前言:时不我待,忽而已春,初夏将至。然惊觉自身Java基础仍薄弱不堪,虽“雄关万道真如铁”,只得“万里关山从头越”。把基础打扎实才是根本,对于日后的工作而言也是极为重要。通过不断的学习和理解加上手动实践代码程序,才是编程学习的上上之选!
题目:使用多线程实现文件的复制。输入初始文件路径,输入目标文件夹路径,实现复制操作。
思路:
1、如果只是实现文件的复制操作,可以通过文件的reNameTo()方法实现,但是如果文件包括文件夹那就只能通过文件IO流操作实现。
2、首先,最基本的实现遍历一个文件夹所有文件并打包到一个集合中。
3、启用多线程,建立线程池,对文件进行IO流操作实现文件的复制。
一、文件的遍历
1、获取全部文件
public static List GetAllFiles(String SourceFile)//获取所有文件
{
List<File> FileList=new ArrayList<>();//文件列表
File file=new File(SourceFile);//获取源文件
if (file.exists()&&file.isDirectory()) {//如果文件存在且是文件夹形式则进行递归
FileList = LongEegodic(file, FileList);
}
return FileList;
}
2、递归
public static List LongEegodic(File file,List res)//递归获取文件、文件夹
{
File filelist[]=file.listFiles();
if (filelist==null)//文件为空直接返回
return null;
for (File file1:filelist)
{
res.add(file1);//无论是文件夹还是文件都加入列表
LongEegodic(file1,res);//递归,继续获取子文件
}
return res;
}
二、文件的IO流实现文件的复制操作
public static void Copy(File Source,File Target)//使用IO流进行文件的复制操作
{
FileInputStream Fis=null;//输入流
FileOutputStream Fos=null;//输出流
try {
Fis=new FileInputStream(Source);
Fos=new FileOutputStream(Target);
byte buf[]=new byte[1024];//每次传送的字节数量
int len=0;
while ((len=Fis.read(buf))!=-1)//如果还有字节则继续传送
{
Fos.write(buf,0,len);//写入目标文件夹
}
}
catch (Exception e)
{
e.printStackTrace();
}
finally {
try {
Fis.close();//关闭流
Fos.flush();//刷新流
Fos.close();
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
三、多线程实现文件复制
public static void main(String args[])
{
Scanner scan=new Scanner(System.in);
System.out.println("要复制的文件夹:");
String SourceFilePath=scan.next();
System.out.println("要复制到哪里去:");
String TargetFilePath=scan.next();
File SourceFile=new File(SourceFilePath);
File TargetFile=new File(TargetFilePath);
new Thread(){//多线程实现文件的复制
public void run() {
if (SourceFile.isFile()) {
System.out.println("复制单个文件");
Copy(SourceFile, TargetFile);
}
else {
List<File> FileLists=GetAllFiles(SourceFilePath);//获取全部文件
ExecutorService threadpool=Executors.newFixedThreadPool(20);//启用线程池,设定线程数量为20
for (File file:FileLists)
{
String PrimaryPath=file.getPath();//原文件路径
String NewPath=PrimaryPath.replace(SourceFile.getParent(),TargetFilePath+"/");//更改为新路径
System.out.println(PrimaryPath+ "变成了" + NewPath);
if (file.isDirectory())
{//文件夹则可以直接创建文件目录
new File(NewPath).mkdirs();
}
else {
threadpool.execute(new Runnable() {
@Override
public void run() {//重写方法
File copyfile=new File(NewPath);
copyfile.getParentFile().mkdirs();//先要有父文件夹
Copy(file,copyfile);//复制文件
}
});
}
}
}
}
}.start();
}
四、完整源码+运行结果
package FileTest;
import java.util.concurrent.Executors;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
import java.util.concurrent.ExecutorService;
public class Test6 {
public static void main(String args[])
{
Scanner scan=new Scanner(System.in);
System.out.println("要复制的文件夹:");
String SourceFilePath=scan.next();
System.out.println("要复制到哪里去:");
String TargetFilePath=scan.next();
File SourceFile=new File(SourceFilePath);
File TargetFile=new File(TargetFilePath);
new Thread(){//多线程实现文件的复制
public void run() {
if (SourceFile.isFile()) {
System.out.println("复制单个文件");
Copy(SourceFile, TargetFile);
}
else {
List<File> FileLists=GetAllFiles(SourceFilePath);//获取全部文件
ExecutorService threadpool=Executors.newFixedThreadPool(20);//启用线程池,设定线程数量为20
for (File file:FileLists)
{
String PrimaryPath=file.getPath();//原文件路径
String NewPath=PrimaryPath.replace(SourceFile.getParent(),TargetFilePath+"/");//更改为新路径
System.out.println(PrimaryPath+ "变成了" + NewPath);
if (file.isDirectory())
{//文件夹则可以直接创建文件目录
new File(NewPath).mkdirs();
}
else {
threadpool.execute(new Runnable() {
@Override
public void run() {//重写方法
File copyfile=new File(NewPath);
copyfile.getParentFile().mkdirs();//先要有父文件夹
Copy(file,copyfile);//复制文件
}
});
}
}
}
}
}.start();
}
public static void Copy(File Source,File Target)//使用IO流进行文件的复制操作
{
FileInputStream Fis=null;//输入流
FileOutputStream Fos=null;//输出流
try {
Fis=new FileInputStream(Source);
Fos=new FileOutputStream(Target);
byte buf[]=new byte[1024];//每次传送的字节数量
int len=0;
while ((len=Fis.read(buf))!=-1)//如果还有字节则继续传送
{
Fos.write(buf,0,len);//写入目标文件夹
}
}
catch (Exception e)
{
e.printStackTrace();
}
finally {
try {
Fis.close();//关闭流
Fos.flush();//刷新流
Fos.close();
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
public static List GetAllFiles(String SourceFile)//获取所有文件
{
List<File> FileList=new ArrayList<>();//文件列表
File file=new File(SourceFile);//获取源文件
if (file.exists()&&file.isDirectory()) {//如果文件存在且是文件夹形式则进行递归
FileList = LongEegodic(file, FileList);
}
return FileList;
}
public static List LongEegodic(File file,List res)//递归获取文件、文件夹
{
File filelist[]=file.listFiles();
if (filelist==null)
return null;
for (File file1:filelist)
{
res.add(file1);
LongEegodic(file1,res);
}
return res;
}
}
结果:
扫描二维码关注公众号,回复:
15136522 查看本文章
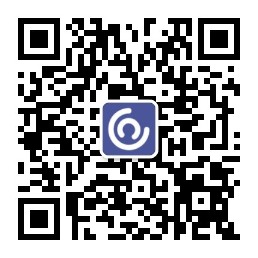
发文不易,恳请大佬们高抬贵手!
点赞:随手点赞是种美德,是大佬们对于本人创作的认可!
评论:往来无白丁,是你我交流的的开始!
收藏:愿君多采撷,是大佬们对在下的赞赏!