前言
数组拍平(扁平化数组)是面试中常考查的知识点,之前发过一篇基础版的数组拍平 传送门
通过之后的学习,又了解了一些其他的数组拍平的操作,详情请看下面的文章
flat
flat 方法可以直接用于数组拍平,不传入参数时默认拍平一层
const arr = ["1", ["2", "3"], ["4", ["5", ["6"]], "7"]];
// 不传参数时,默认“拉平”一层
arr.flat(); // ["1", "2", "3", "4", ["5", ["6"]], "7"]
传入一个整数参数,整数即“拉平”的层数
传入 <=0 的整数将返回原数组,不“拉平”
Infinity 关键字作为参数时,无论多少层嵌套,都会转为一维数组
const arr = [1, [2, 3], [4, [5, [6]], 7]];
// 传入一个整数参数,整数即“拉平”的层数
arr.flat(2);
// [1, 2, 3, 4, 5, [6], 7]
// 传入 <=0 的整数将返回原数组,不“拉平”
arr.flat(0);
arr.flat(-10);
// [1, [2, 3], [4, [5, [6]], 7]];
// Infinity 关键字作为参数时,无论多少层嵌套,都会转为一维数组
arr.flat(Infinity);
// [", 2, 3, 4, 5, 6, 7]
如果原数组有空位,flat()方法会跳过空位。
[1, 2, 3, 4,,].flat(); //[1, 2, 3, 4]
toString
toString 方法是一个比较简单的方法,他是把数组每个元素转化成字符串之后直接拍平
然后通过 map 遍历每个元素,让其变成 Number 类型
const arr = [1, [2, 3], [4, [5, [6]], 7]];
arr.toString();
arr.toString().split(',');
arr.toString().split(',').map(item) => Number(item);
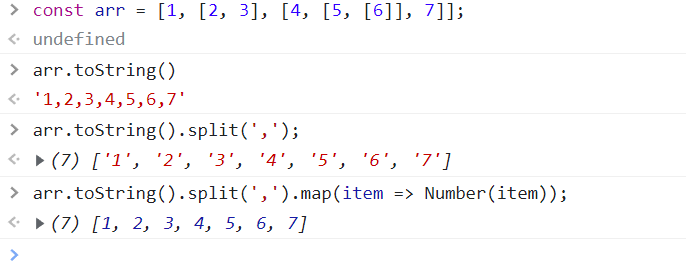
join
join 的操作和 toString 类似,最后也要通过 map 遍历每个元素,让其变成 Number 类型
const arr = [1, [2, 3], [4, [5, [6]], 7]];
arr.join();
arr.join().split(',');
arr.join().split(',').map(item) => Number(item);
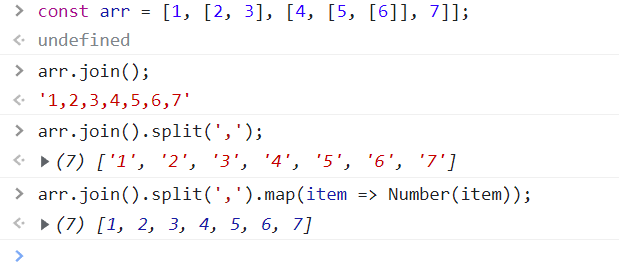
+1
这个方法比较奇怪,应该是arr ‘+1’后转换为string类型的话会自动排平
let a = [1, 2, [3, [4, 5]]] + '1'
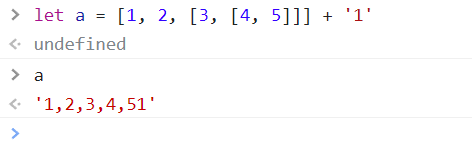
let a = [1, 2, [3, [4, 5]]] + ',1'
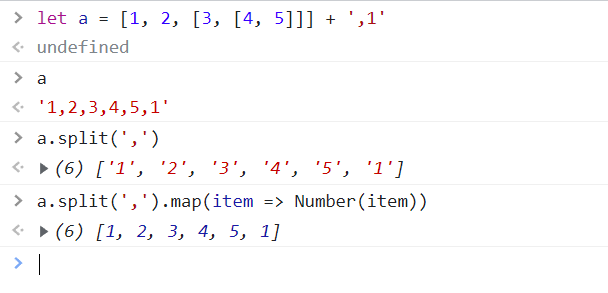
手写代码
简单数组拍平
在 ES2019 之前,可通过 reduce + concat 实现
由于 Array.prototype.concat 既可以连接数组又可以连接单项,十分巧妙
const flatten = (list) => list.reduce((a, b) => a.concat(b), []);
一个更简单的实现方式是 Array.prototype.concat 与 ... 运算符
const flatten = (list) => [].concat(...list);
深层数组拍平
如果要求深层数组打平,可以加一个判断,递归 调用函数
const flatten = (list) =>
list.reduce((a, b) => a.concat(Array.isArray(b) ? flatten(b) : b), []);
优化上面的代码,加上自定义拍平的层数,默认为 1
function flatten(list, depth = 1) {
if (depth === 0) return list;
return list.reduce(
(a, b) => a.concat(Array.isArray(b) ? flatten(b, depth - 1) : b),
[]
);
}
文章如有错误,恳请大家提出问题,本人不胜感激 。 不懂的地方可以评论,我都会 一 一 回复
文章对大家有帮助的话,希望大家能动手点赞鼓励,大家未来一起努力 长路漫漫,道阻且长