JavaScript的继承方式有多种,这里列举3种,分别是原型继承、类继承以及混合继承。
1、原型继承
优点:既继承了父类的模板,又继承了父类的原型对象;
缺点:不是子类实例传参,而是需要通过父类实例,不符合常规。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>javascript的3种继承方式</title> </head> <body> <script type="text/javascript"> //1、原型继承 //父类(水果) function Fruit(name, price) { this.name = name; this.price = price; } //父类的原型对象属性 Fruit.prototype.id = 2018; //子类(苹果) function Apple(color) { this.color = color; } //继承实现 Apple.prototype = new Fruit('apple', 15); var apple = new Apple('red'); console.log(apple.name); console.log(apple.price); console.log(apple.color); console.log(apple.id); </script> </body> </html>
控制台结果:
2、类继承(运用构造函数的方式继承)
优点:继承了父类的模板并且能通过子类实例传参;
缺点:不能继承父类的原型对象。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>javascript的3种继承方式</title> </head> <body> <script type="text/javascript"> //2、类继承(使用构造函数的方式继承) //父类(水果) function Fruit(name, price) { this.name = name; this.price = price; } //父类的原型对象属性 Fruit.prototype.id = 99; //子类(苹果) function Apple(name, price, color) { Fruit.call(this, name, price);//调用call或apply方法实现继承 this.color = color; } var apple = new Apple('apple', 12, 'red'); console.log(apple.name); console.log(apple.price); console.log(apple.color); console.log(apple.id); </script> </body> </html>
控制台结果:
扫描二维码关注公众号,回复:
1572988 查看本文章
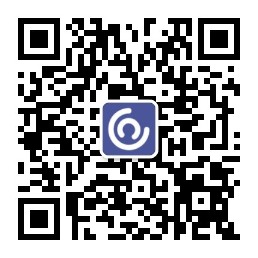
3、混合继承(原型继承+类继承)
优点:既继承了父类的模板,又继承了父类的原型对象,还能实现子类实例的传参。
缺点:Apple.prototype = new Fruit();这步又实例化了一次,当多次调用比较占用资源,影响性能。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>javascript的3种继承方式</title> </head> <body> <script type="text/javascript"> //3、组合继承 //父类(苹果) function Fruit(name, price) { this.name = name; this.price = price; } //父类的原型对象属性 Fruit.prototype.id = 98; //子类(苹果) function Apple(name, price, color) { Fruit.call(this, name, price);//调用call或apply方法实现继承 this.color = color; } //原型继承 Apple.prototype = new Fruit(); var apple = new Apple('apple', 12, 'red'); console.log(apple.name); console.log(apple.price); console.log(apple.color); console.log(apple.id); </script> </body> </html>
控制台结果: