本篇文章承接http://www.cnblogs.com/zhang-zhi/p/7646923.html#3807385,上篇文章描述了对文本文件的简单处理,本章节结合PYQT4实现该功能的GUI图形界面化,简单的UI界面可以更好的提高工具的实用性,所以在此进行一下记录。
主要实现功能效果展示如下:
1、打开本地对话框,选择文件
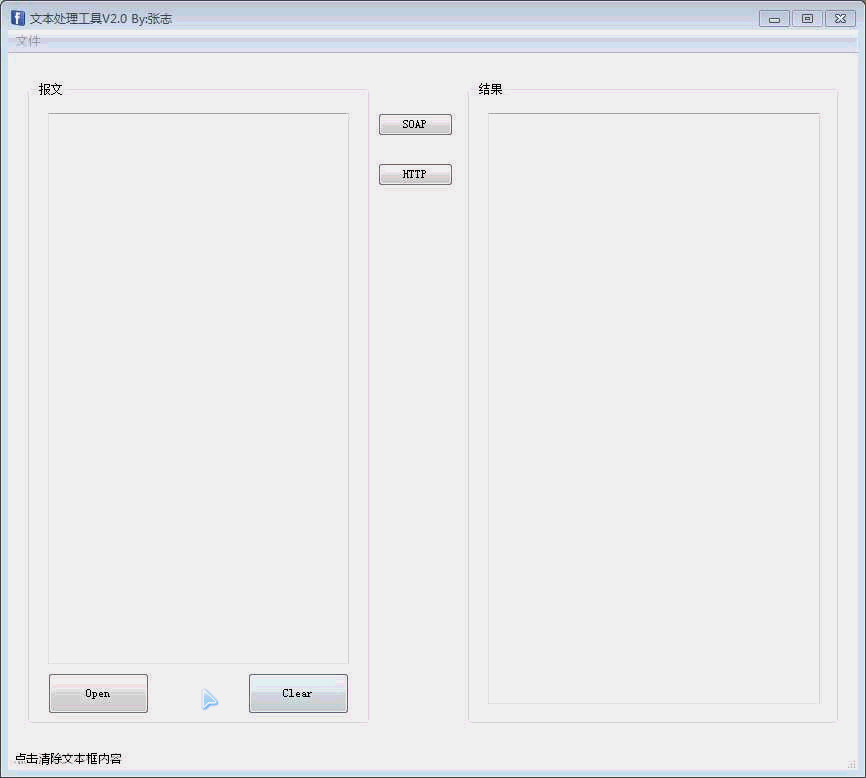
2、文件打开,报文输入及报文清空功能
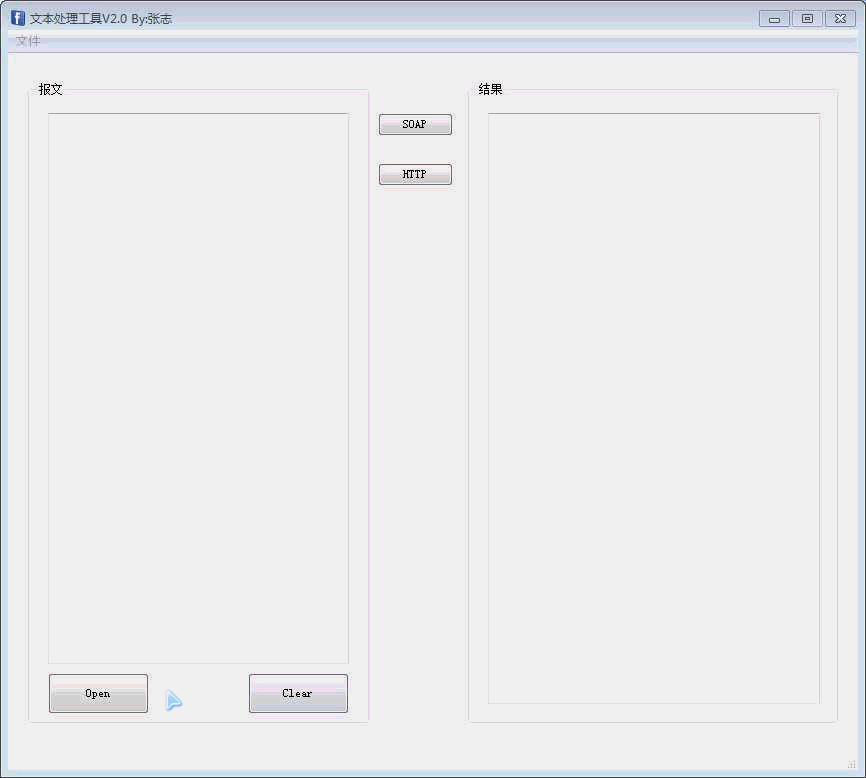
3、核心功能,报文格式转换
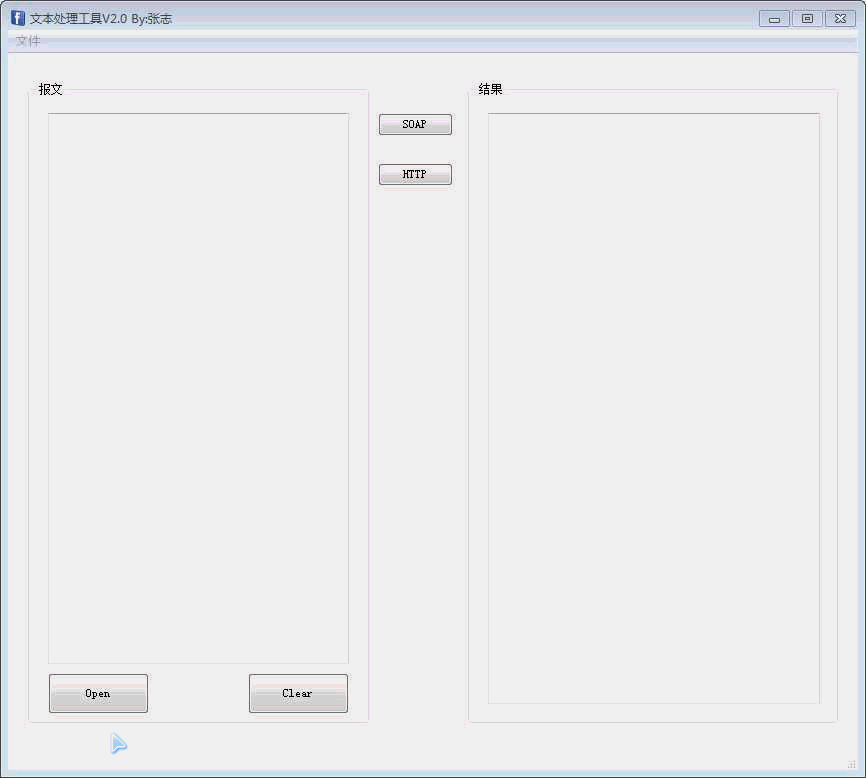
4、退出
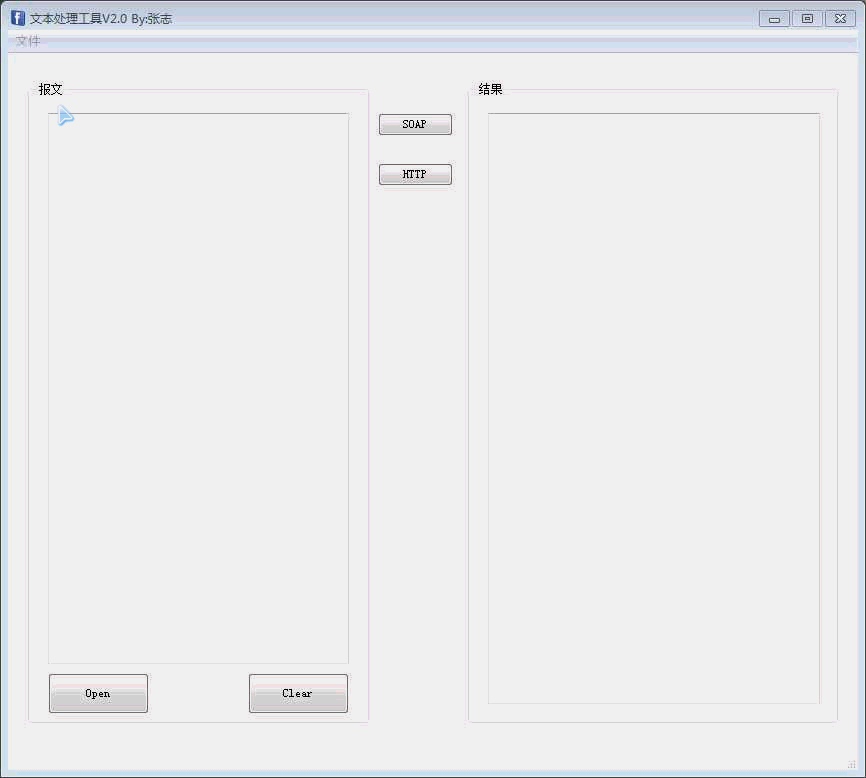
一、Python GUI开发之PYQT4
1、先安装PYQT4
有两种方式可以下载:
①pip下载,终端运行pip install pyqt4
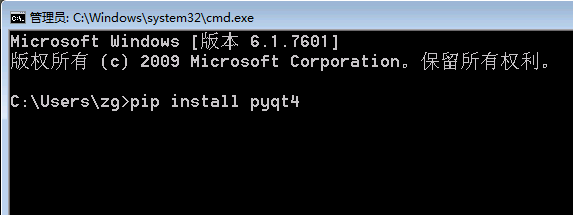
②pycharm工具安装
打开pycharm工具,点击“File”→“settings”→“Project:Project Interpreter”→“+(install)”→“输入pyqt4”,安装即可。
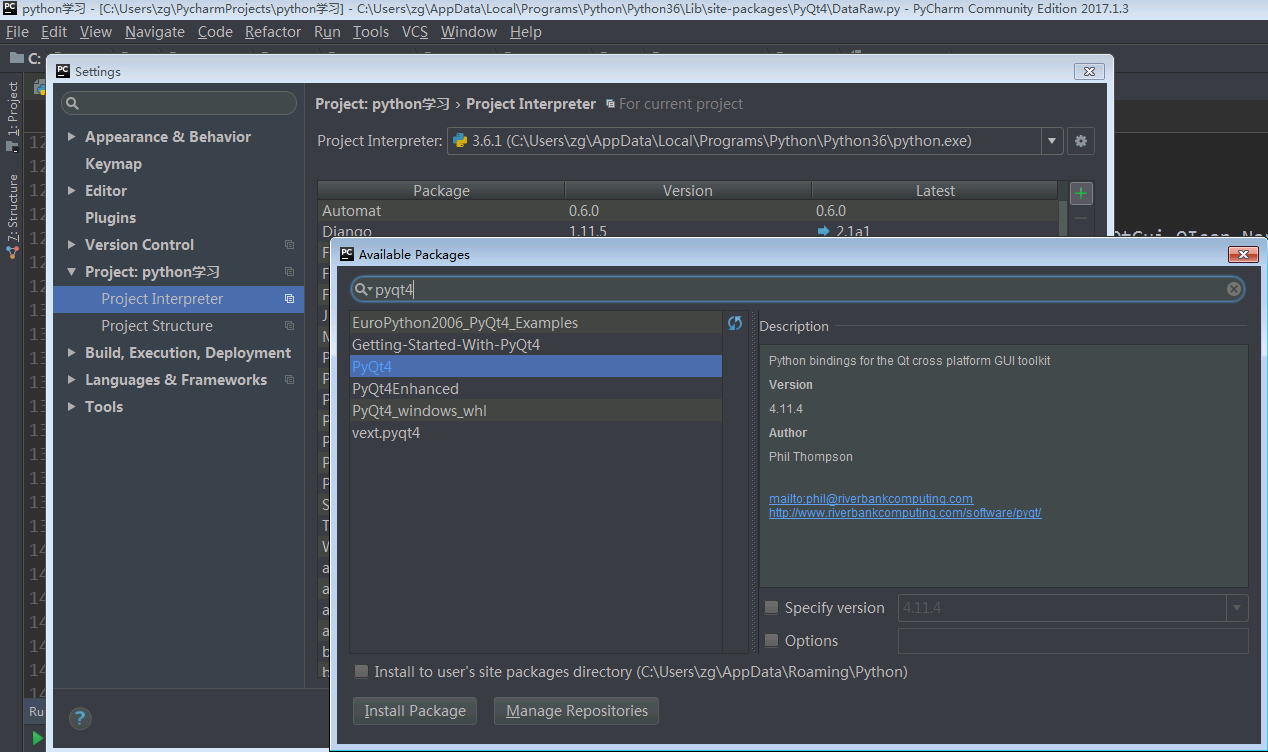
2、在pyqt4的安装目录:C:\Users\zg\AppData\Local\Programs\Python\Python36\Lib\site-packages\PyQt4下,找到
应用程序启动即可。
3、启动designer.exe程序后,进入Qt设计师主界面,就可以开始你的GUI设计之路了。。
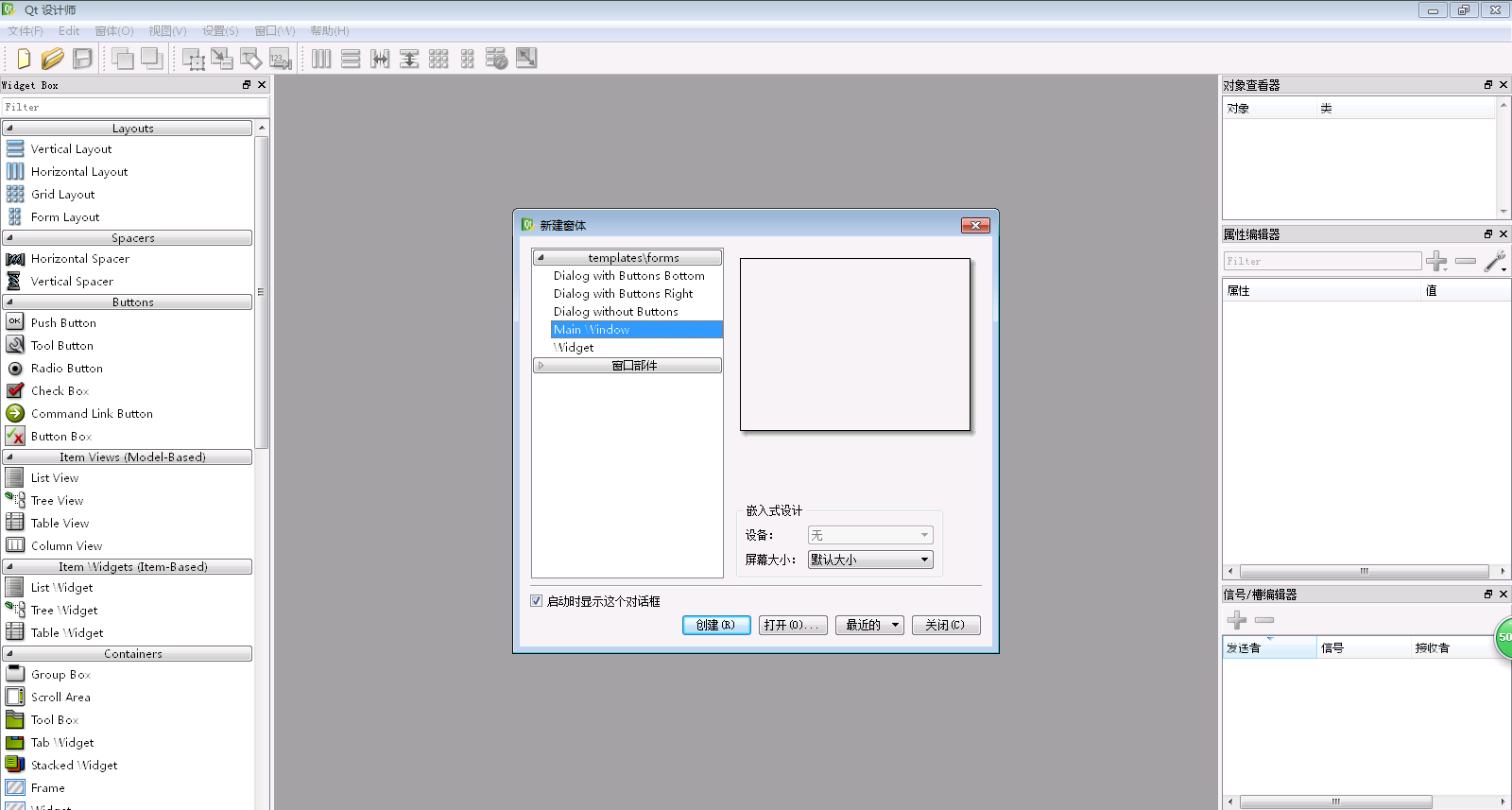
4、选择“Main window”窗体,创建~
5、接下来是重点,pyqt4提供了直接将ui文件转为py文件的功能,非常实用,具体方法为:
编辑好ui界面后,点击保存(最好保存在C:\Users\zg\AppData\Local\Programs\Python\Python36\Scripts目录下),终端执行命令:pyuic4 xxx.ui -o xxx.py即可生成py代码文件xxx.py。
6、根据生成的py代码文件,再进行进一步的功能实现,下面贴出我的GUI界面及代码,仅供参考学习~
一个简单的文本处理工具,实现接口报文的转换与生成
代码如下:
1 # -*- coding: utf-8 -*- 2 3 # Form implementation generated from reading ui file 'DataRaw.ui' 4 # 5 # Created by: PyQt4 UI code generator 4.11.4 6 # 7 # WARNING! All changes made in this file will be lost! 8 import sys 9 import re 10 from PyQt4 import QtCore, QtGui 11 try: 12 _fromUtf8 = QtCore.QString.fromUtf8 13 except AttributeError: 14 def _fromUtf8(s): 15 return s 16 17 try: 18 _encoding = QtGui.QApplication.UnicodeUTF8 19 def _translate(context, text, disambig): 20 return