目录
扫描二维码关注公众号,回复:
15610076 查看本文章
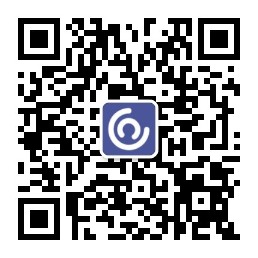
1. 添加依赖
<!--mybatis-plus-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.2</version>
</dependency>
<!--mybatis-plus自动生成代码依赖-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
<version>3.5.2</version>
</dependency>
<!--mybatis-plus模板-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
<version>2.7.4</version>
</dependency>
<!--jdbc-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<!--web-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--mysql-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!--lombok-->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<!--thymeleaf-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!--shiro-->
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-spring-boot-web-starter</artifactId>
<version>1.9.0</version>
</dependency>
2. 建库建表
CREATE DATABASE IF NOT EXISTS `shirodb` CHARACTER SET utf8mb4;
USE `shirodb`;
CREATE TABLE `user` (
`id` BIGINT(20) NOT NULL AUTO_INCREMENT COMMENT '编号',
`name` VARCHAR(30) DEFAULT NULL COMMENT '用户名',
`pwd` VARCHAR(50) DEFAULT NULL COMMENT '密码',
`rid` BIGINT(20) DEFAULT NULL COMMENT '角色编号',
PRIMARY KEY (`id`)
) ENGINE=INNODB AUTO_INCREMENT=2 DEFAULT CHARSET=utf8 COMMENT='用户表';
3. 手动增加数据测试需要
- 先生成加密后的密码添加到数据库里 方便测试使用
package com.jmh.springbootshiro.utls;
import org.apache.shiro.crypto.hash.Md5Hash;
public class ShiroMD5 {
public static void main(String[] args) {
String password = "1234";
//为了保证安全,避免被破解还可以多次迭代加密,保证数据安全
Md5Hash md53 = new Md5Hash(password,"salt",3);
System.out.println("md5带盐的3次加密:"+md53.toHex());
}
}
- 演示
4. 生产代码
- 生成输出目录注意查看、修改
package com.jmh.springbootshiro.generator;
import com.baomidou.mybatisplus.generator.FastAutoGenerator;
import com.baomidou.mybatisplus.generator.config.OutputFile;
import com.baomidou.mybatisplus.generator.engine.FreemarkerTemplateEngine;
import java.util.Collections;
/**
* @author 蒋明辉
* @data 2022/9/28 19:48
*/
public class MybatisPlusGenerator {
public static void main(String[] args) {
FastAutoGenerator.create(
"jdbc:mysql://localhost:3306/shirodb?useUnicode=true&characterEncoding=UTF-8&useSSL=false",
"root",
"1234")
.globalConfig(builder -> {
builder.author("jmh") // 设置作者
//.enableSwagger() // 开启 swagger 模式
.fileOverride() // 覆盖已生成文件
.outputDir("E:\\shiroProject\\springboot-shiro\\src\\main\\java"); // 指定输出目录
})
.packageConfig(builder -> {
builder.parent("com.jmh.springbootshiro") // 设置父包名
//.moduleName("system") // 设置父包模块名
.pathInfo(Collections.singletonMap(OutputFile.xml, "E:\\shiroProject\\springboot-shiro\\src\\main\\resources\\mapper")); // 设置mapperXml生成路径
})
.strategyConfig(builder -> {
builder.addInclude("user") // 设置需要生成的表名
.addTablePrefix("t_", "c_"); // 设置过滤表前缀
})
.templateEngine(new FreemarkerTemplateEngine()) // 使用Freemarker引擎模板,默认的是Velocity引擎模板
.execute();
}
}
- 自动生成目录结构
5. service模块
5.1 IUserService
package com.jmh.springbootshiro.service;
import com.jmh.springbootshiro.entity.User;
import com.baomidou.mybatisplus.extension.service.IService;
/**
* <p>
* 用户表 服务类
* </p>
*
* @author jmh
* @since 2022-11-13
*/
public interface IUserService extends IService<User> {
/**
* 根据用户名查询信息
*/
User queryUserInfoByName(String name);
}
5.2 UserServiceImpl
package com.jmh.springbootshiro.service.impl;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.jmh.springbootshiro.entity.User;
import com.jmh.springbootshiro.mapper.UserMapper;
import com.jmh.springbootshiro.service.IUserService;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
/**
* <p>
* 用户表 服务实现类
* </p>
*
* @author jmh
* @since 2022-11-13
*/
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements IUserService {
@Autowired
private UserMapper userMapper;
@Override
public User queryUserInfoByName(String name) {
QueryWrapper<User> queryWrapper=new QueryWrapper<>();
queryWrapper.eq("name",name);
return userMapper.selectOne(queryWrapper);
}
}
6. MyRealm
package com.jmh.springbootshiro.utls;
import com.jmh.springbootshiro.entity.User;
import com.jmh.springbootshiro.service.IUserService;
