmException e) { e.printStackTrace(); } catch (NoSuchPaddingException e) { e.printStackTrace(); } catch (InvalidParameterSpecException e) { e.printStackTrace(); } catch (InvalidKeyException e) { e.printStackTrace(); } catch (InvalidAlgorithmParameterException e) { e.printStackTrace(); } catch (IllegalBlockSizeException e) { e.printStackTrace(); } catch (BadPaddingException e) { e.printStackTrace(); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } return null; } }请求包HttpRequest
HttpRequest
package com.zking.util; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.io.PrintWriter; import java.net.URL; import java.net.URLConnection; import java.util.List; import java.util.Map; public class HttpRequest { public static void main(String[] args) { //发送 GET 请求 String s=HttpRequest.sendGet("http://v.qq.com/x/cover/kvehb7okfxqstmc.html?vid=e01957zem6o", ""); System.out.println(s); // //发送 POST 请求 // String sr=HttpRequest.sendPost("http://www.toutiao.com/stream/widget/local_weather/data/?city=%E4%B8%8A%E6%B5%B7", ""); // JSONObject json = JSONObject.fromObject(sr); // System.out.println(json.get("data")); } /** * 向指定URL发送GET方法的请求 * * @param url * 发送请求的URL * @param param * 请求参数,请求参数应该是 name1=value1&name2=value2 的形式。 * @return URL 所代表远程资源的响应结果 */ public static String sendGet(String url, String param) { String result = ""; BufferedReader in = null; try { String urlNameString = url + "?" + param; URL realUrl = new URL(urlNameString); // 打开和URL之间的连接 URLConnection connection = realUrl.openConnection(); // 设置通用的请求属性 connection.setRequestProperty("accept", "*/*"); connection.setRequestProperty("connection", "Keep-Alive"); connection.setRequestProperty("user-agent", "Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1;SV1)"); // 建立实际的连接 connection.connect(); // 获取所有响应头字段 Map<String, List<String>> map = connection.getHeaderFields(); // 遍历所有的响应头字段 for (String key : map.keySet()) { System.out.println(key + "--->" + map.get(key)); } // 定义 BufferedReader输入流来读取URL的响应 in = new BufferedReader(new InputStreamReader( connection.getInputStream())); String line; while ((line = in.readLine()) != null) { result += line; } } catch (Exception e) { System.out.println("发送GET请求出现异常!" + e); e.printStackTrace(); } // 使用finally块来关闭输入流 finally { try { if (in != null) { in.close(); } } catch (Exception e2) { e2.printStackTrace(); } } return result; } /** * 向指定 URL 发送POST方法的请求 * * @param url * 发送请求的 URL * @param param * 请求参数,请求参数应该是 name1=value1&name2=value2 的形式。 * @return 所代表远程资源的响应结果 */ public static String sendPost(String url, String param) { PrintWriter out = null; BufferedReader in = null; String result = ""; try { URL realUrl = new URL(url); // 打开和URL之间的连接 URLConnection conn = realUrl.openConnection(); // 设置通用的请求属性 conn.setRequestProperty("accept", "*/*"); conn.setRequestProperty("connection", "Keep-Alive"); conn.setRequestProperty("user-agent", "Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1;SV1)"); // 发送POST请求必须设置如下两行 conn.setDoOutput(true); conn.setDoInput(true); // 获取URLConnection对象对应的输出流 out = new PrintWriter(conn.getOutputStream()); // 发送请求参数 out.print(param); // flush输出流的缓冲 out.flush(); // 定义BufferedReader输入流来读取URL的响应 in = new BufferedReader( new InputStreamReader(conn.getInputStream())); String line; while ((line = in.readLine()) != null) { result += line; } } catch (Exception e) { System.out.println("发送 POST 请求出现异常!"+e); e.printStackTrace(); } //使用finally块来关闭输出流、输入流 finally{ try{ if(out!=null){ out.close(); } if(in!=null){ in.close(); } } catch(IOException ex){ ex.printStackTrace(); } } return result; } }
pom.xml的配置:要配置:rt.jar跟jce.jar都是在jdk/jre/lib里的,记得对应好路劲
<bootclasspath>${java.home}/jre/lib/rt.jar${path.separator}${java.home}/jre/lib/jce.jar</bootclasspath>
<build> <finalName>OneProject</finalName> <pluginManagement> <plugins> <plugin> <artifactId>maven-clean-plugin</artifactId> <version>3.0.0</version> </plugin> <plugin> <groupId>org.apache.maven.pluginsgroupId</groupId> <artifactId>maven-compiler-pluginartifactId</artifactId> <version>2.5.1version</version> <configuration> <source>1.7source</source> <target>1.7target</target> <compilerArguments> <verbose /> <bootclasspath>${java.home}/jre/lib/rt.jar${path.separator}${java.home}/jre/lib/jce.jar</bootclasspath> </compilerArguments> <encoding>utf-8encoding</encoding> </configuration> </plugin> <plugin> <artifactId>maven-resources-plugin</artifactId> <version>3.0.2</version> </plugin> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.7.0</version> </plugin> <plugin> <artifactId>maven-surefire-plugin</artifactId> <version>2.20.1</version> </plugin> <plugin> <artifactId>maven-war-plugin</artifactId> <version>3.2.0</version> </plugin> <plugin> <artifactId>maven-install-plugin</artifactId> <version>2.5.2</version> </plugin> <plugin> <artifactId>maven-deploy-plugin</artifactId> <version>2.8.2</version> </plugin> </plugins> </pluginManagement> <resources> <resource> <directory>src/main/java</directory> <includes> <include>**/*.xml</include> </includes> </resource> </resources> </build>
这里可能还会报406错误,主要是因为返回结果类型的问题
所以得在springmvc.xml中配置
<context:annotation-config /> <mvc:annotation-driven />
这样就可以了,然后我们去小程序控制台看返回结果
这样就大功告成了!