QtGui.QApplication.translate(context, text, disambig, _encoding) 21 except AttributeError: 22 def _translate(context, text, disambig): 23 return QtGui.QApplication.translate(context, text, disambig) 24 25 class Ui_MainWindow(QtGui.QMainWindow): 26 def __init__(self): 27 QtGui.QMainWindow.__init__(self) 28 self.setupUi(self) 29 30 def setupUi(self, MainWindow): 31 MainWindow.setObjectName(_fromUtf8("MainWindow")) 32 MainWindow.resize(850, 740) 33 # MainWindow.setMaximumSize(850, 740) 34 #禁止窗口最大化 35 MainWindow.setWindowFlags(QtCore.Qt.WindowMinimizeButtonHint) 36 #禁止拖拉窗口 37 MainWindow.setFixedSize(MainWindow.width(), MainWindow.height()); 38 MainWindow.setMouseTracking(False) 39 icon = QtGui.QIcon() 40 icon.addPixmap(QtGui.QPixmap(_fromUtf8(r"C:\Users\zg\Desktop\ico\Facebook.ico")), QtGui.QIcon.Normal,QtGui.QIcon.Off) 41 MainWindow.setWindowIcon(icon) 42 self.centralwidget = QtGui.QWidget(MainWindow) 43 self.centralwidget.setObjectName(_fromUtf8("centralwidget")) 44 self.pushButton = QtGui.QPushButton(self.centralwidget) 45 self.pushButton.setGeometry(QtCore.QRect(370, 60, 75, 23)) 46 self.pushButton.setObjectName(_fromUtf8("pushButton")) 47 self.pushButton_2 = QtGui.QPushButton(self.centralwidget) 48 self.pushButton_2.setGeometry(QtCore.QRect(370, 110, 75, 23)) 49 self.pushButton_2.setObjectName(_fromUtf8("pushButton_2")) 50 self.groupBox = QtGui.QGroupBox(self.centralwidget) 51 self.groupBox.setGeometry(QtCore.QRect(20, 30, 341, 641)) 52 self.groupBox.setObjectName(_fromUtf8("groupBox")) 53 self.textEdit_2 = QtGui.QTextEdit(self.groupBox) 54 self.textEdit_2.setGeometry(QtCore.QRect(20, 