一、写在前面
记录FFMpeg PHP使用的点滴,它可以处理音视频(提取图片,进行转码,添加水印,旋转等等),对我们来说是一个强大的轮子。
二、安装步骤
1.添加包依赖,前提是composer已加载。
composer require php-ffmpeg/php-ffmpeg
requires
- php: ^5.3.9 || ^7.0
顺利的话,composer.json里已经有了:
"php-ffmpeg/php-ffmpeg": "^0.14",
2.此时如果使用官方例子来测试的话,可能会报错:Unable to load FFProbe,关于这个问题的讨论可以查看这里,我们需要了解下php-ffmpeg的服务端环境:这里列出了不同系统安装FFmpeg的方法(安装FFmpeg),我们以mac为例,使用brew来安装:
Press Command+Space and type Terminal and press enter/return key.
Run in Terminal app:
ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)" < /dev/null 2> /dev/null
and press enter/return key.
If the screen prompts you to enter a password, please enter your Mac's user password to continue. When you type the password, it won't be displayed on screen, but the system would accept it. So just type your password and press ENTER/RETURN key. Then wait for the command to finish.
Run:
brew install ffmpeg
Done! You can now use ffmpeg
.(了解更多参考这里)
三、封装方法
function ffmpeg()
{
$configuration = array(
'ffmpeg.binaries' => '/usr/local/bin/ffmpeg',
'ffprobe.binaries' => '/usr/local/bin/ffprobe', //这里是电脑或服务器上所安装的位置
// 'timeout' => 3600, // The timeout for the underlying process
// 'ffmpeg.threads' => 12, // The number of threads that FFMpeg should use
);
$ffmpeg = FFMpeg\FFMpeg::create($configuration);
//这里根据需要选择视频(本服务器的视频或者其他网站的视频地址)
// $video = $ffmpeg->open(ROOT_PATH.'public/upload/test/test.mpg');
// $video = $ffmpeg->open(ROOT_PATH.'public/upload/test/test1.mp4');
$video = $ffmpeg->open('https://qiniu-xpc4.xpccdn.com/5cde40867122c.mp4');
// $audio = $ffmpeg->open(ROOT_PATH.'public/upload/test/audio.mp3');
//1.1提取单张图片-第n秒的视频帧
$video->frame(FFMpeg\Coordinate\TimeCode::fromSeconds(10))
->save(ROOT_PATH.'public/upload/test/frame_10.jpg');
$video->frame(FFMpeg\Coordinate\TimeCode::fromSeconds(25))
->save(ROOT_PATH.'public/upload/test/frame_25.jpg');
//1.2提取多张图片--失败
$video->filters()->extractMultipleFrames(FFMpeg\Filters\Video\ExtractMultipleFramesFilter::FRAMERATE_EVERY_10SEC, ROOT_PATH.'public/upload/test/')
->synchronize();
$video->save(new FFMpeg\Format\Video\X264(), ROOT_PATH.'public/upload/test/');
//2.1将视频转码
$format = new FFMpeg\Format\Video\X264();
$format->on('progress', function ($video, $format, $percentage) {
echo "$percentage % transcoded";
});
$format->setKiloBitrate(1000)
->setAudioChannels(2)
->setAudioKiloBitrate(256);
$video->save($format, ROOT_PATH.'public/upload/test/test.avi');
$video->save(new FFMpeg\Format\Video\X264(), ROOT_PATH.'public/upload/test/export-x264.mp4')
->save(new FFMpeg\Format\Video\WMV(), ROOT_PATH.'public/upload/test/export-wmv.wmv')
->save(new FFMpeg\Format\Video\WebM(), ROOT_PATH.'public/upload/test/export-webm.webm');
// 2.2将视频按指定点剪切,并调整大小---失败-Encoding failed
$video->filters()->clip(FFMpeg\Coordinate\TimeCode::fromSeconds(30), FFMpeg\Coordinate\TimeCode::fromSeconds(15));
