文章目录
1. 需求分析
1.1 功能分析
① 登录界面实现用户的登录、注册、修改密码以及注销用户的功能,登录信息包括用户的账号以及密码,登录成功后跳转到生日界面。
② 生日界面实现个人生日信息的录入、查询以及删除功能,录入信息包括个人姓名以及生日日期。
1.2 性能分析
① 系统易操作性
所开发的系统就做到操作简单,尽量使系统操作不受用户对电脑知识水平的限制。
② 系统具有可维护性
由于系统涉及的信息比较广,TXT中的数据需要定期修改,系统可利用的空间及性能也随之下降,为了使系统更好地运转。
2. 技术原理
本项目主要运用了 python 的函数、文件、tkinter 界面设计以及 pickle 模块等技术原理,其中:
① 生日界面的具体技术原理包括Tkinter GUI编程、pickle序列化、文件读写等。这些技术可以帮助开发者快速地构建出实用的桌面应用程序。
② 登录界面的具体技术原理包括pickle模块进行文件操作和序列化、Tkinter GUI编程实现用户界面、条件控制语句实现逻辑判断。
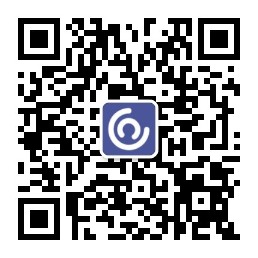
3. 详细设计
3.1 登录界面
① 程序设计
root=tk.Tk()
root.title('登录账户')
screenheight=root.winfo_screenheight()
screenwidth=root.winfo_screenwidth()
height=300
width=500
x=(screenwidth-width)//2
y=(screenheight-height)//2
root.geometry('%dx%d+%d+%d'%(width,height,x,y))
tk.Label(root,text='欢迎光临我的世界',font=('宋体',20),bg='white',fg='blue',width=20,height=2).pack()
tk.Label(root,text='账户',font=('宋体',12)).place(x=140,y=80)
tk.Label(root,text='密码',font=('宋体',12)).place(x=140,y=120)
name=tk.StringVar()
entry_name=tk.Entry(root,textvariable=name,font=('宋体',12),show=None)
entry_name.place(x=180,y=80)
key=tk.StringVar()
entry_key=tk.Entry(root,textvariable=key,font=('宋体',12),show=None)
entry_key.place(x=180,y=120)
login=tk.Button(root,text='登录账户',font=('宋体',12),bg='blue',fg='white',width=10,height=1,command=user_login)
login.place(x=150,y=180)
sign=tk.Button(root,text='注册账户',font=('宋体',12),bg='red',fg='white',width=10,height=1,command=user_sign)
sign.place(x=250,y=180)
change=tk.Button(root,text='修改密码',font=('宋体',12),bg='green',fg='white',width=10,height=1,command=user_change)
change.place(x=150,y=220)
deluser=tk.Button(root,text='注销账户',font=('宋体',12),bg='black',fg='white',width=10,height=1,command=user_del)
deluser.place(x=250,y=220)
root.mainloop()
② 程序分析
这段代码使用Tkinter库创建了一个用户登录界面,提供了登录、注册、修改密码、注销账户等操作。界面包含了标签、输入框、按钮等多种控件。通过使用Tkinter库提供的函数和方法,可以定位、布局、编辑和响应用户界面中的各种控件,实现用户交互功能。具体技术包括Tkinter GUI编程、事件响应机制、条件控制语句等。
3.2 注册界面
① 程序设计
def user_sign():
try:
with open('d:\\user.pickle','rb') as user_file:
user_info=pickle.load(user_file)
except FileNotFoundError:
with open('d:\\user.pickle','wb') as user_file:
user_info={
'admin':'12345'}
pickle.dump(user_info,user_file)
user_file.close()
sign_root=tk.Toplevel(root)
sign_root.title('注册账户')
screenheight=sign_root.winfo_screenheight()
screenwidth=sign_root.winfo_screenwidth()
h=220
w=300
x=(screenwidth-w)//2
y=(screenheight-h)//2
sign_root.geometry("%dx%d+%d+%d"%(w,h,x,y))
new_name=tk.StringVar()
tk.Label(sign_root,text='欢迎来到注册系统',font=('宋体',20),bg='white',fg='red',width=20,height=1).place(x=7,y=10)
tk.Label(sign_root,text='新的账户',font=('宋体',12)).place(x=20,y=60)
entry_name=tk.Entry(sign_root,textvariable=new_name,font=('宋体',12),show=None).place(x=100,y=60)
new_key=tk.StringVar()
tk.Label(sign_root,text='新的密码',font=('宋体',12)).place(x=20,y=100)
entry_key=tk.Entry(sign_root,textvariable=new_key,font=('宋体',12),show='*').place(x=100,y=100)
same_key=tk.StringVar()
