资料源于刘铁猛的C#视频教程
红色是主线程,其它三种是三个方法。
右边三个图分别是同步调用,异步,异步调用的示意图。
1 单播委托
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Threading; namespace Del { public delegate double Calc(double x, double y);//委托是类申明时与类平级 class Program { static void Main(string[] args) { Student stu1 = new Student(){ ID = 1, PenColor = ConsoleColor.Yellow}; Student stu2 = new Student(){ ID = 2, PenColor = ConsoleColor.Green}; Student stu3 = new Student() { ID = 3, PenColor = ConsoleColor.Red }; Action action1 = new Action(stu1.DoHomework); Action action2 = new Action(stu2.DoHomework); Action action3 = new Action(stu3.DoHomework); action1.Invoke(); action2.Invoke(); action3.Invoke(); } } class Student { public int ID { get; set; } public ConsoleColor PenColor { get; set; } public void DoHomework() { for (int i = 0; i < 5; i++) { Console.ForegroundColor = this.PenColor; Console.WriteLine("Student {0} doing homework {1} hours.", this.ID, i); Thread.Sleep(1000); } } } }
扫描二维码关注公众号,回复:
1559689 查看本文章
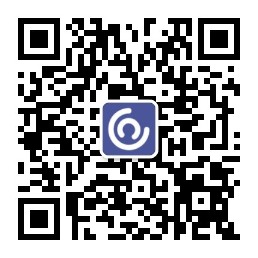
2 多播委托
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Threading; namespace Del { public delegate double Calc(double x, double y);//委托是类申明时与类平级 class Program { static void Main(string[] args) { Student stu1 = new Student(){ ID = 1, PenColor = ConsoleColor.Yellow}; Student stu2 = new Student(){ ID = 2, PenColor = ConsoleColor.Green}; Student stu3 = new Student() { ID = 3, PenColor = ConsoleColor.Red }; Action action1 = new Action(stu1.DoHomework); Action action2 = new Action(stu2.DoHomework); Action action3 = new Action(stu3.DoHomework); //action1.Invoke(); //action2.Invoke(); //action3.Invoke(); action1 += action2; action1 += action3; action1.Invoke(); } } class Student { public int ID { get; set; } public ConsoleColor PenColor { get; set; } public void DoHomework() { for (int i = 0; i < 5; i++) { Console.ForegroundColor = this.PenColor; Console.WriteLine("Student {0} doing homework {1} hours.", this.ID, i); Thread.Sleep(1000); } } } }
3 同步直接调用
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Threading; namespace Del { public delegate double Calc(double x, double y);//委托是类申明时与类平级 class Program { static void Main(string[] args) { Student stu1 = new Student(){ ID = 1, PenColor = ConsoleColor.Yellow}; Student stu2 = new Student(){ ID = 2, PenColor = ConsoleColor.Green}; Student stu3 = new Student() { ID = 3, PenColor = ConsoleColor.Red }; Action action1 = new Action(stu1.DoHomework); Action action2 = new Action(stu2.DoHomework); Action action3 = new Action(stu3.DoHomework); //第一种 同步直 接调用 stu1.DoHomework(); stu2.DoHomework(); stu3.DoHomework(); for (int i = 0; i < 10; i++) { Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("Thread {0}", i); Thread.Sleep(1000); } } } class Student { public int ID { get; set; } public ConsoleColor PenColor { get; set; } public void DoHomework() { for (int i = 0; i < 5; i++) { Console.ForegroundColor = this.PenColor; Console.WriteLine("Student {0} doing homework {1} hours.", this.ID, i); Thread.Sleep(1000); } } } }
4 同步 单播委托间接调用
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Threading; namespace Del { public delegate double Calc(double x, double y);//委托是类申明时与类平级 class Program { static void Main(string[] args) { Student stu1 = new Student(){ ID = 1, PenColor = ConsoleColor.Yellow}; Student stu2 = new Student(){ ID = 2, PenColor = ConsoleColor.Green}; Student stu3 = new Student() { ID = 3, PenColor = ConsoleColor.Red }; Action action1 = new Action(stu1.DoHomework); Action action2 = new Action(stu2.DoHomework); Action action3 = new Action(stu3.DoHomework); //第一种 同步 间接调用 action1.Invoke(); action2.Invoke(); action3.Invoke(); for (int i = 0; i < 10; i++) { Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("Thread {0}", i); Thread.Sleep(1000); } } } class Student { public int ID { get; set; } public ConsoleColor PenColor { get; set; } public void DoHomework() { for (int i = 0; i < 5; i++) { Console.ForegroundColor = this.PenColor; Console.WriteLine("Student {0} doing homework {1} hours.", this.ID, i); Thread.Sleep(1000); } } } }
5 同步 多播委托间接调用