30, 301, 551)) 55 #设置报文框字体 56 font = QtGui.QFont() 57 font.setFamily(_fromUtf8("新宋体")) 58 font.setPointSize(10) 59 font.setBold(False) 60 font.setWeight(50) 61 self.textEdit_2.setFont(font) 62 self.textEdit_2.setMidLineWidth(1) 63 #设置报文框垂直滚动条 64 self.textEdit_2.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAsNeeded) 65 #设置报文框水平滚动条 66 self.textEdit_2.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAsNeeded) 67 self.textEdit_2.setTabChangesFocus(True) 68 self.textEdit_2.setUndoRedoEnabled(False) 69 #设置报文框取消自动换行 70 self.textEdit_2.setLineWrapMode(QtGui.QTextEdit.NoWrap) 71 self.textEdit_2.setOverwriteMode(False) 72 self.textEdit_2.setCursorWidth(1) 73 self.textEdit_2.setTextInteractionFlags(QtCore.Qt.LinksAccessibleByKeyboard | QtCore.Qt.LinksAccessibleByMouse | QtCore.Qt.TextBrowserInteraction | QtCore.Qt.TextEditable | QtCore.Qt.TextEditorInteraction | QtCore.Qt.TextSelectableByKeyboard | QtCore.Qt.TextSelectableByMouse) 74 self.textEdit_2.setMouseTracking(True) 75 self.textEdit_2.setAutoFillBackground(False) 76 self.textEdit_2.setObjectName(_fromUtf8("textEdit_2")) 77 78 self.pushButton_3 = QtGui.QPushButton(self.groupBox) 79 self.pushButton_3.setGeometry(QtCore.QRect(20, 590, 101, 41)) 80 self.pushButton_3.setObjectName(_fromUtf8("pushButton_3")) 81 self.pushButton_4 = QtGui.QPushButton(self.groupBox) 82 self.pushButton_4.setGeometry(QtCore.QRect(220, 590, 101, 41)) 83 self.pushButton_4.setObjectName(_fromUtf8("pushButton_4")) 84 self.groupBox_2 = QtGui.QGroupBox(self.centralwidget) 85 self.groupBox_2.setGeometry(QtCore.QRect(460, 30, 370, 641)) 86 