tk.Label(sign_root,text='请确认密码',font=('宋体',12)).place(x=10,y=140)
entry_keys=tk.Entry(sign_root,textvariable=same_key,font=('宋体',12),show='*').place(x=100,y=140)
def sign_check():
name=new_name.get()
key1=new_key.get()
key2=same_key.get()
with open('d:\\user.pickle','rb') as user_file:
user_info=pickle.load(user_file)
user_file.close()
if name in user_info:
tkinter.messagebox.showerror('提示','该账户已存在!')
elif key1!=key2:
tkinter.messagebox.showerror('提示','两次输入密码不一致,请重新输入!')
else:
user_info[name]=key1
with open(r'd:\\user.pickle','wb') as user_file:
pickle.dump(user_info,user_file)
user_file.close()
with open(r'd:\\%s.pickle'%(name),'wb') as name_file:
dicts={
}
pickle.dump(dicts,name_file)
name_file.close()
tkinter.messagebox.showinfo('提示','注册成功!')
sign_root.destroy()
tk.Button(sign_root,text='注册',font=('宋体',12),bg='red',fg='white',width=5,height=1,command=sign_check).place(x=130,y=180)
② 程序分析
这段代码实现了用户注册的功能,使用了pickle模块读取和写入本地文件,并通过Tkinter创建了一个子窗口用来输入用户名和密码。当用户输入的用户名不在本地文件中时,程序会把新的用户名和密码添加到本地文件中。当用户输入的用户名已经存在时,程序会提示用户该账户已存在。当用户输入的密码不一致时,程序会提示用户两次输入密码不一致。具体技术原理包括pickle模块的读写处理、Tkinter库的子窗口创建及布局、按钮事件响应语句等。
3.3 修改密码
① 程序设计
def user_change():
try:
with open('d:\\user.pickle','rb') as user_file:
user_info=pickle.load(user_file)
except FileNotFoundError:
with open('d:\\user.pickle','wb') as user_file:
user_info={
'admin':'12345'}
pickle.dump(user_info,user_file)
user_file.close()
change_root=tk.Toplevel(root)
old_name=tk.StringVar()
old_key=tk.StringVar()
new_key=tk.StringVar()
same_key=tk.StringVar()
change_root.title('修改密码')
screenheight=change_root.winfo_screenheight()
screenwidth=change_root.winfo_screenwidth()
h=220
w=300
x=(screenwidth-w)//2
y=(screenheight-h)//2
change_root.geometry("%dx%d+%d+%d"%(w,h,x,y))
tk.Label(change_root,text='欢迎来到修改密码系统',font=('宋体',20),bg='white',fg='green',width=20,height=1).place(x=5,y=10)
tk.Label(change_root,text='请输入你的账号',font=('宋体',12),width=15,height=1).place(x=5,y=60)
tk.Entry(change_root,textvariable=old_name,show=None).place(x=130,y=60)
tk.Label(change_root,text='请输入原始密码',font=('宋体',12),width=15,height=1).place(x=5,y=90)
tk.Entry(change_root,textvariable=old_key,show=None).place(x=130,y=90)
tk.Label(change_root,text='请输入修改密码',font=('宋体',12),width=15,height=1).place(x=5,y=120)
tk.Entry(change_root,textvariable=new_key,show='*').place(x=130,y=120)
tk.Label(change_root,text='请确认你的密码',font=('宋体',12),width=15,height=1).place(x=5,y=150)
tk.Entry(change_root,textvariable=same_key,show='*').place(x=130,y=150)
def change_check():
name=old_name.get()
key1=old_key.get()
key2=new_key.get()
key3=same_key.get()
with open("d:\\user.pickle",'rb') as user_file:
user_info=pickle.load(user_file)
user_file.close()
if name in user_info:
if key1==user_info[name]:
if key2==key3:
user_info[name]=key2
with open('d:\\user.pickle','wb') as user_file:
pickle.dump(user_info,user_file)
user_file.close()
tkinter.messagebox.showinfo('提示',"修改成功!")