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Threading; namespace Del { public delegate double Calc(double x, double y);//委托是类申明时与类平级 class Program { static void Main(string[] args) { Student stu1 = new Student(){ ID = 1, PenColor = ConsoleColor.Yellow}; Student stu2 = new Student(){ ID = 2, PenColor = ConsoleColor.Green}; Student stu3 = new Student() { ID = 3, PenColor = ConsoleColor.Red }; Action action1 = new Action(stu1.DoHomework); Action action2 = new Action(stu2.DoHomework); Action action3 = new Action(stu3.DoHomework); //第一种 同步 多播委托间接调用 action1 += action2; action1 += action3; for (int i = 0; i < 10; i++) { Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("Thread {0}", i); Thread.Sleep(1000); } } } class Student { public int ID { get; set; } public ConsoleColor PenColor { get; set; } public void DoHomework() { for (int i = 0; i < 5; i++) { Console.ForegroundColor = this.PenColor; Console.WriteLine("Student {0} doing homework {1} hours.", this.ID, i); Thread.Sleep(1000); } } } }
6 委托 隐式异步调用
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Threading; namespace Del { public delegate double Calc(double x, double y);//委托是类申明时与类平级 class Program { static void Main(string[] args) { Student stu1 = new Student(){ ID = 1, PenColor = ConsoleColor.Yellow}; Student stu2 = new Student(){ ID = 2, PenColor = ConsoleColor.Green}; Student stu3 = new Student() { ID = 3, PenColor = ConsoleColor.Red }; Action action1 = new Action(stu1.DoHomework); Action action2 = new Action(stu2.DoHomework); Action action3 = new Action(stu3.DoHomework); //异步 委托间接调用 action1.BeginInvoke(null, null); action2.BeginInvoke(null, null); action3.BeginInvoke(null, null); for (int i = 0; i < 10; i++) { Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("Thread {0}", i); Thread.Sleep(1000); } } } class Student { public int ID { get; set; } public ConsoleColor PenColor { get; set; } public void DoHomework() { for (int i = 0; i < 5; i++) { Console.ForegroundColor = this.PenColor; Console.WriteLine("Student {0} doing homework {1} hours.", this.ID, i); Thread.Sleep(1000); } } } }
7 显式异步调用
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Threading; namespace Del { public delegate double Calc(double x, double y);//委托是类申明时与类平级 class Program { static void Main(string[] args) { Student stu1 = new Student(){ ID = 1, PenColor = ConsoleColor.Yellow}; Student stu2 = new Student(){ ID = 2, PenColor = ConsoleColor.Green}; Student stu3 = new Student() { ID = 3, PenColor = ConsoleColor.Red }; Thread thread1 = new Thread(new ThreadStart(stu1.DoHomework)); Thread thread2 = new Thread(new ThreadStart(stu2.DoHomework)); Thread thread3 = new Thread(new ThreadStart(stu3.DoHomework)); thread1.Start(); thread2.Start(); thread3.Start(); for (int i = 0; i < 10; i++) { Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("Thread {0}", i); Thread.Sleep(500); } } } class Student { public int ID { get; set; } public ConsoleColor PenColor { get; set; } public void DoHomework() { for (int i = 0; i < 5; i++) { Console.ForegroundColor = this.PenColor; Console.WriteLine("Student {0} doing homework {1} hours.", this.ID, i); Thread.Sleep(1000); } } } }
8 task异步线程
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading; using System.Threading.Tasks; namespace Del { public delegate double Calc(double x, double y);//委托是类申明时与类平级 class Program { static void Main(string[] args) { Student stu1 = new Student(){ ID = 1, PenColor = ConsoleColor.Yellow}; Student stu2 = new Student(){ ID = 2, PenColor = ConsoleColor.Green}; Student stu3 = new Student() { ID = 3, PenColor = ConsoleColor.Red }; Task task1 = new Task(new Action(stu1.DoHomework)); Task task2 = new Task(new Action(stu2.DoHomework)); Task task3 = new Task(new Action(stu3.DoHomework)); task1.Start(); task2.Start(); task3.Start(); for (int i = 0; i < 10; i++) { Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("Thread {0}", i); Thread.Sleep(500); } } } class Student { public int ID { get; set; } public ConsoleColor PenColor { get; set; } public void DoHomework() { for (int i = 0; i < 5; i++) { Console.ForegroundColor = this.PenColor; Console.WriteLine("Student {0} doing homework {1} hours.", this.ID, i); Thread.Sleep(1000); } } } }
6,7,8 都发生了资源的争夺,谁也不等谁。