self.groupBox_2.setObjectName(_fromUtf8("groupBox_2")) 87 self.textEdit = QtGui.QTextEdit(self.groupBox_2) 88 self.textEdit.setGeometry(QtCore.QRect(20, 30, 332, 591)) 89 #结果框字体设置 90 font = QtGui.QFont() 91 font.setFamily(_fromUtf8("新宋体")) 92 font.setPointSize(10) 93 font.setBold(False) 94 font.setWeight(50) 95 self.textEdit.setFont(font) 96 self.textEdit.setMidLineWidth(1) 97 #结果框垂直滚动条设置开关 98 self.textEdit.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAsNeeded) 99 # 结果框水平滚动条设置开关 100 self.textEdit.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAsNeeded) 101 self.textEdit.setTabChangesFocus(True) 102 self.textEdit.setUndoRedoEnabled(False) 103 #结果框取消自动换行 104 self.textEdit.setLineWrapMode(QtGui.QTextEdit.NoWrap) 105 self.textEdit.setOverwriteMode(False) 106 self.textEdit.setCursorWidth(1) 107 self.textEdit.setTextInteractionFlags(QtCore.Qt.LinksAccessibleByKeyboard | QtCore.Qt.LinksAccessibleByMouse | QtCore.Qt.TextBrowserInteraction | QtCore.Qt.TextEditable | QtCore.Qt.TextEditorInteraction | QtCore.Qt.TextSelectableByKeyboard | QtCore.Qt.TextSelectableByMouse) 108 self.textEdit.setMouseTracking(True) 109 self.textEdit.setAutoFillBackground(False) 110 self.textEdit.setObjectName(_fromUtf8("textEdit")) 111 112 # self.customContextMenuRequested(QPoint) 113 MainWindow.setCentralWidget(self.centralwidget) 114 self.menubar = QtGui.QMenuBar(MainWindow) 115 self.menubar.setGeometry(QtCore.QRect(0, 0, 800, 23)) 116 self.menubar.setObjectName(_fromUtf8("menubar")) 117 self.menu = QtGui.QMenu(self.menubar) 118 self.menu.setContextMenuPolicy(QtCore.Qt.DefaultContextMenu) 119 