题目
你会得到一个双链表,其中包含的节点有一个下一个指针、一个前一个指针和一个额外的 子指针 。这个子指针可能指向一个单独的双向链表,也包含这些特殊的节点。这些子列表可以有一个或多个自己的子列表,以此类推,以生成如下面的示例所示的 多层数据结构 。
给定链表的头节点 head ,将链表 扁平化 ,以便所有节点都出现在单层双链表中。让 curr 是一个带有子列表的节点。子列表中的节点应该出现在扁平化列表中的 curr 之后 和 curr.next 之前 。
返回 扁平列表的 head 。列表中的节点必须将其 所有 子指针设置为 null 。
示例 1:
输入:head = [1,2,3,4,5,6,null,null,null,7,8,9,10,null,null,11,12]
输出:[1,2,3,7,8,11,12,9,10,4,5,6]
解释:输入的多级列表如上图所示。
扁平化后的链表如下图:
示例 2:
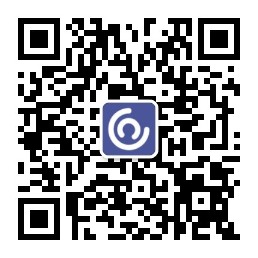
输入:head = [1,2,null,3]
输出:[1,3,2]
解释:输入的多级列表如上图所示。
扁平化后的链表如下图:
示例 3:
输入:head = []
输出:[]
说明:输入中可能存在空列表。
提示:
- 节点数目不超过 1000
- 1 <= Node.val <= 105
如何表示测试用例中的多级链表?
以 示例 1 为例:
1---2---3---4---5---6--NULL
|
7---8---9---10--NULL
|
11--12--NULL
序列化其中的每一级之后:
[1,2,3,4,5,6,null]
[7,8,9,10,null]
[11,12,null]
为了将每一级都序列化到一起,我们需要每一级中添加值为 null 的元素,以表示没有节点连接到上一级的上级节点。
[1,2,3,4,5,6,null]
[null,null,7,8,9,10,null]
[null,11,12,null]
合并所有序列化结果,并去除末尾的 null 。
[1,2,3,4,5,6,null,null,null,7,8,9,10,null,null,11,12]
解题思路
1.因为链表可能为空,所以我们先判断一下链表是否为空,若为空直接返回 head 节点。
2.我们构造两个方法 child()和 tail(),child()用于找到链表中带孩子的节点,若链表中不存在带孩子的节点,那么它会返回 null ,tail()用于找出链表的尾节点。
3.我们先用 while 循环去判断此链表是否还存在带有孩子链表的节点,若不存在直接返回 head ,若存在我们先设置一个 father 节点用于存储有孩子的节点,再设置一个 child 用于存储 这个有孩子的节点指向的他的孩子,tail 节点存储了孩子链表的尾节点,用于与父链表进行连接。
4.如果 father 节点的 next 节点指向空,那么我们直接将 father.next = child; child.prev = father; father.child = null;就不用再考虑 father 后面的节点,因为后面已经没有节点了,如果father 节点的 next 节点不为空则我们令 tail.next = father.next; father.next.prev = tail; father.next = child; child.prev = father; father.child = null;不但要完成 father 前半部分与孩子链表的连接,还要完成 father 后半部分与孩子节点的连接,直到链表不存在孩子链表,返回head。
举个栗子:输入:head = [1,2,3,4,5,6,null,null,null,7,8,9,10]
(1)chlid()方法遍历head 找出带有孩子的节点
(2)此时cur.child != null 跳出 while 循环,返回 cur 节点。
(3)设置一个child节点指向father节点的孩子节点
(4)用tail()方法对孩子链表进行遍历,找出孩子链表的尾节点
![]()
(5)此时 temp.next = null 跳出 while 循环,返回 temp
(6)因为father.next节点不为空,所以孩子链表的首尾都要进行连接
tail.next = father.next;
father.next.prev = tail;
father.next = child;
child.prev = father;
father.child = null;
return head;
代码实现
class Solution {
public Node flatten(Node head) {
if(head == null){
return head;
}
while(child(head) != null){
Node father = child(head);
Node child = father.child;
Node tail = tail(child);
if(father.next == null){
father.next = child;
child.prev = father;
father.child = null;
}else{
tail.next = father.next;
father.next.prev = tail;
father.next = child;
child.prev = father;
father.child = null;
}
}
return head;
}
public Node child(Node head){
Node cur = head;
while(cur != null && cur.child == null ){
cur = cur.next;
}
return cur;
}
public Node tail(Node head){
Node temp = head;
while(temp.next != null){
temp = temp.next;
}
return temp;
}
}