23.7. 类型安全的配置属性
使用@Value(“${property}”)注解注入配置属性有时可能比较笨重,特别是需要使用多个properties或你的数据本身有层次结构。为了控制和校验你的应用配置,Spring Boot提供一个允许强类型beans的替代方法来使用properties。
示例:
@Component
@ConfigurationProperties(prefix="connection")
public class ConnectionSettings {
private String username;
private InetAddress remoteAddress;
// ... getters and setters
}
当@EnableConfigurationProperties注解应用到你的@Configuration时,任何被@ConfigurationProperties注解的beans将自动被Environment属性配置。这种风格的配置特别适合与SpringApplication的外部YAML配置进行配合使用。
# application.yml
connection:
username: admin
remoteAddress: 192.168.1.1
# additional configuration as required
为了使用@ConfigurationProperties beans,你可以使用与其他任何bean相同的方式注入它们。
@Service
public class MyService {
@Autowired
private ConnectionSettings connection;
//...
@PostConstruct
public void openConnection() {
Server server = new Server();
this.connection.configure(server);
}
}
你可以通过在@EnableConfigurationProperties注解中直接简单的列出属性类来快捷的注册@ConfigurationProperties bean的定义。
@Configuration
@EnableConfigurationProperties(ConnectionSettings.class)
public class MyConfiguration {
}
注:使用@ConfigurationProperties能够产生可被IDEs使用的元数据文件。具体参考[Appendix B, Configuration meta-data](…/X. Appendices/B. Configuration meta-data.md)。
23.7.1. 第三方配置
正如使用@ConfigurationProperties注解一个类,你也可以在@Bean方法上使用它。当你需要绑定属性到不受你控制的第三方组件时,这种方式非常有用。
为了从Environment属性配置一个bean,将@ConfigurationProperties添加到它的bean注册过程:
@ConfigurationProperties(prefix = "foo")
@Bean
public FooComponent fooComponent() {
...
}
和上面ConnectionSettings的示例方式相同,任何以foo为前缀的属性定义都会被映射到FooComponent上。
23.7.2. 松散的绑定(Relaxed binding)
Spring Boot使用一些宽松的规则用于绑定Environment属性到@ConfigurationProperties beans,所以Environment属性名和bean属性名不需要精确匹配。常见的示例中有用的包括虚线分割(比如,context–path绑定到contextPath)和将环境属性转为大写字母(比如,PORT绑定port)。
示例:
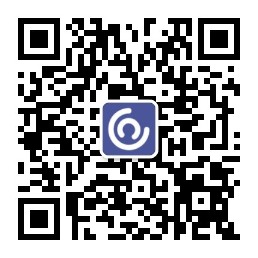
@Component
@ConfigurationProperties(prefix="person")
public class ConnectionSettings {
private String firstName;
}
下面的属性名都能用于上面的@ConfigurationProperties类:
属性 | 说明 |
---|---|
person.firstName | 标准驼峰规则 |
person.first-name | 虚线表示,推荐用于.properties和.yml文件中 |
PERSON_FIRST_NAME | 大写形式,使用系统环境变量时推荐 |
Spring会尝试强制外部的应用属性在绑定到@ConfigurationProperties beans时类型是正确的。如果需要自定义类型转换,你可以提供一个ConversionService bean(bean id为conversionService)或自定义属性编辑器(通过一个CustomEditorConfigurer bean)。
23.7.3. @ConfigurationProperties校验
Spring Boot将尝试校验外部的配置,默认使用JSR-303(如果在classpath路径中)。你可以轻松的为你的@ConfigurationProperties类添加JSR-303 javax.validation约束注解:
@Component
@ConfigurationProperties(prefix="connection")
public class ConnectionSettings {
@NotNull
private InetAddress remoteAddress;
// ... getters and setters
}
你也可以通过创建一个叫做configurationPropertiesValidator的bean来添加自定义的Spring Validator。
注:spring-boot-actuator模块包含一个暴露所有@ConfigurationProperties beans的端点。简单地将你的web浏览器指向/configprops或使用等效的JMX端点。具体参考[Production ready features](…/V. Spring Boot Actuator/40. Endpoints.md)。