change_root.destroy()
else:
tkinter.messagebox.showerror('提示','两次密码不一致,请重新输入!')
else:
tkinter.messagebox.showerror('提示','密码错误,请重新输入!')
else:
exist=tkinter.messagebox.askyesno('提示','该账户不存在,是否立即注册账户?')
if exist:
user_sign()
tk.Button(change_root,text='修改',font=('宋体',12),bg='green',fg='white',command=change_check).place(x=130,y=180)
② 程序分析
这段代码实现了用户修改密码的功能,通过创建Tkinter库的子窗口实现用户输入信息,并使用pickle模块读取和写入本地文件进行存储。当用户输入的用户名和密码都正确时,程序会将新的密码替换原有的密码保存在本地文件中。当用户输入的用户名不存在时,程序会提示用户是否立即注册该账户。当用户输入的密码不一致时,程序会提示用户两次输入密码不一致。具体技术原理包括pickle模块的读写处理、Tkinter库的子窗口界面创建、条件判断语句等。
3.4 注销账户
① 程序设计
def user_del():
try:
with open('d:\\user.pickle','rb') as user_file:
user_info=pickle.load(user_file)
except FileNotFoundError:
with open('d:\\user.pickle','wb') as user_file:
user_info={
'admin':'12345'}
pickle.dump(user_info,user_file)
user_file.close()
old_name=tk.StringVar()
old_key=tk.StringVar()
same_key=tk.StringVar()
del_root=tk.Toplevel(root)
del_root.title('注销账户')
screenheight=del_root.winfo_screenheight()
screenwidth=del_root.winfo_screenwidth()
h=220
w=300
x=(screenwidth-w)//2
y=(screenheight-h)//2
del_root.geometry("%dx%d+%d+%d"%(w,h,x,y))
tk.Label(del_root,text='欢迎来到注销账户系统',font=('宋体',20),bg='white',fg='black',width=20,height=1).place(x=5,y=10)
tk.Label(del_root,text='请输入你的账号',font=('宋体',12),width=15,height=1).place(x=5,y=60)
tk.Entry(del_root,textvariable=old_name,show=None).place(x=130,y=60)
tk.Label(del_root,text='请输入原始密码',font=('宋体',12),width=15,height=1).place(x=5,y=100)
tk.Entry(del_root,textvariable=old_key,show=None).place(x=130,y=100)
tk.Label(del_root,text='请确认你的密码',font=('宋体',12),width=15,height=1).place(x=5,y=140)
tk.Entry(del_root,textvariable=same_key,show='*').place(x=130,y=140)
def del_check():
name=old_name.get()
key1=old_key.get()
key2=same_key.get()
with open('d:\\user.pickle','rb') as user_file:
user_info=pickle.load(user_file)
user_file.close()
if name in user_info:
if key1==key2:
if key1==user_info[name]:
user_info.pop(name)
with open('d:\\user.pickle','wb') as user_file:
pickle.dump(user_info,user_file)
user_file.close()
tkinter.messagebox.showinfo('提示','注销成功!')
else:
tkinter.messagebox.showerror('提示','密码错误!')
else:
tkinter.messagebox.showerror('提示','两次密码不一致!')
else:
exist=tkinter.messagebox.askyesno('提示','该账户不存在,是否立即注册?')
