动态sql——if标签
以查询Product表为例:
Mybatis详细教程,详细可点这里
1.Product.xml配置文件
使用模糊查询的时候可能会用到不同的字段,如果查询一次使用一条sql语句,会变得难以维护,就能使用Mybatis的动态sql—-if标签
;如果没有传入参数那么就是查询所有,这样就可以一条语句应付多种情况。
<mapper namespace="com.bean">
<select id="listProductByName" resultType="Product">
select * from product_
<if test="name!=null">
where name like concat('%',#{name},'%')
</if>
</select>
</mapper>
2.test
// 查询Product使用模糊查询
private static void listProductByName(SqlSession session) {
Map<String, Object> params = new HashMap<>();
params.put("name", "b");//"b"通过动态输入灵活查询
List<Product> ps = session.selectList("listProductByName", params);
for (Product product : ps) {
System.out.println(product);
}
}
动态sql——where标签
1.修改Product.xml配置文件
当出现多条件查询的时候不能愉快的直接使用
select * from product_ where name like concat(‘%’,#{name},’%’) and price > #{price}会出现name为空price不为空的语句错误;这时候就使用where标签。
如果任何条件都不成立,那么就在sql语句里就不会出现where关键字
如果有任何条件成立,会自动去掉多出来的 and 或者 or。
<select id="listProductByName" resultType="Product">
select * from product_
<where>
<if test="name!=null">
and name like concat('%',#{name},'%')
</if>
<if test="price!=null and price!=0">
and price > #{price}
</if>
</where>
</select>
2.test
// 多条件查询
private static void listProductByNameAndPrice(SqlSession session) {
Map<String, Object> params = new HashMap<>();
params.put("name", "b");// 这时params值为不为空都可以正常运行
params.put("price", "2");
List<Product> ps = session.selectList("listProductByName", params);
for (Product product : ps) {
System.out.println(product);
}
}
动态sql——set标签
1.修改Product.xml配置文件
与where标签类似的,在update语句里也会碰到多个字段相关的问题。 在这种情况下,就可以使用set标签:
<update id="updateProduct" parameterType="Product">
update product_
<set>
<if test="name!=null">name=#{name},</if>
<if test="price!=null">price=#{price}</if>
</set>
where id=#{id}
</update>
2.test
// 修改Product
private static void updateProductById(SqlSession session) {
Product p=new Product(5,"product zz",9.99f);
session.update("updateProduct", p);
}
动态sql——trim标签
trim 用来定制想要的功能,比如where标签就可以用
<trim prefix="WHERE" prefixOverrides="AND|OR">
</trim>
替换
set标签就可以用
<trim prefix="SET" suffixOverrides=",">
</trim>
替换。
<select id="listProductByName" resultType="Product">
select * from product_
<trim prefix="WHERE" prefixOverrides="AND|OR"><!-- =where -->
<if test="name!=null">
and name like concat('%',#{name},'%')
</if>
<if test="price!=null and price!=0">
and price > #{price}
</if>
</trim>
</select>
<update id="updateProduct" parameterType="Product">
update product_
<trim prefix="SET" suffixOverrides=","><!-- set -->
<if test="name!=null">name=#{name},</if>
<if test="price!=null">price=#{price}</if>
</trim>
where id=#{id}
</update>
效果和where和set一样。
动态sql——choose标签
1.修改Product.xml配置文件
扫描二维码关注公众号,回复:
1565419 查看本文章
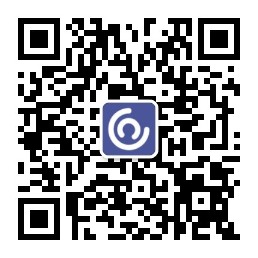
<!-- Mybatis里面没有else标签,但是可以使用when otherwise标签来达到这样的效果。 -->
<select id="productList" resultType="Product">
select * from product_
<where>
<choose>
<when test="name!=null">and name like concat('%',#{name},'%')</when>
<when test="price!=null and price!=0">and price > #{price}</when>
<otherwise>
and id>1
</otherwise>
<!-- 起作用是: 提供了任何条件,就进行条件查询,否则就使用id>1这个条件。 -->
</choose>
</where>
</select>
2.test
private static void productList(SqlSession session) {
Map<String, Object> params = new HashMap<>();
List<Product> ps = session.selectList("productList", params);
for (Product product : ps) {
System.out.println(product);
}
}
动态sql——foreach标签
1.修改Product.xml配置文件
<!-- foreach标签通常用于in 这样的语法里,比如查询id是1,2,3的数据 -->
<select id="listProductForeach" resultType="Product">
SELECT * FROM product_
WHERE ID in
<foreach item="item" index="index" collection="list" open="("
separator="," close=")">
#{item}
</foreach>
</select>
2.test
private static void productListForeach(SqlSession session) {
List<Integer> ids = new ArrayList<>();
ids.add(1);
ids.add(2);
ids.add(3);
List<Product> ps = session.selectList("listProductForeach", ids);
for (Product product : ps) {
System.out.println(product);
}
}
动态sql——bind标签
1.修改Product.xml配置文件
<!-- bind标签就像是再做一次字符串拼接,方便后续使用 -->
<!-- 如下,在模糊查询的基础上,把模糊查询改为bind标签。 -->
<select id="listProductBind" resultType="Product">
<bind name="likename" value="'%'+name+'%'" />
select * from product_ where name like #{likename}
</select>
2.test
private static void listProductBind(SqlSession session) {
Map<String, String> params = new HashMap<>();
params.put("name", "product");
List<Product> ps = session.selectList("listProductBind", params);
for (Product product : ps) {
System.out.println(product);
}
}