self.menu.setObjectName(_fromUtf8("menu")) 120 MainWindow.setMenuBar(self.menubar) 121 self.statusbar = QtGui.QStatusBar(MainWindow) 122 self.statusbar.setObjectName(_fromUtf8("statusbar")) 123 MainWindow.setStatusBar(self.statusbar) 124 self.action = QtGui.QAction(MainWindow) 125 self.action.setCheckable(False) 126 icon = QtGui.QIcon() 127 icon.addPixmap(QtGui.QPixmap(_fromUtf8(r"C:\Users\zg\Desktop\---\ico\Open.ico")), QtGui.QIcon.Normal, QtGui.QIcon.Off) 128 self.action.setIcon(icon) 129 self.action.setShortcutContext(QtCore.Qt.WidgetShortcut) 130 self.action.setObjectName(_fromUtf8("action")) 131 self.action_2 = QtGui.QAction(MainWindow) 132 icon1 = QtGui.QIcon() 133 icon1.addPixmap(QtGui.QPixmap(_fromUtf8(r"C:\Users\zg\Desktop\---\ico\Log Out.ico")), QtGui.QIcon.Normal, QtGui.QIcon.Off) 134 self.action_2.setIcon(icon1) 135 self.action_2.setObjectName(_fromUtf8("action_2")) 136 self.menu.addAction(self.action) 137 self.menu.addAction(self.action_2) 138 self.menubar.addAction(self.menu.menuAction()) 139 140 self.retranslateUi(MainWindow) 141 QtCore.QObject.connect(self.action_2, QtCore.SIGNAL(_fromUtf8("triggered()")), MainWindow.close) 142 QtCore.QObject.connect(self.action, QtCore.SIGNAL(_fromUtf8("triggered()")), self.openfile) 143 QtCore.QObject.connect(self.pushButton, QtCore.SIGNAL(_fromUtf8("clicked()")), self.soap) 144 QtCore.QObject.connect(self.pushButton_2, QtCore.SIGNAL(_fromUtf8("clicked()")), self.http) 145 QtCore.QObject.connect(self.pushButton_3, QtCore.SIGNAL(_fromUtf8("clicked()")), self.openfile) 146 QtCore.QObject.connect(self.pushButton_4, QtCore.SIGNAL(_fromUtf8("clicked()")), self.textEdit_2.clear) 