大家好,我是锋哥,看到一个不错的springboot+vue前后端分离的学生选课管理系统,分享下哈。
项目介绍
这是一个采用前后端分离开发的项目,前端采用 Vue 开发、后端采用 SpringBoot + Mybatis 开发。
项目部署
1. 将 studentms.sql 导入mysql数据库
2. 运行前端webstorm导入student_client运行
3. 运行后端idea导入student_server
项目展示
1、登陆界面
2、admin 主界面
3、动态搜索框与表格展示
4、学生端首页展示
5、教师端成绩搜索与编辑
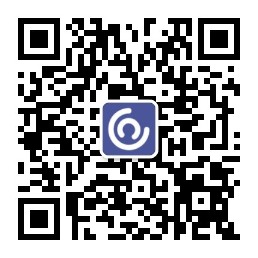
前端部分
1、项目运行
由于涉及大量的 ES6/7 等新属性,node 需要 6.0 以上版本
2、技术栈
-
Vuex
-
Router
-
Axios
-
Element ui
-
sessionStorage
3、项目介绍
采用 vue 2.0 开发,通过调用后端提供的数据接口实现数据的动态渲染。项目默认端口号 8080
-
使用监视器,得益于 Mybatis 强大的动态 SQL 功能,实现高性能动态搜索功能
-
使用 router 配置路由,实现不同用户类型导航栏的动态渲染
-
使用 axios 异步加载后端数据
-
使用 element ui 实现表单的前端校验功能
-
使用 sessionStorage 实现登录拦截
-
分别实现了基于前端和后端的数据分页功能
4、系统功能
1、admin
-
实现对教师,学生,课程的 CRUD
-
实现对教师业务以及学生业务的全方位控制
2、teacher
-
实现查询我开设的课程,以及选择我课程的学生信息
-
对学生成绩的登陆
3、student
-
实现选课退课的功能
-
实现成绩查询的功能
后端部分
1、项目运行
JDK 版本需要 1.8或者以上
2、技术栈
-
Spring boot 2.6.3
-
Mybatis
-
Maven
3、项目介绍
采用 Restful 风格开发,采用 CrossOrigin 解决跨域问题。采用注解以及 xml 文件配置 SQL 语句,实现动态 SQL 的功能,为前端提供完备的数据接口。
由于 vue 项目占用了 8080 Tomcat 默认端口,所以指定项目启动在 10086 端口, 可以使用 YAML 文件配置,使用 Maven 项目进行打包。
4、系统功能
实现前端 Ajax 请求的全部数据接口,Get 请求通过 RESTful 风格开发。
数据库设计
部分代码
@RestController
@CrossOrigin("*")
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentService studentService;
@PostMapping("/addStudent")
public boolean addStudent(@RequestBody Student student) {
System.out.println("正在保存学生对象" + student);
return studentService.save(student);
}
@PostMapping("/login")
public boolean login(@RequestBody Student student) {
System.out.println("正在验证学生登陆 " + student);
Student s = studentService.findById(student.getSid());
if (s == null || !s.getPassword().equals(student.getPassword())) {
return false;
}
else {
return true;
}
}
@PostMapping("/findBySearch")
public List<Student> findBySearch(@RequestBody Student student) {
Integer fuzzy = (student.getPassword() == null) ? 0 : 1;
return studentService.findBySearch(student.getSid(), student.getSname(), fuzzy);
}
@GetMapping("/findById/{sid}")
public Student findById(@PathVariable("sid") Integer sid) {
System.out.println("正在查询学生信息 By id " + sid);
return studentService.findById(sid);
}
@GetMapping("/findByPage/{page}/{size}")
public List<Student> findByPage(@PathVariable("page") int page, @PathVariable("size") int size) {
System.out.println("查询学生列表分页 " + page + " " + size);
return studentService.findByPage(page, size);
}
@GetMapping("/getLength")
public Integer getLength() {
return studentService.getLength();
}
@GetMapping("/deleteById/{sid}")
public boolean deleteById(@PathVariable("sid") int sid) {
System.out.println("正在删除学生 sid:" + sid);
return studentService.deleteById(sid);
}
@PostMapping("/updateStudent")
public boolean updateStudent(@RequestBody Student student) {
System.out.println("更新 " + student);
return studentService.updateById(student);
}
}
<template>
<div>
<el-form style="width: 60%" :model="ruleForm" :rules="rules" ref="ruleForm" label-width="100px" class="demo-ruleForm">
<el-form-item label="课程名" prop="cname">
<el-input v-model="ruleForm.cname"></el-input>
</el-form-item>
<el-form-item label="学分" prop="ccredit">
<el-input v-model.number="ruleForm.ccredit"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('ruleForm')">提交</el-button>
<el-button @click="resetForm('ruleForm')">重置</el-button>
<el-button @click="test">test</el-button>
</el-form-item>
</el-form>
</div>
</template>
<script>
export default {
data() {
return {
ruleForm: {
cname: null,
ccredit: null
},
rules: {
cname: [
{ required: true, message: '请输入名称', trigger: 'blur' },
],
ccredit: [
{ required: true, message: '请输入学分', trigger: 'change' },
{ type: 'number', message: '请输入数字', trigger: 'blur' },
],
}
};
},
methods: {
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
// 通过前端校验
const that = this
// console.log(this.ruleForm)
axios.post("http://localhost:10086/course/save", this.ruleForm).then(function (resp) {
console.log(resp)
if (resp.data === true) {
that.$message({
showClose: true,
message: '插入成功',
type: 'success'
});
}
else {
that.$message.error('插入失败,请检查数据库t');
}
that.$router.push("/queryCourse")
})
} else {
return false;
}
});
},
resetForm(formName) {
this.$refs[formName].resetFields();
},
test() {
console.log(this.ruleForm)
}
}
}
</script>
源码下载
(CSDN 0积分下载):springboot+vue学生选课管理系统-Java文档类资源-CSDN下载
或者加锋哥WX: java8822 (备用:java9266) 领取也行
热门推荐
我写了一套SpringBoot+SpringSecurity+Vue权限系统 实战课程,免费分享给CSDN的朋友们_java1234_小锋的博客-CSDN博客
我写了一套SpringBoot微信小程序电商全栈就业实战课程,免费分享给CSDN的朋友们_java1234_小锋的博客-CSDN博客_java 实现微信分享