Json-Lib、Org.Json、Jackson、Gson、FastJson五种方式转换json类型
只列举了最省事的方式。不涉及复制情况和速度。
测试用例,一个User类,属性name,age,location。重写toString()。
-
public
class User {
-
private String name;
-
private Integer age;
-
private String location;
-
-
public User() {
-
}
-
public User(String name) {
-
this.name = name;
-
}
-
public User(String name, Integer age) {
-
this.name = name;
-
this.age = age;
-
}
-
public User(String name, Integer age, String location) {
-
this.name = name;
-
this.age = age;
-
this.location = location;
-
}
-
-
public String getName() {
-
return name;
-
}
-
public void setName(String name) {
-
this.name = name;
-
}
-
public Integer getAge() {
-
return age;
-
}
-
public void setAge(Integer age) {
-
this.age = age;
-
}
-
public String getLocation() {
-
return location;
-
}
-
public void setLocation(String location) {
-
this.location = location;
-
}
-
-
@Override
-
public String toString() {
-
return
"User{" +
-
"name='" + name +
'\'' +
-
", age=" + age +
-
", location='" + location +
'\'' +
-
'}';
-
}
-
}
-
1、
Json-
Lib
-
maven依赖如下,需注意<classifier>jdk15</classifier>,jar包区分jdk1.
3和jdk1.
5版本
-
-
<dependency>
-
<groupId>net.sf.json-lib</groupId>
-
<artifactId>json-lib</artifactId>
-
<version>
2.4</version>
-
<classifier>jdk15</classifier>
-
</dependency>
-
-
测试demo
-
-
import net.sf.json.JSONObject;
-
-
public
class JsonLibDemo {
-
-
public
static void main(
String[] args) {
-
//创建测试object
-
User user = new
User(
"李宁",
24,
"北京");
-
System.out.
println(user);
-
-
//转成json字符串
-
JSONObject jsonObject =
JSONObject.fromObject(user);
-
String json = jsonObject.
toString();
-
System.out.
println(json);
-
-
//json字符串转成对象
-
JSONObject jsonObject1 =
JSONObject.fromObject(json);
-
User user1 = (
User)
JSONObject.toBean(jsonObject1,
User.
class);
-
System.out.
println(user1);
-
}
-
}
-
2、org.json
-
maven依赖如下
-
-
<dependency>
-
<groupId>org.json</groupId>
-
<artifactId>json</artifactId>
-
<version>
20170516</version>
-
</dependency>
-
-
测试demo
-
-
import org.json.JSONObject;
-
-
public
class
OrgJsonDemo {
-
public static void main(String[] args) {
-
//创建测试object
-
User user =
new User(
"李宁",
24,
"北京");
-
System.
out.println(user);
-
-
//转成json字符串
-
String json =
new JSONObject(user).toString();
-
System.
out.println(json);
-
-
//json字符串转成对象
-
JSONObject jsonObject =
new JSONObject(json);
-
String name = jsonObject.getString(
"name");
-
Integer age = jsonObject.getInt(
"age");
-
String location = jsonObject.getString(
"location");
-
User user1 =
new User(name,age,location);
-
System.
out.println(user1);
-
}
-
}
-
3、Jackson
-
maven依赖
-
-
<dependency>
-
<groupId>com.fasterxml.jackson.core</groupId>
-
<artifactId>jackson-databind</artifactId>
-
<version>
2.9
.0</version>
-
</dependency>
-
-
测试demo
-
-
import com.fasterxml.jackson.databind.ObjectMapper;
-
-
public
class
JacksonDemo {
-
public static void main(String[] args) {
-
//创建测试object
-
User user =
new User(
"李宁",
24,
"北京");
-
System.
out.println(user);
-
-
//转成json字符串
-
ObjectMapper mapper =
new ObjectMapper();
-
try {
-
String json = mapper.writeValueAsString(user);
-
System.
out.println(json);
-
-
-
//json字符串转成对象
-
User user1 = mapper.readValue(json,User.class);
-
System.
out.println(user1);
-
-
}
catch (java.io.IOException e) {
-
e.printStackTrace();
-
}
-
}
-
}
-
4、Gson
-
maven依赖
-
-
<dependency>
-
<groupId>com.google.code.gson</groupId>
-
<artifactId>gson</artifactId>
-
<version>
2.8
.1</version>
-
</dependency>
-
-
测试demo
-
-
import com.google.gson.Gson;
-
-
public
class
GsonDemo {
-
public static void main(String[] args) {
-
//创建测试object
-
User user =
new User(
"李宁",
24,
"北京");
-
System.
out.println(user);
-
-
//转成json字符串
-
Gson gson =
new Gson();
-
String json = gson.toJson(user);
-
System.
out.println(json);
-
-
//json字符串转成对象
-
User user1 = gson.fromJson(json,User.class);
-
System.
out.println(user1);
-
}
-
}
-
5、
FastJson
-
maven依赖
-
-
<dependency>
-
<groupId>com.alibaba</groupId>
-
<artifactId>fastjson</artifactId>
-
<version>
1.2.
37</version>
-
</dependency>
-
-
测试demo
-
-
import com.alibaba.fastjson.JSON;
-
-
public
class FastJsonDemo {
-
public
static void main(
String[] args) {
-
//创建测试object
-
User user = new
User(
"李宁",
24,
"北京");
-
System.out.
println(user);
-
-
//转成json字符串
-
String json =
JSON.toJSON(user).
toString();
-
System.out.
println(json);
-
-
//json字符串转成对象
-
User user1 =
JSON.parseObject(json,
User.
class);
-
System.out.
println(user1);
-
-
}
-
}
json-lib时间有些久远,jar包只更新到2010年
org.json用起来有些繁琐
Jackson、Gson、FastJson只需一两句话就可以搞定
原文出自:https://blog.csdn.net/n447194252/article/details/77747465
扫描二维码关注公众号,回复:
15192203 查看本文章
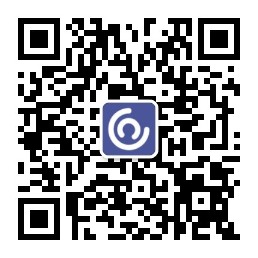