147 QtCore.QMetaObject.connectSlotsByName(MainWindow) 148 149 def soap(self): 150 linenum = 1 151 header = 'soap_request(\n\t"StepName=google", \n\t"ExpectedResponse=AnySoap", \n\t"URL=http://api.google.com/search/beta2", \n\t"SOAPEnvelope= "\n\t"<?xml version=\\"1.0\\" encoding=\\"utf-8\\"?>"\n' 152 last = '\n\t'+'"Snapshot=t1.inf",\n\t"ResponseParam=result",\n\tLAST );' 153 src = self.textEdit_2.toPlainText() 154 lines = src.splitlines() 155 Message = '' 156 for line in lines: 157 #判断该行是否为空行 158 if len(line.strip())>0: 159 #找出每行第一个非空字符的位置 160 num = re.search(r'\S', line).span()[0] 161 if linenum < len(lines): 162 #对每行进行拼接 163 line = "\t"+line[:num] + '"' + line[num:].replace('"',r'\"').rstrip() + '"' + '\n' 164 linenum += 1 165 else: 166 line = "\t"+line[:num] + '"' + line[num:].replace('"',r'\"').rstrip() + '"' + ',' 167 else: 168 line = '\n' 169 linenum += 1 170 Message += line 171 if len(lines)>0: 172 try: 173 Trans_Message = header + Message + last 174 #输出到界面 175 self.textEdit.clear() 176 self.textEdit.append(Trans_Message) 177 except: 178 print("输入错误") 179 else: 180 print("异常") 181 # http报文转换 182 def http(self): 183 linenum = 1 184 header = 'web_custom_request(\n\t\"name\",\n\t\"Method=POST\",\n\t\"URL=http://{SERVERIP}:7017/ciitcmp/commserver\",\n\t\"Body=\"\n' 185 last = '\tLAST );' 186 src = self.textEdit_2.toPlainText() 187 lines = src.splitlines() 188 Message = '' 189 for line in lines: 190 # 判断是否是空行 191 if len(line.strip()) == 0 or line.startswith('#'): 192 continue 193 # data = '\r\t\n' 194 linenum += 1 195 elif