AppIntro的使用
- AppIntro的依赖(添加到gradle依赖项中):
repositories {
maven { url "https://jitpack.io" }
}
dependencies {
// AndroidX Capable version
implementation 'com.github.AppIntro:AppIntro:6.1.0'
// *** OR ***
// Latest version compatible with the old Support Library
implementation 'com.github.AppIntro:AppIntro:4.2.3'
}
- 创建一个类来使用Activity来继承AppIntro(或继承AppIntro2,展示幻灯片效果不同),并且重写其中的三个方法onCreate()、 onSkipPressed()、onDonePressed()
- 在onCreate中使用addSlide()方法来进行展示欢迎界面的图片
- 在manifests清单文件中进行声明
- 如下所示:
AppIntroActivity.java
package com.it.appintro;
import android.os.Bundle;
import androidx.fragment.app.Fragment;
import com.github.appintro.AppIntro;
import com.github.appintro.AppIntroFragment;
import org.jetbrains.annotations.Nullable;
public class AppIntroActivity extends AppIntro {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/*
* AppIntroFragment.newInstance()有多个重载方法,这里使用的是AppIntroFragment.newInstance(CharSequence title,CharSequence
* description,int imageDrawable)
*
* */
addSlide(AppIntroFragment.newInstance("Welcome!",
"This is a demo example in java of AppIntro library, with a custom background on each slide!",
R.drawable.img1));
addSlide(AppIntroFragment.newInstance(
"Clean App Intros",
"This library offers developers the ability to add clean app intros at the start of their apps.",
R.drawable.img2
));
}
@Override
protected void onSkipPressed(@Nullable Fragment currentFragment) {
super.onSkipPressed(currentFragment);
//finish直接返回上一层
finish();
}
@Override
protected void onDonePressed(@Nullable Fragment currentFragment) {
super.onDonePressed(currentFragment);
//finish直接返回上一层
finish();
}
}
manifests清单文件
<activity android:name=".AppIntroActivity"
android:label="AppIntroActivity"/>
运行效果如下所示:
共有两页幻灯片,左下角和右下角有跳转按钮和返回按钮(分别对应着onSkipPress方法和onDonePress方法),由于背景颜色的原因让我们无法看见(背景颜色和按钮颜色均可进行设置),但进行点击还是会进行执行我们预先定义好的finish()方法
注意点:
- 不能在onCreate的方法中使用setContentView()方法,AppIntro的超类将自动处理
- onCreate()方法有多个重载方法,我们需要使用的是只有一个Bundle参数的onCreate方法
- 建议不要将 MyCustomAppIntro 声明为你的第一个活动,除非你希望每次你的应用程序启动时都启动 MyCustomAppIntro。理想情况下,应该只向用户显示一次 AppIntro 活动,并且完成后应该隐藏它(可以在 SharedPreferences 中使用标志)。
AppIntroFragment.newInstance()的重载方法学习
我们知道,addSlide的方法调用需要传递一个Fragment参数,我们平常使用AppIntroFragment.newInstance()的方式来获取Fragment类型的对象,显而易见,AppIntroFragment.newInstance()使用的是单例模式,并且该方法存有多个重载方法,但是其中蕴含一定的规律,参数多的方法与参数少的方法进行相比,参数多的方法中会包含参数低的方法中的所有参数,所以我们只需要学习最多参数的AppIntroFragment.newInstance()方法即可掌握大多数的重载方法,而参数最多的形式为:
addSlide(AppIntroFragment.newInstance(
title = "The title of your slide",
description = "A description that will be shown on the bottom",
imageDrawable = R.drawable.the_central_icon,
backgroundDrawable = R.drawable.the_background_image,
titleColor = Color.YELLOW,
descriptionColor = Color.RED,
backgroundColor = Color.BLUE,
titleTypefaceFontRes = R.font.opensans_regular,
descriptionTypefaceFontRes = R.font.opensans_regular,
))
我们来对AppIntroActivity.java文件进行修改:
package com.it.appintro;
import android.annotation.SuppressLint;
import android.graphics.Color;
import android.os.Bundle;
import androidx.fragment.app.Fragment;
import com.github.appintro.AppIntro;
import com.github.appintro.AppIntroFragment;
import org.jetbrains.annotations.Nullable;
public class AppIntroActivity extends AppIntro {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/*
* AppIntroFragment.newInstance()有多个重载方法,这里使用的是AppIntroFragment.newInstance(CharSequence title,CharSequence
* description,int imageDrawable)
*
* */
AppIntroFragment.newInstance();
addSlide(AppIntroFragment.newInstance("Welcome!",
