文章目录
前言
一. scope的类型总览
-
设置依赖范围
- 可以对引入的资源进行设置,设置其生效的范围。生效范围主要有以下:
-
主代码是否有效
- 测试代码是否有效
- 是否参与打包
- 而上述三种不同范围的组合,便有了下标四个<scope>对应的值
二. 详解
1.compile(默认选项)
表示为当前依赖参与项目的编译、测试和运行阶段,scope的默认选项。打包之时,会打到包里去。
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
</dependency>
2. test
表示为当前依赖只参与测试阶段,打包之时,不会打到包里
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
3. runtime
表示为当前依赖只参与运行阶段,一般这种类库都是接口与实现相分离的类库,如mysql的驱动包
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
只有编译会使用,程序运行时不需要依赖的。
案例一:lombok 只在编译时,把 getter、setter 设置好,不需要运行时使用。
案例二:servlet-api 只在编译时,需要引入,运行时由 tomcat 容器提供。
4.privided
表示为当前依赖在打包过程中,不需要打进去,需要由运行的环境来提供,比如springboot打war包,使用外部tomcat启动,而不是使用内置的tomcat。区别在于打包阶段进行了exclude操作。
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
5. system
表示为当前依赖不从maven仓库获取,从本地系统获取,结合systempath使用,常用于无法从maven仓库获取的包。
<dependency>
<groupId>com.supermap</groupId>
<artifactId>data</artifactId>
<version>1.0</version>
<scope>system</scope>
<systemPath>
<systemPath>${project.basedir}/lib/projectJarTest-0.0.1-SNAPSHOT.jar</systemPath>
</systemPath>
</dependency>
6. import
这个是maven2.0.9版本后出的属性,import只能在dependencyManagement的中使用,能解决maven单继承问题,import依赖关系实际上并不参与限制依赖关系的传递性。
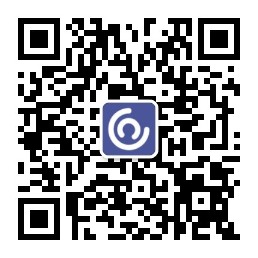
表示为当前项目依赖为多继承关系,常用于项目中自定义父工程,需要注意的是只能用在dependencyManagement里面,且仅用于type=pom的dependency。
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>${spring-boot.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
例:
如spring-boot-dependencies下的依赖可以直接引入,比如下面这些:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
<version>2.0.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
<version>2.0.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-ldap</artifactId>
<version>2.0.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
<version>2.0.4.RELEASE</version>
</dependency>
直接引入,不需要指定版本号:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
但是spring-cloud-dependencies下的依赖不能直接引入,比如下面这些:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-gateway-dependencies</artifactId>
<version>${spring-cloud-gateway.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-consul-dependencies</artifactId>
<version>${spring-cloud-consul.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-sleuth-dependencies</artifactId>
<version>${spring-cloud-sleuth.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-vault-dependencies</artifactId>
<version>${spring-cloud-vault.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
不能直接引入,如:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-gateway-dependencies</artifactId>
</dependency>
因为它里面的依赖都含有
<type>pom</type>
<scope>import</scope>
而是需要看spring-cloud-gateway-dependencies的中的包,才能直接引入
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
<version>${project.version}</version>
</dependency>
三.optional
optional 为 true 的 话 作 用 是 让 新 项 目 依 赖 本 项 目 的 时 候 , 不 去 依 赖 此 jar包,设 置 false或者不设值采用默认, 新 项 目 就 会 能 引 用 到 此 jar 包 compile,缺省值,适用于所有阶段,会随着项目一起发布。 编译范围依赖在所有的classpath 中可用,同时它们也会被打包
<dependency>
<groupId>org.springframework.kafka</groupId>
<artifactId>spring-kafka</artifactId>
<optional>true</optional>
<!-- 简单说明下:
这是关键:optional 为 true 的 话 作 用 是 让 新 项 目 依 赖 本 项 目 的 时 候 , 不 去 依 赖 此 jar包,设 置 false或者不设值采用默认, 新 项 目 就 会 能 引 用 到 此 jar 包
compile,缺省值,适用于所有阶段,会随着项目一起发布。 编译范围依赖在所有的classpath 中可用,同时它们也会被打包
-->
</dependency>