len(line.strip()) > 0 and linenum < len(lines): 196 num = re.search(r'\S', line).span()[0] 197 data = '\t' + line[:num] + '"' + line[num:].replace('"', r'\"').rstrip() + '"' + '\n' 198 linenum += 1 199 else: 200 num = re.search(r'\S', line).span()[0] 201 data = '\t' + line[:num] + '"' + line[num:].replace('"', r'\"').rstrip() + '"' + ',' + '\n' 202 Message += data 203 if len(lines)>0: 204 try: 205 # #print(myMd5_Digest) 206 Trans_Message = header + Message + last 207 #输出到界面 208 self.textEdit.clear() 209 self.textEdit.append(Trans_Message) 210 except: 211 pass 212 else: 213 pass 214 #打开本地文件夹 215 def openfile(self): 216 try: 217 filename = QtGui.QFileDialog.getOpenFileName(self,"Open file","/") 218 with open(filename, 'r') as f: 219 FileData = f.read() 220 self.textEdit_2.setPlainText(FileData) 221 except: 222 print("关闭文件对话框!") 223 def retranslateUi(self, MainWindow): 224 MainWindow.setWindowTitle(_translate("MainWindow", "文本处理工具V2.0 By:---", None)) 225 MainWindow.setStatusTip(_translate("MainWindow", "文本处理工具", None)) 226 self.pushButton.setStatusTip(_translate("MainWindow", "点击将报文转换为soap请求", None)) 227 self.pushButton.setText(_translate("MainWindow", "SOAP", None)) 228 self.pushButton_2.setStatusTip(_translate("MainWindow", "点击将报文转换为http请求", None)) 229 self.pushButton_2.setText(_translate("MainWindow", "HTTP", None)) 230 self.groupBox.setTitle(_translate("MainWindow", "报文", None)) 231 self.textEdit_2.setStatusTip(_translate("MainWindow", "文本输入框", None)) 232 self.pushButton_3.setStatusTip(_translate("MainWindow", "点击打开本地文件", None)) 233 self.pushButton_3.setText(_translate("MainWindow", "Open", None)) 