"This is a demo example in java of AppIntro library, with a custom background on each slide!",
R.drawable.img1, Color.GRAY,Color.RED,Color.BLACK)); //最后两个参数分别是标题的字体和讲述的字体,这里不再赘述
addSlide(AppIntroFragment.newInstance(
"Clean App Intros",
"This library offers developers the ability to add clean app intros at the start of their apps.",
R.drawable.img2,Color.GRAY,Color.RED,Color.BLACK
));
}
@Override
protected void onSkipPressed(@Nullable Fragment currentFragment) {
super.onSkipPressed(currentFragment);
//finish直接返回上一层
finish();
}
@Override
protected void onDonePressed(@Nullable Fragment currentFragment) {
super.onDonePressed(currentFragment);
//finish直接返回上一层
finish();
}
}
运行效果如下所示:
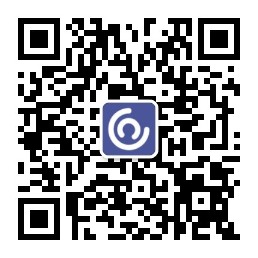
其中AppIntroFragment.newInstance()还存有一个及其特殊的重载方法,那就是通过自定义布局文件来进行展示自定义的幻灯片,代码如下所示:
custom_activity.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
android:orientation="vertical"
android:background="@drawable/img1"
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="25sp"
android:gravity="center"
android:textColor="@color/black"
android:layout_marginTop="20dp"
android:text="这是自定义的"
/>
</LinearLayout>
//添加自定义的幻灯片
addSlide(AppIntroCustomLayoutFragment.newInstance(R.layout.custom_activity));
通过Slider来进行添加幻灯片
我们也可以不采用链式编程的想法,通过手动进行设置Slider来进行设置幻灯片:
//通过SliderPage来添加幻灯片
SliderPage sliderPage = new SliderPage();
sliderPage.setTitle("这是SliderPage");
sliderPage.setDescription("这是自定义的SliderPage");
sliderPage.setImageDrawable(R.drawable.img2);
sliderPage.setBackgroundColor(Color.GRAY);
sliderPage.setTitleColor(Color.BLUE);
sliderPage.setDescriptionColor(Color.RED);
addSlide(AppIntroFragment.newInstance(sliderPage));
AppIntro的多种配置
基本入门AppIntro之后的使用就是采取多种配置来找到自己喜爱的风格,有淡出,过渡等等,具体配置请查看官文文档,在此附上官方文档地址:
https://github.com/AppIntro/AppIntro
最后附上官方文档中的AppIntro例子(其中的具体注释已经汉化,可以进行学习一下AppIntro的各种配置):
package com.github.appintro.example.ui.java;
import android.os.Bundle;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
import com.github.appintro.AppIntro;
import com.github.appintro.AppIntroFragment;
import com.github.appintro.AppIntroPageTransformerType;
import com.github.appintro.example.R;
public class JavaIntro extends AppIntro {
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
addSlide(AppIntroFragment.newInstance("Welcome!",
"This is a demo example in java of AppIntro library, with a custom background on each slide!",
R.drawable.ic_slide1));
addSlide(AppIntroFragment.newInstance(
"Clean App Intros",
"This library offers developers the ability to add clean app intros at the start of their apps.",
R.drawable.ic_slide2
));
addSlide(AppIntroFragment.newInstance(
"Simple, yet Customizable",
"The library offers a lot of customization, while keeping it simple for those that like simple.",
R.drawable.ic_slide3
));
addSlide(AppIntroFragment.newInstance(
"Explore",
"Feel free to explore the rest of the library demo!",
R.drawable.ic_slide4
));
// 淡出过渡
setTransformer(AppIntroPageTransformerType.Fade.INSTANCE);
// 显示/隐藏状态栏
showStatusBar(true);
//加快或减慢滚动速度
setScrollDurationFactor(2);
//在两个幻灯片之间启用颜色“淡出”动画(确保幻灯片实现)
setColorTransitionsEnabled(true);
//防止后退按钮退出幻灯片
setSystemBackButtonLocked(true);
//激活向导模式(一些美学上的改变)
setWizardMode(true);
// 显示/隐藏跳过按钮
setSkipButtonEnabled(true);
//启用沉浸模式(无状态和导航条)
setImmersiveMode();
// 启用/禁用页面指示符
setIndicatorEnabled(true);
//展示/隐藏所有按钮
setButtonsEnabled(true);
}
@Override
protected void onSkipPressed(Fragment currentFragment) {
super.onSkipPressed(currentFragment);
finish();
}
@Override
protected void onDonePressed(Fragment currentFragment) {
super.onDonePressed(currentFragment);
finish();
}
}