if exist:
user_sign()
tk.Button(del_root,text='注销',font=('宋体',12),bg='black',fg='white',command=del_check).place(x=130,y=180)
② 程序分析
这段代码实现了用户注销账户的功能,同样使用了Tkinter库的子窗口,通过pickle模块对本地文件进行读写存储。当用户输入的用户名和密码都正确时,程序会将该账户从本地文件中删除。当用户输入的用户名不存在时,程序会提示用户是否立即注册该账户。当用户输入的密码不一致时,程序会提示用户两次输入密码不一致的错误信息。具体技术原理包括pickle模块的读写处理、Tkinter库的子窗口界面创建、条件判断语句等。
3.5 生日界面
① 程序设计
def birth():
birthday=tk.Toplevel(root)
birthday.title('欢迎光临')
screenheight=root.winfo_screenheight()
screenwidth=root.winfo_screenwidth()
height=300
width=500
x=(screenwidth-width)//2
y=(screenheight-height)//2
birthday.geometry('%dx%d+%d+%d'%(width,height,x,y))
var1=tk.StringVar()
var2=tk.StringVar()
var3=tk.StringVar()
var4=tk.StringVar()
tk.Label(birthday,text='欢迎%s进入系统'%(user_name),font=('宋体',12),bg='blue',fg='white',width=20,height=3).place(x=160,y=20)
tk.Label(birthday,text='姓名',font=('宋体',12),bg='white',fg='black').place(x=20,y=100)
tk.Label(birthday,text='生日',font=('宋体',12),bg='white',fg='black').place(x=240,y=100)
tk.Label(birthday,text='姓名',font=('宋体',12),bg='white',fg='black').place(x=20,y=170)
tk.Label(birthday,text='生日',font=('宋体',12),bg='white',fg='black').place(x=240,y=170)
tk.Entry(birthday,textvariable=var1).place(x=80,y=100)
tk.Entry(birthday,textvariable=var2).place(x=300,y=100)
tk.Entry(birthday,textvariable=var3).place(x=80,y=170)
tk.Entry(birthday,textvariable=var4).place(x=150,y=240)
def write():
key=var1.get()
value=var2.get()
with open('d:\\%s.pickle'%(user_name),'rb') as file:
dicts=pickle.load(file)
dicts[key]=value
with open('d:\\%s.pickle'%(user_name),'wb') as file:
pickle.dump(dicts,file)
file.close()
def read():
name=var3.get()
with open('d:\\%s.pickle'%(user_name),'rb') as file:
dicts=pickle.load(file)
file.close()
if name in dicts and dicts[name]!='':
re=dicts[name]
else:
re='ERROR!'
t.delete(1.0,tk.END)
t.insert('end',re)
def delete():
name=var4.get()
with open('d:\\%s.pickle'%(user_name),'rb') as file:
dicts=pickle.load(file)
file.close()
if name in dicts:
del name
tk.messagebox.showinfo("提示","删除成功!")
else:
tk.messagebox.showerror("警告","删除失败!")
pass
with open('d:\\%s.pickle'%(user_name),'wb') as file:
pickle.dump(dicts,file)
file.close()
t=tk.Text(birthday,font=('宋体',12),bg='white',fg='black',width=20,height=1)
t.place(x=290,y=170)
tk.Button(birthday,text='录入',font=('宋体',12),bg='red',fg='white',command=write).place(x=220,y=130)
tk.Button(birthday,text='查询',font=('宋体',12),bg='yellow',fg='white',command=read).place(x=220,y=200)
tk.Button(birthday,text='删除',font=('宋体',12),bg='black',fg='white',command=delete).place(x=300,y=235)
birthday.mainloop()
② 程序分析
这段代码实现了一个记录用户生日的小功能,通过Tkinter库创建一个子窗口,用户可以输入姓名和生日,并将其保存在本地pickle文件中。程序还提供了查询和删除用户生日的功能。具体技术原理包括Tkinter库的子窗口创建、布局和事件响应,pickle模块的读写处理等。