$video->filters()->resize(new FFMpeg\Coordinate\Dimension(320, 240), FFMpeg\Filters\Video\ResizeFilter::RESIZEMODE_INSET, true);
$video->save(new FFMpeg\Format\Video\X264(), ROOT_PATH.'public/upload/test/cut_and_resize.mp4');
//2.3将视频旋转90角度
$angle = FFMpeg\Filters\Video\RotateFilter::ROTATE_90;
$video->filters()->rotate($angle);
$video->save(new FFMpeg\Format\Video\X264(), ROOT_PATH.'public/upload/test/ROTATE_90.mpg');
//2.4给视频添加水印
$video->filters()
->watermark(ROOT_PATH.'public/upload/test/water.png', array(
'position' => 'relative',
'bottom' => 50,
'right' => 50,
));
$video->save(new FFMpeg\Format\Video\X264(), ROOT_PATH.'public/upload/test/watermark.mp4');
//2.5拼接不同视频,生成新的视频
$video
->concat(array(ROOT_PATH.'public/upload/test/watermark.mp4', ROOT_PATH.'public/upload/test/export-x264.mp4'))
->saveFromSameCodecs(ROOT_PATH.'public/upload/test/contact.mp4', TRUE);
//3.1从视频里提取音频,并生成波形图片
$audio_format = new FFMpeg\Format\Audio\Mp3();//Set an audio format
$video->save($audio_format, ROOT_PATH.'public/upload/test/audio.mp3');//Extract the audio into a new file as mp3
$audio = $ffmpeg->open( ROOT_PATH.'public/upload/test/audio.mp3' );//Set the audio file
$waveform = $audio->waveform(640, 120, array('#00FF00')); // Create the waveform
$waveform->save(ROOT_PATH.'public/upload/test/waveform.png' );
//3.2从视频里提取gif图片
$video->gif(FFMpeg\Coordinate\TimeCode::fromSeconds(2), new FFMpeg\Coordinate\Dimension(640, 480), 3)
->save(ROOT_PATH.'public/upload/test/gif.gif');
//4.音频转码
$format = new FFMpeg\Format\Audio\Flac();
$format->on('progress', function ($audio, $format, $percentage) {
echo "$percentage % transcoded";
});
$format
->setAudioChannels(2)
->setAudioKiloBitrate(256);
$audio->save($format, ROOT_PATH.'public/upload/test/audio.flac');
//5.媒体相关信息
$ffprobe = FFMpeg\FFProbe::create($configuration);
$ffprobe
->format(ROOT_PATH.'public/upload/test/contact.mp4') // extracts file informations
->get('duration'); // returns the duration property
print_r($ffprobe);exit;
//下面是打印的数据
[properties:FFMpeg\FFProbe\DataMapping\AbstractData:private] => Array
(
[filename] => /Applications/MAMP/htdocs/manfan_app/public/upload/test/contact.mp4
[nb_streams] => 1
[nb_programs] => 0
[format_name] => mov,mp4,m4a,3gp,3g2,mj2
[format_long_name] => QuickTime / MOV
[start_time] => 0.000000
[duration] => 22.000000
[size] => 2779382
[bit_rate] => 1010684
[probe_score] => 100
[tags] => Array
(
[major_brand] => isom
[minor_version] => 512
[compatible_brands] => isomiso2avc1mp41
[encoder] => Lavf58.20.100
)
)
}
关于以上方法的说明:
1.以上处理的文件,均在:public/upload/test/文件夹下,更多例子和小功能访问github/packagist查看。
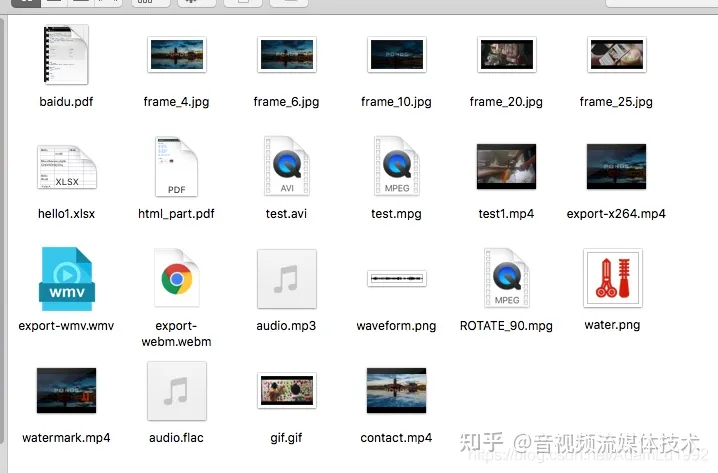
2.其中有两点测试报错误:Encoding failed,关于这点可以参考:讨论,基本是环境配置或者依赖其他包未加载的问题。
最后,有什么问题欢迎讨论,争取少跳坑。
原文 FFMpeg-从安装到使用_use ffmpeg\ffmpeg;_Adam_Lu的博客-CSDN博客
★文末名片可以免费领取音视频开发学习资料,内容包括(FFmpeg ,webRTC ,rtmp ,hls ,rtsp ,ffplay ,srs)以及音视频学习路线图等等。
见下方!↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓↓