1、ES6之前,继承都用构造函数来实现;
对象的继承,先申明一个对象,里面添加实例成员
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title></title>
</head>
<body>
<script>
// 1、实例成员 就是构造函数内部通过this添加的成员,uname age sing 都是实例成员,只能通过实例化的对象来访问
function Weber(uname, age) {
this.uname = uname;
this.age = age;
this.write = function() {
console.log("----------write--" + this.uname);
}
}
var qyz = new Weber("青阳子", 36);
qyz.write();
</script>
</body>
</html>
构造函数的调用需要用new操作符,而普通函数的调用又分很多种,但是都不会用到new操作符。所以,构造函数和普通函数的区别就在这个new操作符里,现在让我们来好好研究一下这个new操作符。
用new操作符创建对象时发生的事情:
第一步: 创建一个Object对象实例。
第二步: 将构造函数的执行对象赋给新生成的这个实例。
第三步: 执行构造函数中的代码
第四步: 返回新生成的对象实例
注意:原本的构造函数是window对象的方法,如果不用new操作符而直接调用,那么构造函数的执行对象就 是window,即this指向了window。现在用new操作符后,this就指向了新生成的对象。理解这一步至关重要。
实例成员
通过构造函数创建的对象称为实例对象,实例对象中的属性和方法称为实例成员。
// 1.实例成员就是构造函数内部通过this添加的成员 uname age sing 就是实例成员
// 实例成员只能通过实例化的对象来访问
2、静态成员,就是在构造函数本身上添加的成员
Weber.sex = "男";
console.log(Weber.sex) //男
console.log(qyz.sex) //undefined
3、构造函数存在的问题:浪费内存
var qyz = new Weber("青阳子", 36);
var lee = new Weber("李博涵", 4);
console.log(qyz.write === lee.write); //false 比较的是内存地址,不一致
//所有对象应该公用一套函数,以节省空间
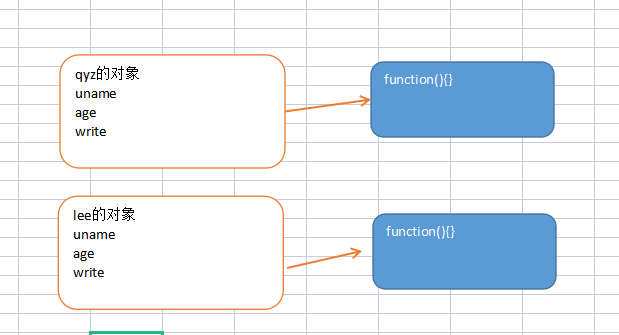
1.构造函数原型 prototype
构造函数通过原型分配的函数是所有对象所共享的。
JavaScript 规定,每一个构造函数都有一个 prototype 属性,指向另一个对象。注意这个 prototype 就是一个对象,这个对象的所有属性和方法,都会被构造函数所拥有。
我们可以把那些不变的方法,直接定义在 prototype 对象上,这样所有对象的实例就可以共享这些方法。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<script>
// 1. 构造函数的问题.
function Weber(uname, age) {
this.uname = uname;
this.age = age;
// this.write = function() {
// console.log('我会写博客');
// }
}
Weber.prototype.write = function() {
console.log('我会写博客');
}
var qyz = new Weber('青阳子', 38);
var lee = new Weber('李博涵', 4);
console.log(qyz.write === lee.write);
// console.dir(Weber);
qyz.write();
lee.write();
// 2. 一般情况下,我们的公共属性定义到构造函数里面, 公共的方法我们放到原型对象身上
</script>
</body>
</html>
一个对象,我们也称prototype 为原型对象, 原型的作用是:共享方法
2.对象原型 __proto__
对象都会有一个属性 __proto__ 指向构造函数的 prototype 原型对象,之所以我们对象可以使用构造函数 prototype 原型对象的属性和方法,就是因为对象有 __proto__ 原型的存在。
对象原型__proto__
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<script>
function Weber(uname, age) {
this.uname = uname;
this.age = age;
}
Weber.prototype.write = function() {
console.log('我会唱歌');
}
var qyz = new Weber('青阳子', 36);
var lee = new Weber('李博涵', 4);
qyz.write();
console.log(qyz); // 对象身上系统自己添加一个 __proto__ 指向我们构造函数的原型对象 prototype