import org.apache.shiro.authc.AuthenticationException;
import org.apache.shiro.authc.AuthenticationInfo;
import org.apache.shiro.authc.AuthenticationToken;
import org.apache.shiro.authc.SimpleAuthenticationInfo;
import org.apache.shiro.authz.AuthorizationInfo;
import org.apache.shiro.realm.AuthorizingRealm;
import org.apache.shiro.subject.PrincipalCollection;
import org.apache.shiro.util.ByteSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class MyRealm extends AuthorizingRealm {
@Autowired
private IUserService service;
/**
* 授权信息
* @param principalCollection
* @return
*/
@Override
protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principalCollection) {
return null;
}
/**
* 认证信息
* @param authenticationToken
* @return
* @throws AuthenticationException
*/
@Override
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken authenticationToken) throws AuthenticationException {
//1获取用户身份信息
String username = authenticationToken.getPrincipal().toString();
//2调用业务层获取用户信息(数据库)
User user = service.queryUserInfoByName(username);
//3非空判断,将数据封装返回
if (user != null){
AuthenticationInfo info = new SimpleAuthenticationInfo(
authenticationToken.getPrincipal(),
user.getPwd(),
ByteSource.Util.bytes("salt"),
authenticationToken.getPrincipal().toString()
);
return info;
}
return null;
}
}
7. ShiroConfig
package com.jmh.springbootshiro.config;
import com.jmh.springbootshiro.utls.MyRealm;
import org.apache.shiro.authc.credential.HashedCredentialsMatcher;
import org.apache.shiro.spring.web.config.DefaultShiroFilterChainDefinition;
import org.apache.shiro.web.mgt.DefaultWebSecurityManager;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class ShiroConfig {
@Autowired
private MyRealm myRealm;
/**
* 配置SecurityManager
* @return
*/
@Bean
public DefaultWebSecurityManager defaultWebSecurityManager(){
//1创建defaultWebSecurityManager 对象
DefaultWebSecurityManager defaultWebSecurityManager = new DefaultWebSecurityManager();
//2创建加密对象,设置相关属性
HashedCredentialsMatcher matcher = new HashedCredentialsMatcher();
//2.1采用md5加密
matcher.setHashAlgorithmName("MD5");
//2.2迭代加密次数
matcher.setHashIterations(3);
//3将加密对象存储到myRealm中
myRealm.setCredentialsMatcher(matcher);
//4将myRealm存入defaultWebSecurityManager 对象
defaultWebSecurityManager.setRealm(myRealm);
return defaultWebSecurityManager;
}
}
8. controller
package com.jmh.springbootshiro.controller;
import org.apache.shiro.SecurityUtils;
import org.apache.shiro.authc.AuthenticationException;
import org.apache.shiro.authc.AuthenticationToken;
import org.apache.shiro.authc.UsernamePasswordToken;
import org.apache.shiro.subject.Subject;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RestController;
/**
* <p>
* 用户表 前端控制器
* </p>
*
* @author jmh
* @since 2022-11-13
*/
@RestController
@RequestMapping("/user")
public class UserController {
@RequestMapping("/login")
public String login(String name,String pwd){
//1获取subject对象
Subject subject = SecurityUtils.getSubject();
//2封装请求数据到token
AuthenticationToken token = new UsernamePasswordToken(name,pwd);
//3调用login方法进行登录认证
try {
subject.login(token);
return "登录成功";
} catch (AuthenticationException e) {
e.printStackTrace();
return "登录失败";
}
}
@RequestMapping("/test")
public String test(){
return "test";
}
}
9. 配置application.yml文件
server:
port: 8080
spring:
application:
name: shiro
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/shirodb?characterEncoding=utf-8&useSSL=false
username: root
password: 1234
jackson:
date-format: yyyy-MM-dd HH:mm:ss
time-zone: GMT+8
#整合mybatisPlus
mybatis-plus:
#配置SQL映射文件路径
mapper-locations: classpath:mapper/*.xml
#配置日志输出
logging:
level:
com.jmh.springbootshiro.mapper: debug
#开放shiro认证信息访问路径
shiro:
loginUrl: /user/login
10. 配置启动类
package com.jmh.springbootshiro;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@MapperScan("com.jmh.springbootshiro.mapper")
public class SpringbootShiroApplication {
public static void main(String[] args) {
SpringApplication.run(SpringbootShiroApplication.class, args);
}
}
11. 启动项目开始测试