234 self.pushButton_4.setStatusTip(_translate("MainWindow", "点击清除文本框内容", None)) 235 self.pushButton_4.setText(_translate("MainWindow", "Clear", None)) 236 self.groupBox_2.setTitle(_translate("MainWindow", "结果", None)) 237 self.textEdit.setStatusTip(_translate("MainWindow", "结果输出框", None)) 238 self.menu.setTitle(_translate("MainWindow", "文件", None)) 239 self.action.setText(_translate("MainWindow", "打开", None)) 240 self.action.setStatusTip(_translate("MainWindow", "点击打开本地文件", None)) 241 self.action.setShortcut(_translate("MainWindow", "Ctrl+D", None)) 242 self.action_2.setText(_translate("MainWindow", "退出", None)) 243 self.action_2.setStatusTip(_translate("MainWindow", "点击退出程序", None)) 244 self.action_2.setShortcut(_translate("MainWindow", "Ctrl+C", None)) 245 if __name__ =='__main__': 246 app = QtGui.QApplication(sys.argv) 247 Form = QtGui.QMainWindow() 248 ui = Ui_MainWindow() 249 ui.setupUi(Form) 250 Form.show() 251 sys.exit(app.exec_())
二、将py文件打包成exe文件
实现exe文件打包的工具很多,如:pyinstaller、py2exe、cxfreeze等,这里使用cxfreeze进行文件打包,直接使用pip install pyinstaller安装pyinstaller即可。
此时可以为exe文件添加自己想要的ico图标,方法如下:
注意:将ico文件放在py文件相同目录下,并修改xxx.spec文件(与xxx.py文件同一目录下,相同名字的spec文件)
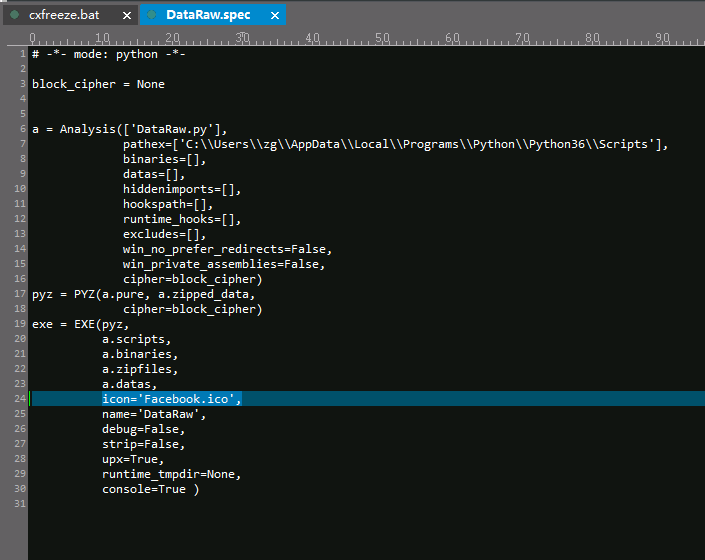
打开终端,执行pyinstaller xxx.spec
生成exe文件~
笔者用cxfreeze进行过exe文件的打包,过程中发生了一下,特此记录一下~
①、安装cxfreeze完毕后,到安装目录C:\Users\zg\AppData\Local\Programs\Python\Python36\Scripts\pyinstaller下,运行命令行窗口,执行命令cxfreeze,若发生以下报错
②、需在该目录下,创建cxfreeze.bat文件,文件内容为
3、执行如下命令打包即可
cxfreeze xxx.py --target-dir=d:\dist\ --icon=d:\xxxx.ico
以上就是就是Python PYQT4的简单应用了,实现的功能比较简单,后续会在进行相应的功能优化,敬请期待~~~
---恢复内容结束---