console.log(qyz.__proto__ === Weber.prototype);
// 方法的查找规则: 首先先看qyz 对象身上是否有 write 方法,如果有就执行这个对象上的write
// 如果么有write 这个方法,因为有__proto__ 的存在,就去构造函数原型对象prototype身上去查找write这个方法
</script>
</body>
</html>
__proto__对象原型和原型对象 prototype 是等价的
__proto__对象原型的意义就在于为对象的查找机制提供一个方向,或者说一条路线,但是它是一个非标准属性, 因此实际开发中,不可以使用这个属性,它只是内部指向原型对象 prototype
3. constructor 构造函数
对象原型( __proto__)和构造函数(prototype)原型对象里面都有一个属性 constructor 属性 ,constructor 我们称为构造函数,因为它指回构造函数本身。
constructor 主要用于记录该对象引用于哪个构造函数,它可以让原型对象重新指向原来的构造函数。
一般情况下,对象的方法都在构造函数的原型对象中设置。如果有多个对象的方法,我们可以给原型对象采取对象形式赋值,但是这样就会覆盖构造函数原型对象原来的内容,这样修改后的原型对象 constructor 就不再指向当前构造函数了。
此时,我们可以在修改后的原型对象中,添加一个 constructor 指向原来的构造函数
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<script>
function Weber(uname, age) {
this.uname = uname;
this.age = age;
}
Weber.prototype.write = function() {
console.log('我会写博客');
}
var qyz = new Weber('青阳子', 36);
// 1. 只要是对象就有__proto__ 原型, 指向原型对象
console.log(Weber.prototype);
console.log(Weber.prototype.__proto__ === Object.prototype);
// 2.我们Weber原型对象里面的__proto__原型指向的是 Object.prototype
console.log(Object.prototype.__proto__);
// 3. 我们Object.prototype原型对象里面的__proto__原型 指向为 null
</script>
</body>
</html>
4.JavaScript 的成员查找机制(规则)
① 当访问一个对象的属性(包括方法)时,首先查找这个对象自身有没有该属性。
② 如果没有就查找它的原型(也就是 __proto__指向的 prototype 原型对象)。
③ 如果还没有就查找原型对象的原型(Object的原型对象)。
④ 依此类推一直找到 Object 为止(null)。
⑤ __proto__对象原型的意义就在于为对象成员查找机制提供一个方向,或者说一条路线。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<script>
function Weber(uname, age) {
this.uname = uname;
this.age = age;
}
Weber.prototype.write = function() {
console.log('我会写博客');
}
Weber.prototype.sex = '女';
// Object.prototype.sex = '男';
var qyz = new Weber('青阳子', 36);
qyz.sex = '男';
console.log(qyz.sex);
console.log(Object.prototype);
console.log(qyz);
console.log(Weber.prototype);
console.log(qyz.toString());
</script>
</body>
</html>
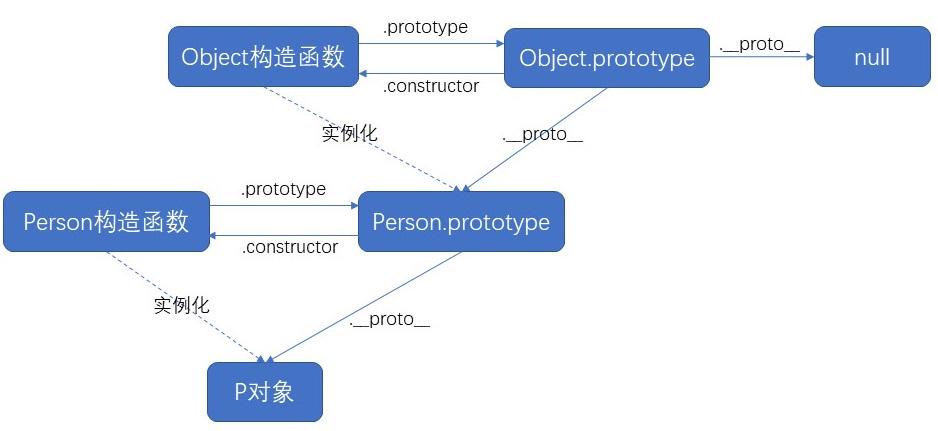
5. 原型对象this指向
构造函数中的this 指向我们实例对象.
原型对象里面放的是方法, 这个方法里面的this 指向的是 这个方法的调用者, 也就是这个实例对象.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<script>
function Weber(uname, age) {
this.uname = uname;
this.age = age;
}
var that;
Weber.prototype.write = function() {
console.log('我会写博客');
that = this;
}
var qyz = new Weber('青阳子', 36);
// 1. 在构造函数中,里面this指向的是对象实例 qyz
qyz.write();
console.log(that === qyz);
// 2.原型对象函数里面的this 指向的是 实例对象 qyz
</script>
</body>
</html>